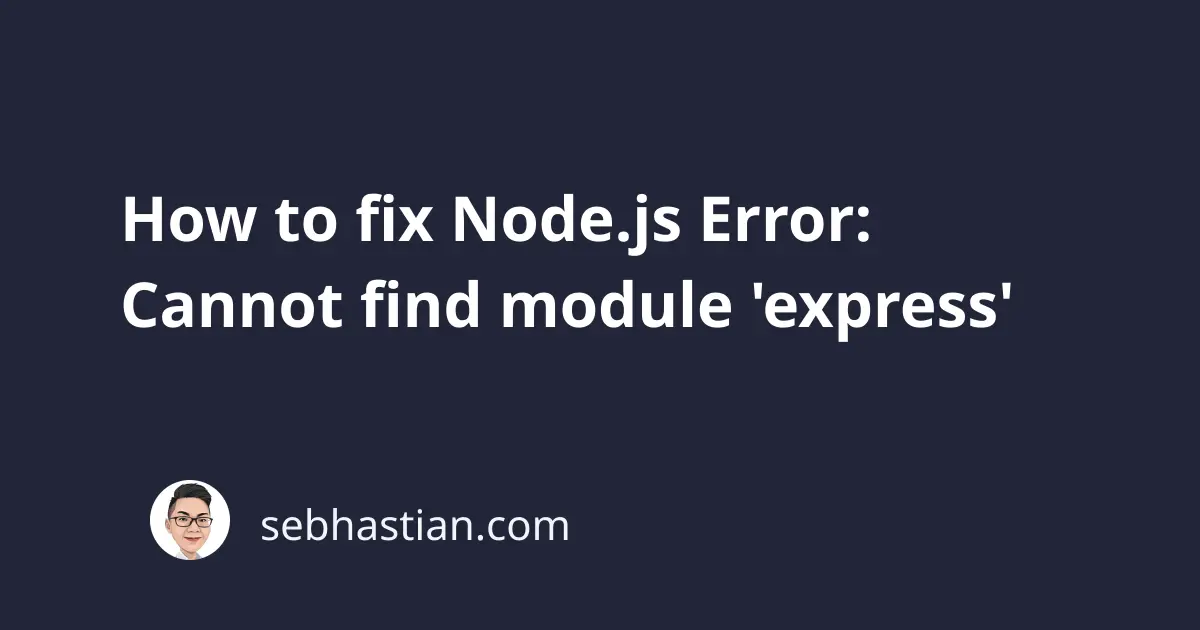
One common error that you might encounter when running a Node.js application is:
Error: Cannot find module 'express'
This error occurs when you run a Node.js application that requires the Express module, but the module can’t be found in your local node_modules
folder.
Let me show you an example that causes this error and how you can fix it.
How to reproduce this error
Suppose you’re developing a web application using Node.js as your JavaScript runner.
You write the code to import the express
module and create a server at a specific port as shown below:
const express = require("express");
const app = express();
const port = 3000;
app.listen(3000, () => {
console.log(`Example app listening on port ${port}`);
});
app.get("/", (req, res) => {
res.send("Hello World!");
});
Next, you run the code using the node index
or node app.js
command from your terminal.
But you get the following error:
Error: Cannot find module 'express'
This error occurs because Node.js can’t find the express
module inside your project folder.
Node.js needs the package to be installed using npm or Yarn so that it can be used.
How to fix this error
To resolve this error, you need to install the express
module using npm or Yarn.
Here’s the command you need to run:
# If you use npm:
npm install express
# If you use Yarn:
yarn add express
Note that you need to install the package locally in your project folder.
If you previously installed Express using the global -g
option as follows:
npm install -g express
Then the error won’t be resolved because Node.js doesn’t search the global package when running your code.
You need to make sure that you’re installing express locally by searching for the express
folder inside the node_modules
folder as shown below:
project
├── app.js
├── node_modules
│ └── express
├── package-lock.json
└── package.json
...
You might also see other related packages that are required by Express as dependencies, but the main point is that Node.js will look for the express
folder when you run the const express = require("express");
line.
If you have the package installed, then the error should be resolved.
Conclusion
The Node.js Error: Cannot find module ’express’ occurs when you try to import the express
module without having it installed on your local project folder.
To resolve this error, run the command npm install express
or yarn add express
from the project root folder.
Installing the package globally won’t resolve this error. You need to install it right on the project.
I hope this tutorial helps. Happy coding! 🙌