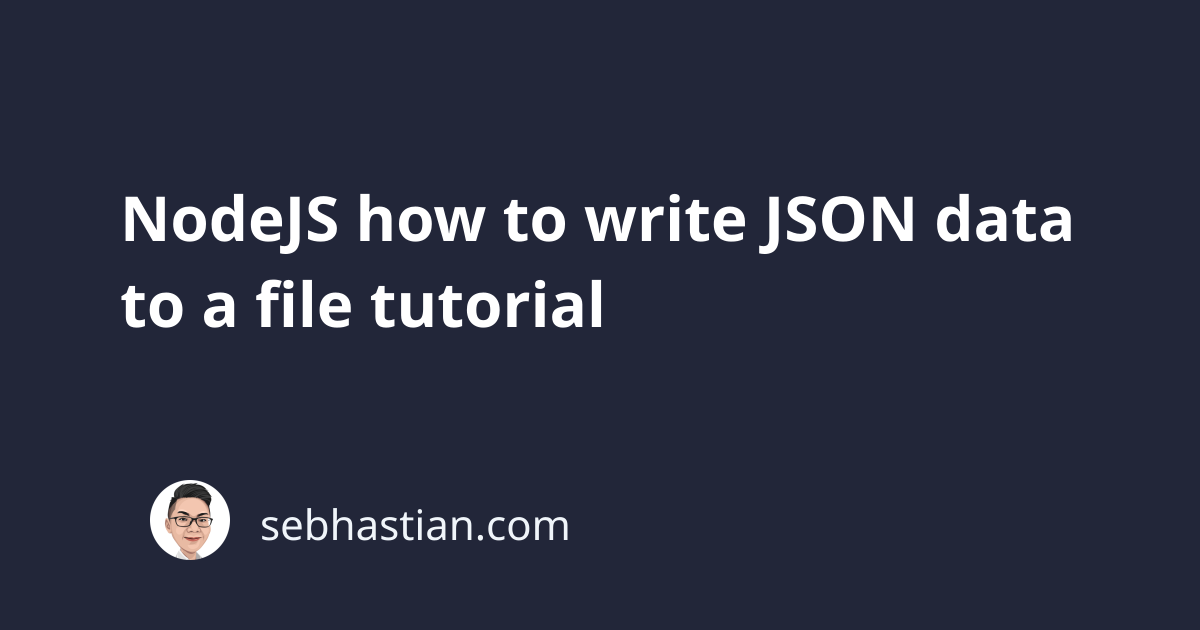
You can write a JSON string to a .json
file using NodeJS by using the fs
module.
The fs
module is a built-in NodeJS module that allows you to work with the file system on your computer. The module has several methods that enable you to write data to a file and save it on your computer.
To use the module, you just need to require()
it as follows:
const fs = require("fs");
With the module available for your JavaScript code, you can use the writeFile()
or the writeFileSync()
method to write and save your JSON string to a .json
file.
First, you need to make sure you have a valid JSON string. If you have an object data type, you can use JSON.stringify()
method to convert the object into a valid JSON string.
const user = {
name: "Nathan",
age: 28,
role: "Web developer",
};
const userData = JSON.stringify(user);
console.log(userData);
// '{"name":"Nathan","age":28,"role":"Web developer"}'
Once you have a valid JSON string, you can call the writeFile()
method to write the string to a file.
You need to pass the following parameters to the method:
- The file name
string
for the output file as the first parameter - The content
string
to write into the file as the second parameter - The callback
function
to execute when the write process is finished
If there’s an error during the write process, the method will pass the Error
object err
to the callback function. You can simply log the error using console.error()
method when the err
object is defined.
Here’s an example of the writeFile()
method in action:
const fs = require("fs");
const user = {
name: "Nathan",
age: 28,
role: "Web developer",
};
const userData = JSON.stringify(user);
fs.writeFile("output.json", userData, function (err) {
if (err) {
console.error(err);
} else {
console.log("output.json has been saved with the user data");
}
});
The code above will write create a new file named output.json
with the JSON string userData
as its content.
You can also write the file synchronously by using the writeFileSync()
method. You only need to pass the file name and the string you want to write to the file as follows:
const fs = require("fs");
const user = {
name: "Nathan",
age: 28,
role: "Web developer",
};
const userData = JSON.stringify(user);
fs.writeFileSync("output.json", userData);
console.log("output.json has been saved with the user data");
Be aware that an error with the writeFileSync()
method will cause your JavaScript program to crash.
To prevent the method from crashing the program, you need to wrap it using a try..catch
block as follows:
const fs = require("fs");
const user = {
name: "Nathan",
age: 28,
role: "Web developer",
};
const userData = JSON.stringify(user);
try {
fs.writeFileSync("output.json", userData);
console.log("output.json has been saved with the user data");
} catch (err) {
console.error(err);
}
With that, the error during the write process won’t crash your JavaScript program.
And that’s how you can write a JSON string to a .json
file using NodeJS 😉