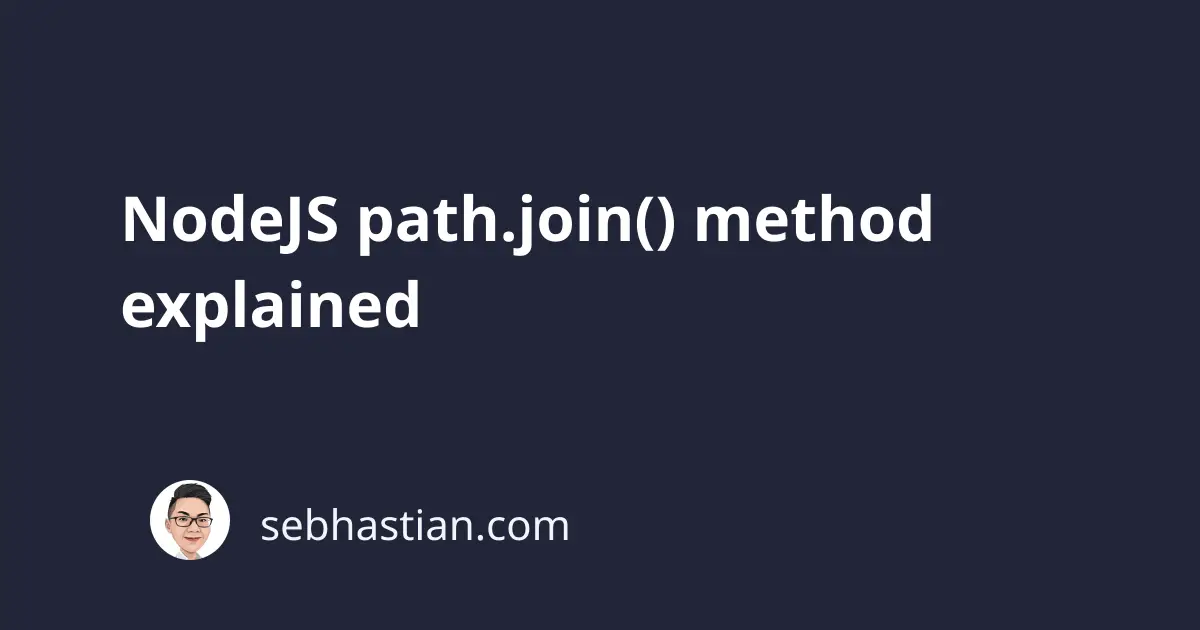
Every computer operating system has a file path that you can navigate using the command line.
The join()
method from the path
module in NodeJS provides you with a way to concatenate multiple path segments together to form a new path.
path.join(path, ...)
The join()
method accepts as many path
parameters as you need. The paths are specified as string
types and separated by a comma.
The method will normalize the path segments and return a joined string
path.
Here’s an example of the join()
method in action:
const path = require('path');
const myPath = path.join("users", "admin", "index.js");
console.log(myPath); // users/admin/index.js
When you want the path to go up instead of down, you can add the ../
before the name of the folder or file. Here’s an example:
const path = require('path');
const myPath = path.join("users", "admin", "../index.js");
console.log(myPath); // users/index.js
const anotherPath = path.join("home","users", "../admin", "index.js");
console.log(anotherPath); // home/admin/index.js
And that’s how the path.join()
method works 😉