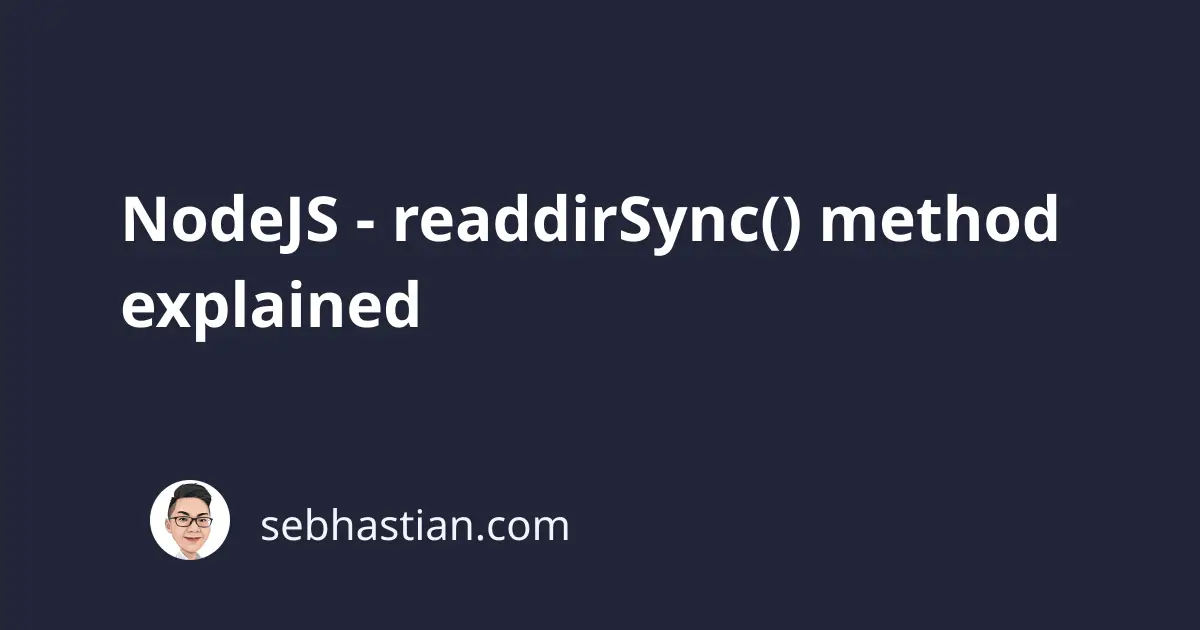
The readdirSync()
from the fs
module allows you to read a folder’s content using NodeJS. The method will list all filenames inside the folder as an array and return them to you for further operations.
The method `read accepts the following 2 parameters:
path
to the directory - string (required)options
to affect the method return value - object (optional)
The options
parameter allows you to pass additional parameters as follows:
encoding
- specifies the encoding used for the filenames returned byreaddirSync()
withFileTypes
- The returned array will containDirent
objects instead of just a string
The following code example will read the content of the current directory or folder and log the file names to the console:
const fs = require("fs");
const files = fs.readdirSync(__dirname);
console.log(files); // Log the content of the current folder
When you need to retrieve the content of a specific folder, you can pass the path
to the folder as a string. The code below will look into the assets
folder, which is right inside the current folder:
const fs = require("fs");
const path = "./assets";
const files = fs.readdirSync(path);
console.log(files); // Log the content of assets folder
When you set the withFileTypes
option to true
, the method will return an array of Dirent
objects as follows:
const fs = require("fs");
const files = fs.readdirSync(__dirname, { withFileTypes: true });
console.log(files);
[
Dirent { name: '.DS_Store', [Symbol(type)]: 1 },
Dirent { name: 'assets', [Symbol(type)]: 2 },
Dirent { name: 'index.html', [Symbol(type)]: 1 },
Dirent { name: 'index.js', [Symbol(type)]: 1 },
Dirent { name: 'read.js', [Symbol(type)]: 1 },
Dirent { name: 'vue.png', [Symbol(type)]: 1 }
]
Unfortunately, the Dirent
object doesn’t have the file types property where you can check for a specific type. When you need to return only specific file types, you can use a combination of array filter()
method and extname()
method from the path
module.
First, read the folder without passing any option. Then, use the filter()
method on the returned array and return
only files with the specified extension.
For example, the code below will return only files with .js
extension in my current folder:
const fs = require("fs");
const path = require("path");
const files = fs.readdirSync(__dirname);
const jsFiles = files.filter(function (file) {
return path.extname(file) === ".js";
});
console.log("The following files are using .js extension: ");
console.log(jsFiles); // [ 'index.js', 'read.js' ]
By using fs.readdirSync()
method, you can easily read the content of any folder located in your filesystem.