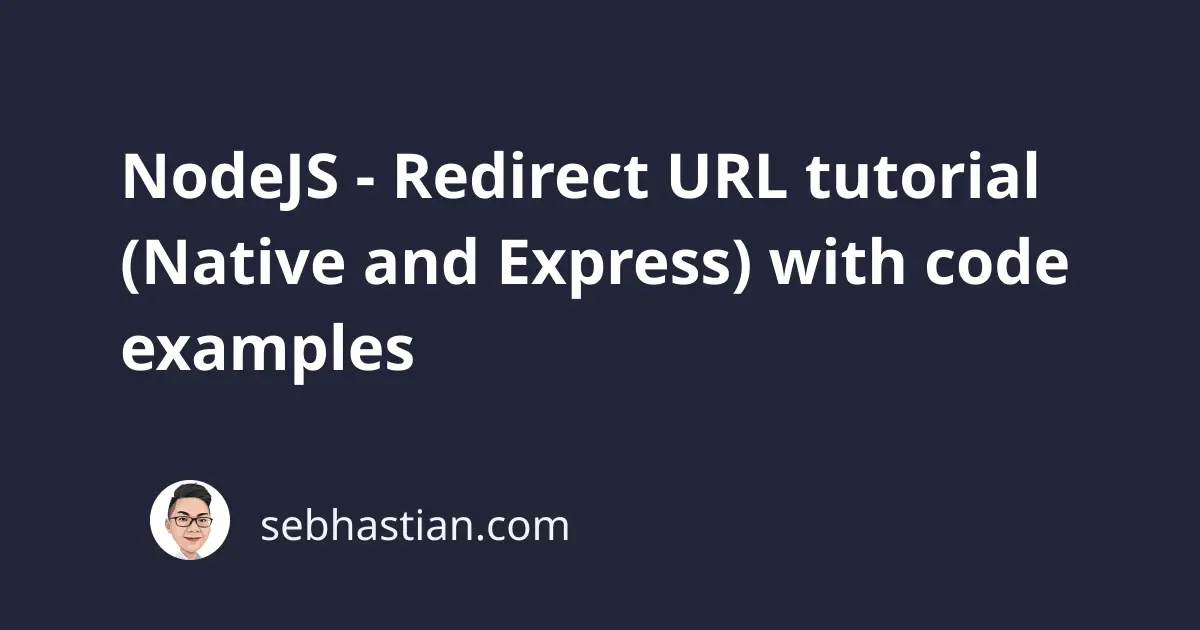
When you create web applications using NodeJS, you will eventually need to perform a URL redirect for two common reasons:
- The previous URL has been moved to another URL (301 or 302 redirect)
- The request is unknown (404)
This tutorial will help you do both redirects using native NodeJS http
module and Express framework. The code example for this tutorial is available on GitHub.
Let’s start with how to perform a redirect using http
module.
NodeJS redirect using http module
The http
module is a built-in NodeJS module that allows you to create an HTTP server to receive HTTP requests and write responses. To use the module, you can require()
it on your JavaScript file:
const http = require("http");
Then, create a server using the createServer()
method that receives a request (req
) and response (res
) listener:
const http = require("http");
const server = http.createServer(function (req, res) {});
server.listen(3000, function () {
console.log("server started at port 3000");
});
And run the created server
using server.listen()
method, passing the HTTP port to listen for requests:
server.listen(3000, function () {
console.log("server started at port 3000");
});
Now you need to write the responses your server will understand inside the createServer()
method. First, grab the request url which is stored in req.url
:
const server = http.createServer(function (req, res) {
const url = req.url;
});
Then, you need to write an if
block to see if the request URL matches a certain pattern. For example, here’s how to respond to the index route /
requested by the client:
const server = http.createServer(function (req, res) {
const url = req.url;
if (url === "/") {
// do a 200 response
res.writeHead(200, { "Content-Type": "text/html" });
res.write("<h1>Hello World!<h1>");
res.end();
}
});
The res.writeHead()
method allows you to write the HTTP response header, while res.write()
will put content in the response body. The res.end()
method tells your server to end the response process.
To do a 301 or 302 redirect, you just need to use the same methods for the URL that has been moved. For example, let’s do a 302 redirect when clients request the /google
route.
Instead of writing a response using res.write()
, you need to write the HTTP response header with the location
property as follows:
const server = http.createServer(function (req, res) {
const url = req.url;
if (url === "/") {
// do a 200 response
res.writeHead(200, { "Content-Type": "text/html" });
res.write("<h1>Hello World!<h1>");
res.end();
} else if (url === "/google") {
// do a 302 redirect
res.writeHead(302, {
location: "https://google.com",
});
res.end();
}
});
With that, you will be redirected to google.com when you hit the localhost:3000/google
URL.
Finally, to do a 404 redirect, you can create an else
block for all URLs that may be requested by your clients and use writeHead()
to send a 404 response as shown below:
const server = http.createServer(function (req, res) {
const url = req.url;
if (url === "/") {
// do a 200 response
res.writeHead(200, { "Content-Type": "text/html" });
res.write("<h1>Hello World!<h1>");
res.end();
} else if (url === "/google") {
// do a 302 redirect
res.writeHead(302, {
location: "https://google.com",
});
res.end();
} else {
// do a 404 redirect
res.writeHead(404);
res.write("<h1>Sorry nothing found!<h1>");
res.end();
}
});
That’s how you can create redirects using native NodeJS module. Let’s learn how you can do the same using Express framework next.
NodeJS redirect using Express framework
To perform a redirect using Express framework, you simply need to send a response using the redirect()
method provided by the framework.
For example, here’s how you perform a 302
redirect with Express framework:
const express = require("express");
const app = express();
const port = 3000;
app.get("/google", (req, res) => {
res.redirect(301, "https://google.com");
});
app.listen(port, () => {
console.log(`Express app listening at http://localhost:${port}`);
});
To perform a 404 response, you need to create an Express middleware. This middleware will be responsible for sending the 404
response. You need to make sure that this middleware is written just above app.listen()
method so that it will be run only after all other routes have been checked.
Here’s an example:
const express = require("express");
const app = express();
const port = 3000;
app.get("/", (req, res) => {
res.status(200).type("html").send("<h1>Hello World!<h1>");
});
app.get("/google", (req, res) => {
res.redirect(301, "https://google.com");
});
app.use(function (req, res, next) {
res.status(404).send("<h1>Sorry nothing found!<h1>");
});
app.listen(port, () => {
console.log(`Express app listening at http://localhost:${port}`);
});
And that’s how to perform redirect using Express framework.
Conclusion
Both NodeJS native module and Express framework are able to perform a response redirection, so you don’t have to use Express just for its redirect feature.