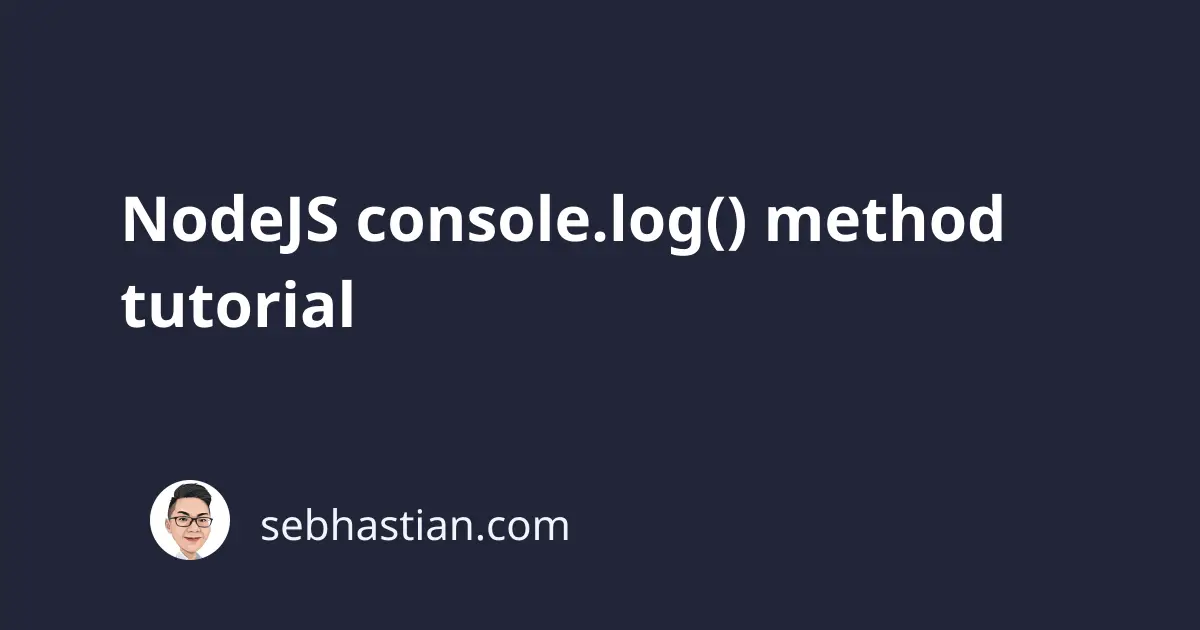
The NodeJS console.log()
method is a built-in method of the console
module that provides you with an easy way to debug and print important information related to your application’s development.
The console
module is always available by default, so you can just call the console.log()
method anytime you want to print a certain output.
You need to pass the message you want to log into the console as the first argument of the method:
console.log(message);
For example, the following code will print "Hello, World!"
to the console for you:
console.log("Hello, World!");
Save the code under the name of index.js
and run the file using node
command as follows:
node index.js
The output of your command will be as follows:
The console.log()
method also allows you to print variable values to the console in three ways.
First, you can print a variable value by using string concatenation or string interpolation as follows:
let name = "Nathan";
// string concatenation
console.log("Hello, " + name); // Hello, Nathan
// string interpolation
console.log(`Hello, ${name}`); // Hello, Nathan
Second, you can simply pass the variable values you want to be printed as arguments to the method as follows:
let name = "Nathan";
let age = 28;
console.log("Hello, my name is", name, "and I'm", age, "years old");
// Hello, my name is Nathan and I'm 28 years old
Notice how five arguments are passed to the console.log()
method above. This is because the method will use format extra arguments passed into the method as space-separated values in one string.
Finally, you can also use formatted placeholders to print variable values. Notice the use of formatter %s
and %d
inside the console.log()
method below:
let name = "Nathan";
let age = 28;
console.log("Hello, my name is %s and I'm %d years old", name, age);
The console.log()
method allows you to use formatters to add variables into your message. The available formatters are as follows:
%s
format a variable as a string%i
or%d
format a variable as a number (integer)%o
format a variable as an object
Formatters will process the variables you passed into the method from left to right.
In case of the example above, the first formatter found is %s
and it will be replaced by the name
variable. The second formatter %d
will be replaced by the variable age
.
Keep in mind that console.log()
method is meant to help you in debugging and developing JavaScript applications. Make sure that you remove any trace of console.log()
in your application before you deploy it for the production environment.