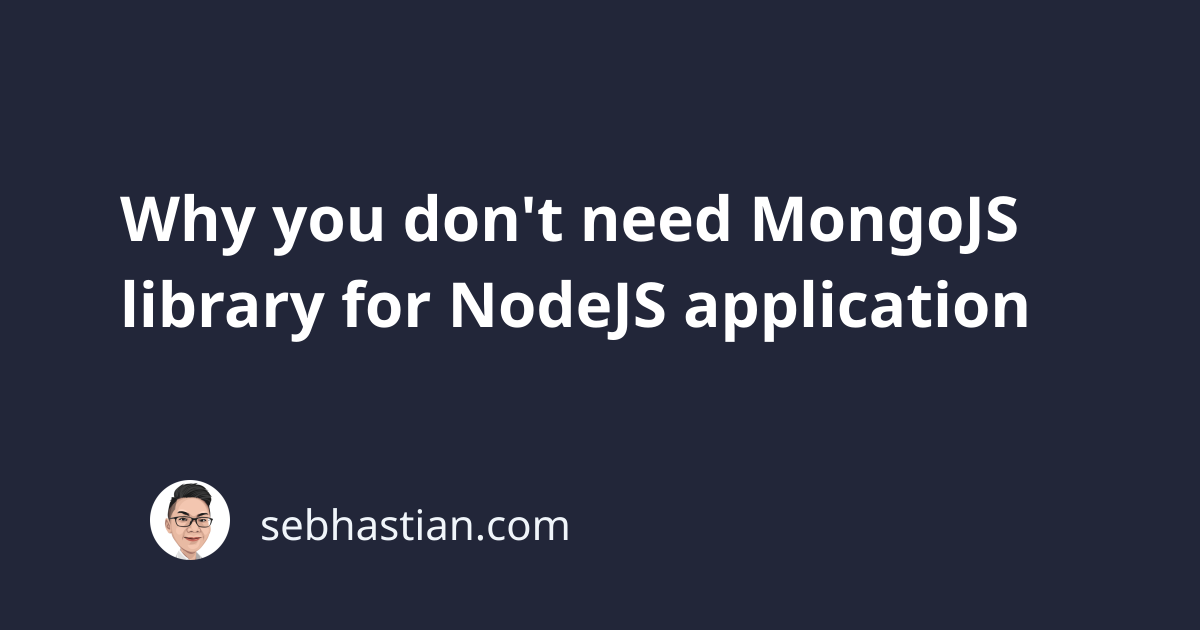
The mongojs
npm package is a NodeJS module that implements the MongoDB native driver in NodeJS. The purpose of the package is to help JavaScript developers to connect their NodeJS instance to their MongoDB instance.
But with the release of the official MongoDB driver for NodeJS, the mongojs
package is now obsolete and it’s recommended for you to use mongodb
package instead.
The mongodb
NodeJS driver comes with asynchronous Javascript API functions that you can use to connect and manipulate your MongoDB data.
You need to install the mongodb
package to your project first:
npm install mongodb
# or
yarn add mongodb
Once installed, you need to import the MongoClient
class from the library as follows:
const { MongoClient } = require("mongodb");
Then, you can connect to your MongoDB database by creating a new instance of the MongoClient
class and passing the URI as its argument:
const uri = "mongodb+srv://<Your MongoDB atlas cluster scheme>";
const client = new MongoClient(uri, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
If you’re installing MongoDB locally, then you can connect by passing the MongoDB localhost
URI scheme as shown below:
const client = new MongoClient("mongodb://localhost:27017", {
useNewUrlParser: true,
useUnifiedTopology: true,
});
Then, connect to the database using the client.connect()
method as shown below:
client.connect(async (err) => {
if (err) {
console.log(err);
}
const collection = client.db("test").collection("animals");
// perform actions on the collection object
});
Almost all MongoDB API methods will return a Promise
object when you don’t pass a callback
function to the methods.
This means you can use either the callback
pattern, the promise chain pattern, or the async/await
pattern when manipulating your collection’s data.
