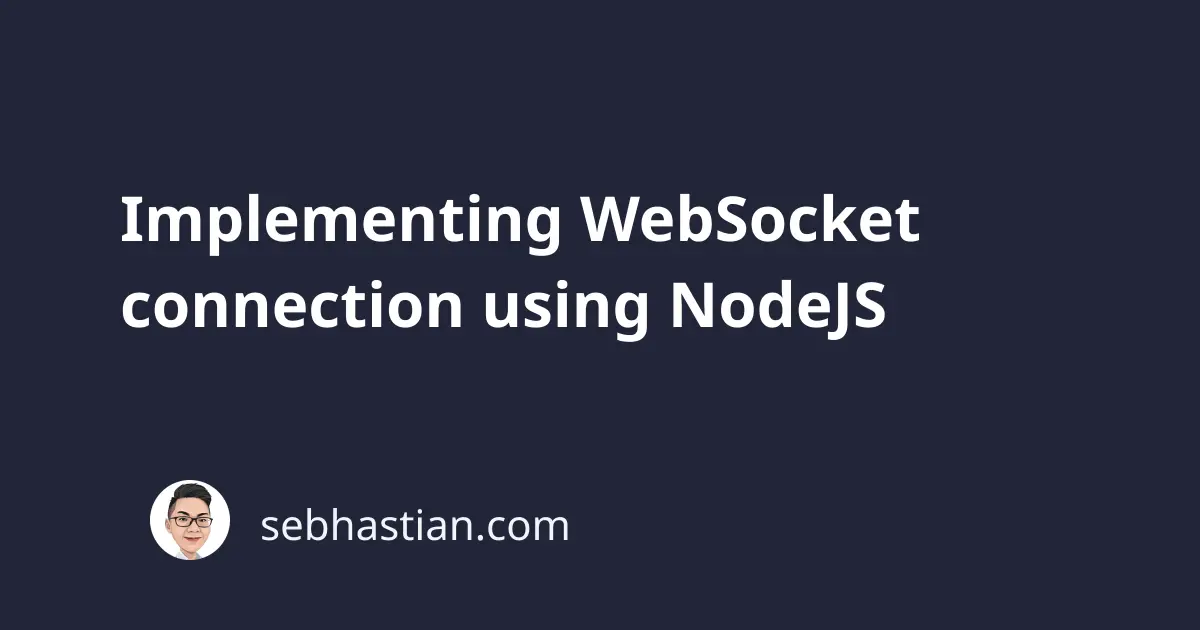
WebSocket is a computer communications protocol that offers you a two-way communication channel between a client and a server.
It’s quite different from the HTTP protocol which is used as the standard communication protocol in the browser.
With a WebSocket connection, the server can send messages to the client without waiting for a client request. In HTTP connection, a request must be initiated by the client.
Once a WebSocket connection has been established between the client and the server, the WebSocket channel will be kept open in the background so that the communication will have lower overhead and latency when compared to HTTP connection.
Just like how you can create an HTTP server to receive HTTP requests, you can also create a WebSocket server to establish WebSocket connections using NodeJS.
This tutorial will help learn how to create your own WebSocket server with NodeJS
The complete implementation code can be found in this node-websocket-example repo.
Creating a WebSocket server in NodeJS
To create a WebSocket server in NodeJS, you can use the ws module, which is the most widely used WebSocket implementation for NodeJS.
First, you need to install the ws
module to your project:
npm install ws
# or
yarn add ws
Once you have the module installed, you can immediately create an index.js
file where you create a new WebSocket server.
First, import the ws
module and open a WebSocket server connection on a specific port. I’m going to use port 8080 in the example below:
const WebSocket = require("ws");
const wss = new WebSocket.Server({ port: 8080 });
Next, write the code to let your Websocket server send a message to the client when a connection has been established.
You need to listen to WebSocket connection
event and send()
a message when a connection has been made:
wss.on("connection", (ws) => {
// A connection has been made
// send back a message
ws.send("Hi client! You are now connected to me!");
});
Finally, you also need to listen to the message
event, which will be triggered whenever the client send a message to the server as follows:
wss.on("connection", (ws) => {
// A connection has been made
// send back a message
ws.send("Hi client! You are now connected to me!");
// listen to the message event
// then log the received message
ws.on("message", (message) => {
console.log("New message from client: %s", message);
});
});
And that’s all you need to create a WebSocket server using NodeJS. Let’s create a WebSocket client to connect to your ws
server from the browser.
Connecting to a WebSocket from the browser.
Most popular web browsers like Chrome, Safari and Firefox already implements a native JavaScript WebSocket API that you can use to connect to a WebSocket server.
Here’s the list of WebSocket API support according to caniuse.com:
Let’s try connecting to the WebSocket server with a simple HTML file.
First, create an index.html
file with the following <body>
content:
<body>
<h1>WebSocket Client Implementation</h1>
<button id="wsConnect">Connect to WebSocket</button>
</body>
Next, add an event listener to the <button>
element that will listen for the click
event.
The event listener will execute a function that opens a connection to your WebSocket server:
const button = document.querySelector("#wsConnect");
button.addEventListener("click", () => {
const url = "ws://localhost:8080";
const wsClient = new WebSocket(url);
});
The constructor new WebSocket()
will return a new WebSocket object from which you can listen for WebSocket related events.
For example, you can listen to WebSocket.onopen
property, which is an event handler that gets triggered when the connection is ready to send and receive data:
const button = document.querySelector("#wsConnect");
button.addEventListener("click", () => {
const url = "ws://localhost:8080";
const wsClient = new WebSocket(url);
wsClient.onopen = () => {
console.log(`Connection to ${url} has been established`);
};
});
Next, you can also listen to the onmessage
event handler, which gets triggered when the client receives a message:
wsClient.onmessage = (e) => {
console.log(e.data);
};
Finally, you can add a listener for the onerror
property too. It’s called when the WebSocket connection encounters an error:
wsClient.onerror = (err) => {
console.log(`WebSocket error: ${err}`);
};
Here’s the full HTML page code for testing WebSocket connections:
<body>
<h1>WebSocket Client Implementation</h1>
<button id="wsConnect">Connect to WebSocket</button>
<script>
const button = document.querySelector("#wsConnect");
button.addEventListener("click", () => {
const url = "ws://localhost:8080";
const wsClient = new WebSocket(url);
wsClient.onopen = () => {
console.log(`Connection to ${url} has been established`);
};
// receive message from the server
wsClient.onmessage = (e) => {
console.log(e.data);
};
// Log WebSocket error events
wsClient.onerror = (err) => {
console.log(`WebSocket error: ${err}`);
};
});
</script>
</body>
For the full list of WebSocket object properties, you can see the MDN WebSocket documentation.
It’s time to test your WebSocket implementation. Open the index.html
file from your browser and click on the button.
You will see the logs from the browser console as follows:
You’ve successfully established a WebSocket connection between the NodeJS server and the browser! Try sending a message to the server from the onopen
handler function:
wsClient.onopen = () => {
console.log(`Connection to ${url} has been established`);
wsClient.send("Hello World!");
};
You’ll see the text logged from the NodeJS console:
You’ve just learned how to implement WebSocket connections using NodeJS and the browser. Great job! 😉
For exercise, try adding a form with a text <input>
element and a submit button. You can send the message to the server using WebSocket.send()
method each time the submit button is clicked.