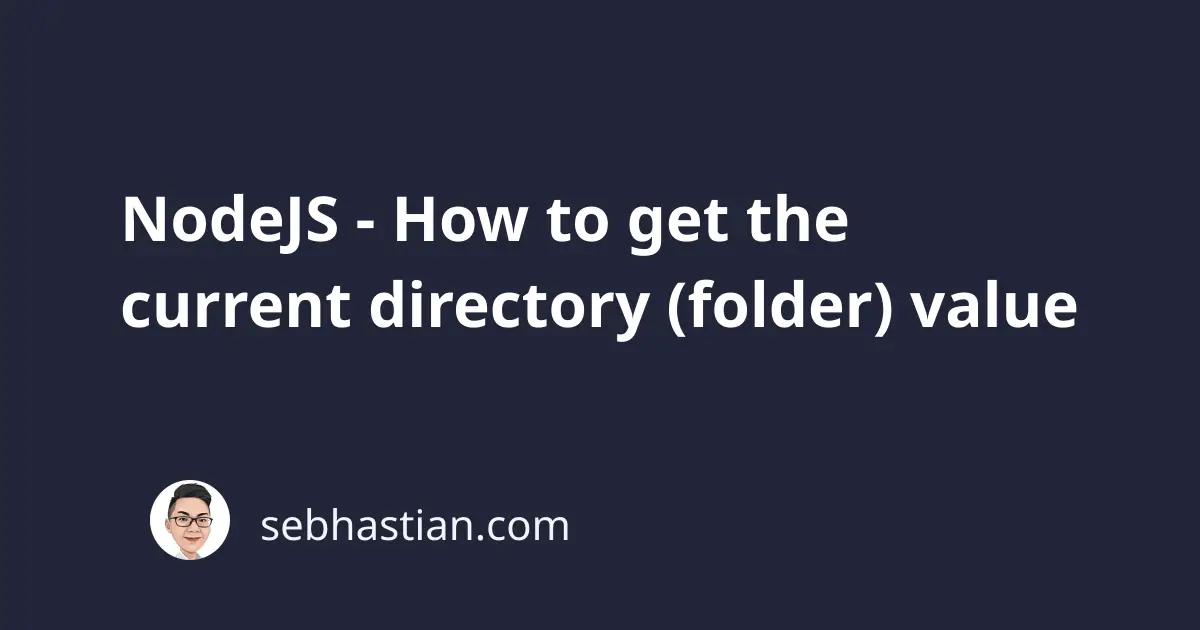
Sometimes, you need to get a string representing the current directory or folder your JavaScript code is being executed.
There are two ways you can get the current directory in NodeJS:
- Using the special variable
__dirname
- Using the built-in method
process.cwd()
This tutorial will show you how to use both ways. Let’s look at __dirname
first.
Get current directory with __dirname
The __dirname
variable is a special variable available when you run JavaScript code using NodeJS.
The code below shows how you can use the special variable:
console.log("Current directory:", __dirname);
The variable will print the path to the directory, starting from the root directory.
Here’s the output on my computer:
Current directory: /Users/nsebhastian/Desktop/test
And just like that, you can get the current working directory in NodeJS. Let’s look at process.cwd()
method next.
Get current directory with process.cwd()
The process.cwd()
method works just like the __dirname
variable. It prints the path to the current working directory from the root directory:
console.log("Current directory:", process.cwd());
// Current directory: /Users/nsebhastian/Desktop/test
Exclusively get the current directory
When you need only the current directory where the script is being executed, you can use the built-in path
module:
const path = require("path");
The path
module has the basename()
method, which returns only the last portion of a path. The last portion of the __dirname
variable for my computer is test
so it will be returned by the method:
const path = require("path");
const directoryName = path.basename(__dirname);
console.log(directoryName); // test
And that’s how you can get the string representing the current directory or folder in NodeJS.