See also: Wait for JavaScript functions to finish using callbacks and promises
Here’s how to use the connect()
method using the async/await
pattern. Don’t forget to wrap the code below inside an async
function:
try {
await client.connect();
console.log(`connect OK`);
const collection = client.db("test").collection("animals");
// perform actions on the collection object
} catch (error) {
console.log(`connect error: ${error}`);
}
Once connected, you can choose the database you want to connect with by calling the client.db()
method and specify the database string
as its argument:
const db = client.db("test");
You can also choose the collection you want to manipulate by calling the db.collection()
method and specify the collection string
as its argument:
const db = client.db("test");
const collection = db.collection("animals");
The db()
and collection()
method calls can be chained for brevity.
Once a connection has been established to the MongoDB instance, you can manipulate the database using methods provided by MongoDB library.
Inserting documents to a MongoDB collection
To insert a document to your MongoDB collection, you can use either insertOne()
or insertMany()
method.
The insertOne()
method is used to insert a single document into your collection object. Here’s an example of the method in action:
await collection.insertOne({
name: "Barnes",
species: "Horse",
age: 5,
});
Or when using callback pattern:
collection.insertOne(
{
name: "Barnes",
species: "Horse",
age: 5,
},
(err, result) => {
// handle error/ result...
}
);
When using insertMany()
method, you need to wrap all your object
documents in a single array
:
await collection.insertOne([
{
name: "Barnes",
species: "Horse",
age: 5,
},
{
name: "Jack"
species: "Dog",
age: 4,
}
]);
Next, let’s look at finding documents in your collection.
Finding documents in a MongoDB collection
To find documents inside your MongoDB collection, the library provides you with the findOne()
and find()
method.
Here’s how to use them:
const doc = await collection.findOne({ name: "Barnes" });
console.log('The document with { name: "Barnes" } =>', doc);
// or
const filteredDocs = await collection.find({ species: "Horse" }).toArray();
console.log("Found documents =>", filteredDocs);
To retrieve all documents available in your collection, you can pass an empty object {}
as the parameter to your find()
method call:
const docs = await collection.find({}).toArray();
console.log("All documents =>", docs);
To query by comparison, you need to use the built-in Comparison Query Operators provided by MongoDB.
For example, the following find()
call only retrieve documents with age
value greater than 2
:
const filteredDocs = await collection.find({ age: { $gt: 2 } }).toArray();
console.log("Found documents =>", filteredDocs);
Next, let’s look at how you can update the documents.
Updating documents in a MongoDB collection
You can update documents inside your collection by using the updateOne()
or updateMany()
method.
The update query starts with the $set
operator as shown below:
{ $set: { <field1>: <value1>, <field2>: <value2>, ... } }
For example, the code below updates a document with name:"Barnes"
to have age: 10
:
const updatedDoc = await collection.updateOne(
{ name: "Barnes" },
{ $set: { age: 10 } }
);
console.log("Updated document =>", updatedDoc);
When there is more than one document that matches the query, the updateOne()
method only updates the first document returned by the query (commonly the first document inserted into the collection)
To update all documents that match the query, you need to use the updateMany()
method:
const updatedDocs = await collection.updateMany(
{ species: "Dog" },
{ $set: { age: 3 } }
);
console.log("Set all Dog age to 3 =>", updatedDocs);
Now let’s see how you can delete documents from the collection.
Deleting documents in a MongoDB collection
The deleteOne()
and deleteMany()
methods are used to remove document(s) from your collection.
You need to pass the query for the delete methods as shown below:
const deletedDoc = await collection.deleteOne({ name: "Barnes" });
console.log('Deleted document with {name: "Barnes"} =>', deletedDoc);
// or
const deletedDocs = await collection.deleteMany({ species: "Horse" })
console.log("Deleted horse documents =>", deletedDocs);
Closing your MongoDB connection
Finally, when you’re done with manipulating the database entries, you need to close the Database connection properly to free your computing resource and prevent connection leak
Just call the client.close()
method as shown below:
client.close();
And that will be all for this tutorial.
For more information, visit the official MongoDB NodeJS driver documentation and select the API documentation for your current version from the right side menu:
Thank you for reading 😉