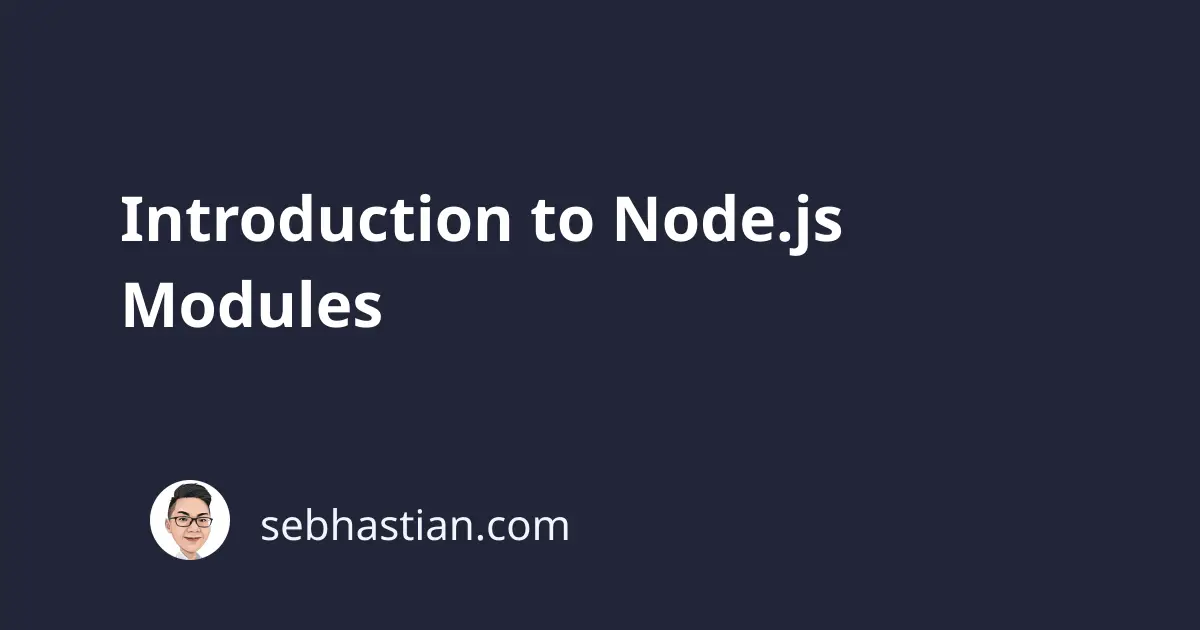
Node.js used the CommonJS module format as a blueprint to implement its own Modules feature. Modules are one of the most used features for building web applications in JavaScript, because it allows you to:
- Share your code with others
- Use code shared by others in your project
You might wonder, “Why Node uses CommonJS specification instead of ES6 Modules (ESM) specification?”
This is because Node.js has been under development since 2009, while ESM was released in 2015.
At the time of this writing, Node.js development team is trying to stabilize the support of ES6 Modules syntax so that you and I can use ESM both in the browser and server.
But even so, the majority of server-side JavaScript code would probably be written using CommonJS format. Node.js team has decided to support CommonJS format for the foreseeable future anyway, so you still need to learn it to collaborate with others.
Here’s the simplest module export example:
// sayHello.js
function sayHello(){
console.log("Hello World!")
}
module.exports = sayHello
You simply need to use module.exports
syntax in order to export a variable for other files to import. Next, you can import your exported variable in other files by using the require
syntax like the following:
const hello = require('./sayHello')
You need to assign the result of require
function call into a variable. The name of the variable doesn’t have to match the name of your module that you exported. You can name the variable any valid JavaScript variable name. Also, you don’t need to write the .js
extension behind your file name.
Because hello
is now an alias for sayHello
function, you can print “Hello World!” by calling on it:
const hello = require('./sayHello')
hello()
Import from a directory
Alternatively, you can also import from a directory name, and Node will automatically look for index.js
file inside that directory:
// will look for the ./lib/index.js file
const hello = require('./lib')
If the directory doesn’t have an index.js
file, Node will throw an error.
Alternative export syntax
There are two export syntaxes that you can use in Node.js: module.exports
and just exports
syntax. When you use the exports
syntax, you need to assign values that you want to export into the exports
object property instead of directly assigning to exports
.
The following exports will return an empty object {}
when imported:
exports = "89"
While the following will return { userId: '89' }
:
exports.userId = "89"
If there are both module.exports
and exports
syntax in one file, module.exports
will be prioritized and exports
will be ignored.
The following example will return ‘Joan’:
exports.userId = "89"
module.exports = 'Joan'
Multiple exports
CommonJS module will always export a single object, but you can include multiple variables as the object properties. Here’s how you do it with module.exports
syntax:
function sayHello(){
console.log("Hello World!")
}
const username = 'Joan'
module.exports = {username, sayHello}
Or when using object dot notation:
module.exports.username = username
module.exports.sayHello = sayHello
But if you prefer dot notation, you can use exports
syntax for a shorter code:
exports.username = username
exports.sayHello = sayHello
You can then import the assigned properties with a destructuring assignment:
const { username, sayHello } = require('./sayHello')
Or with a regular assignment and dot notation:
const exports = require('./sayHello')
exports.sayHello()
console.log(exports.username)
And that’s how you import and export variables in server-side JavaScript code.