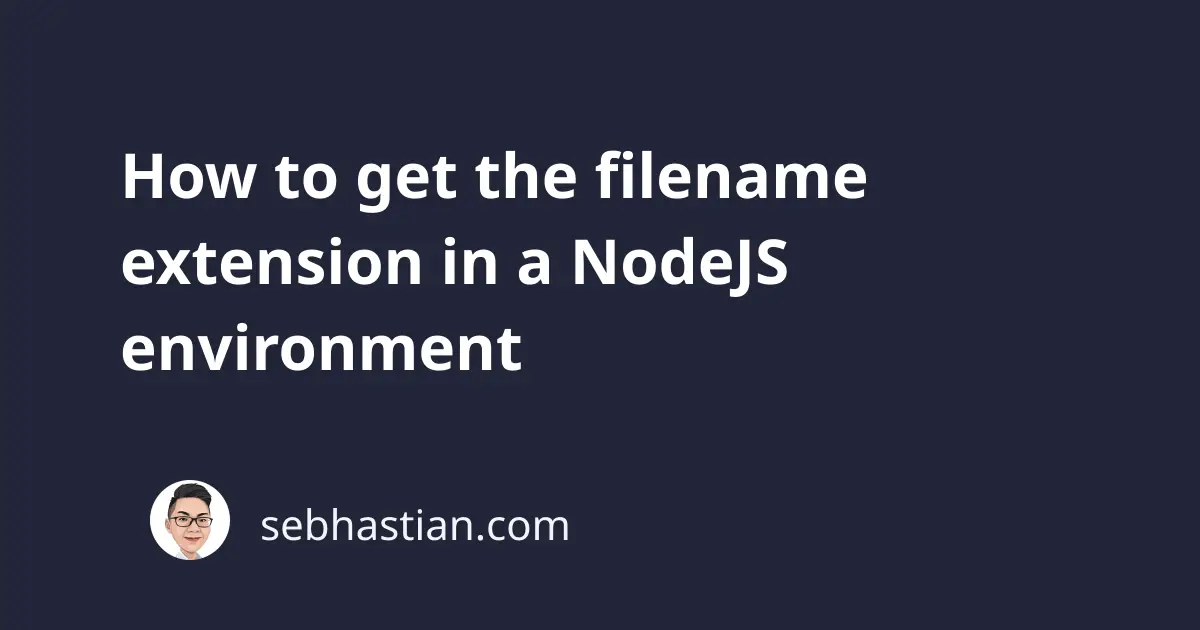
NodeJS can help you to extract the extension type of file names in your filesystem.
The built-in extname()
method from the path
module returns the extension of the string
path from the last occurence of the period .
character to the end of the string.
When no period character is found, the method will return an empty string:
const path = require("path");
path.extname("index.md"); // .md
path.extname("index."); // "."
path.extname("index"); // ""
Using the method, you can easily find the extension of files in a certain directory by combining the method with the fs.readdirSync()
method.
The readdirSync()
method returns an array of file names, and you can log the extension of each file using the path.extname()
method.
Here’s an example of printing all available file names and their extensions in the current directory:
const fs = require("fs");
const path = require("path");
const files = fs.readdirSync(__dirname);
files.forEach(function (file) {
console.log(`File name: ${file} | Extension type: ${path.extname(file)}`);
});
The path
module is only available under NodeJS runtime, so you need to find another way to extract file extension if you’re running JavaScript in the browser.