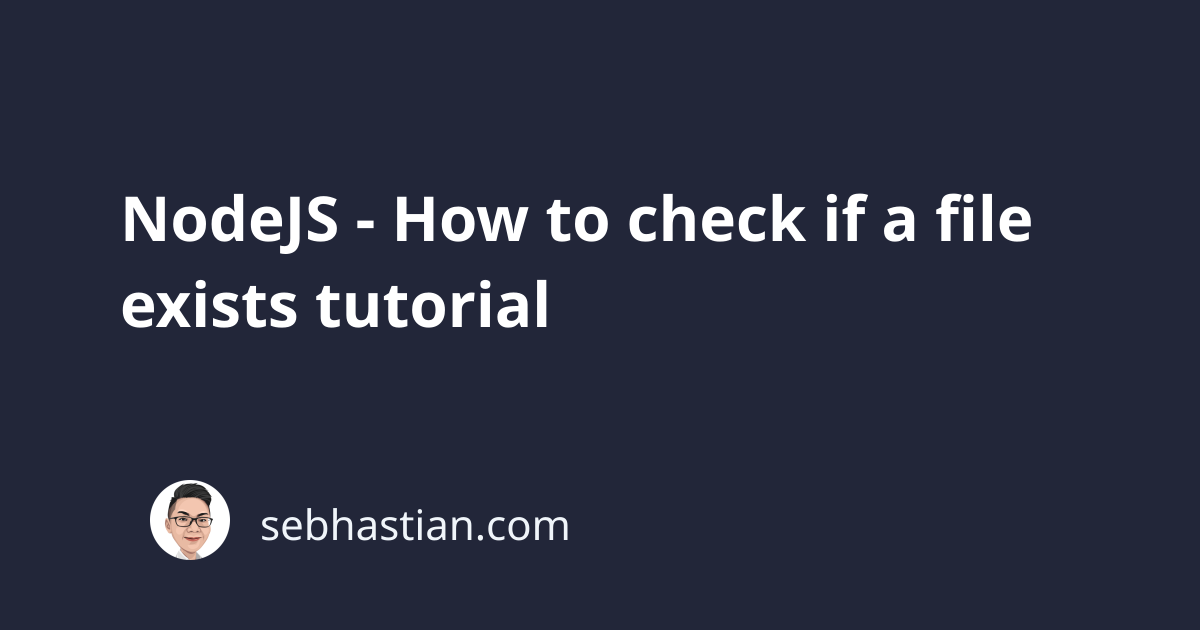
In NodeJS, You can check if a certain file exists under your filesystem by using the file system module, under the alias fs
:
const fs = require("fs");
There are four methods that you can use to check if a certain file or folder exists inside the fs
module:
fs.existsSync()
fs.exists()
fs.accessSync()
fs.access()
This tutorial will explain each method with example code below. Let’s start with fs.existsSync()
method.
Checking file existence with existsSync()
The fs.existsSync()
method allows you to check for the existence of a file by tracing if a specified path can be accessed from the current directory where the script is executed. It returns true
when the path exists and false
when it’s not.
The fs.existsSync()
is a synchronous method, which means it stops the execution of JavaScript code until the method finish its process. Suppose you have a folder with the following files:
project
├── index.html
└── util.js
Let’s say you want to check for the existence of index.html
inside the project
folder using NodeJS script inside util.js
file. Here’s how you can do it:
// util.js
const fs = require("fs");
const path = "./index.html";
if (fs.existsSync(path)) {
// path exists
console.log("exists:", path);
} else {
console.log("DOES NOT exist:", path);
}
Now you only need to run util.js
from the console using node util.js
:
$ node util.js
path exists: ./index.html
The existsSync()
method can also check for the existence of a folder. The following code checks if the assets/
folder exists or not:
const fs = require("fs");
const path = "./assets";
if (fs.existsSync(path)) {
// path exists
console.log("exists:", path);
} else {
console.log("DOES NOT exist:", path);
}
Create the assets/
folder if you want to see it logged to the console.
The existsSync()
method has an asynchronous version called exists()
which you’re going to learn about next.
Checking file existence with exists()
The fs.exists()
method checks for the existence of a path asynchronously.
The method accepts two parameters:
- The
path
to check - The
callback
function to execute after checking, passing boolean valueisExist
to the function as its argument
Note: This method is already deprecated in recent NodeJS version because the callback
function should pass two arguments, the first being the error
object, and the second the isExist
argument. Still, I will leave it here for you to learn.
For example, to check if index.html
file exists in the current folder, you can write the following code:
const fs = require("fs");
const path = "./index.html";
fs.exists(path, function (isExist) {
if (isExist) {
console.log("exists:", path);
} else {
console.log("DOES NOT exist:", path);
}
});
But since the method is already deprecated, you are recommended to use the fs.existsSync()
method instead.
Checking file existence with accessSync()
The fs.accessSync()
method is used to check for a user’s permission to a specified file or folder. When the file or folder is not found, it will throw an error that you need to catch using the try..catch
block.
The method accepts two parameters as follows:
- The
path
of the file you want to check - The
mode
to check for (explained further below)
The mode
parameter is optional. It defines how the check process will be executed by accessSync()
. The default value is fs.constants.F_OK
which checks for the existence of the file.
You can also use the following constants for the mode
parameter:
fs.constants.R_OK
to check for read permissionfs.constants.W_OK
to check for write permissionfs.constants.X_OK
to check for execute permission
These constants are also known as Node File Access Constants
The code below checks for the existence of file.txt
file:
const fs = require("fs");
const path = "./file.txt";
try {
fs.accessSync(path);
console.log("exists:", path);
} catch (err) {
console.log("DOES NOT exist:", path);
console.error(err);
}
If you want to check for write and read access, you can pass the mode
parameter with bitwise OR
operator as follows:
const fs = require("fs");
const path = "./file.txt";
try {
fs.accessSync(path, fs.constants.R_OK | fs.constants.W_OK);
console.log("can read/write:", path);
} catch (err) {
console.log("no access:", path);
console.error(err);
}
And that’s how you use the accessSync()
method. Let’s check on the asynchronous version of the method called access()
next.
Checking file existence with access()
The fs.access()
method allows you to check for the existence or permissions of a specified file or folder asynchronously.
The method accepts three parameters, two of them are the same as accessSync()
parameters:
- The
path
of the file you want to check - The
mode
to check for (seeaccessSync()
method for detail) - The
callback
function to execute, passing theerror
object for any error encountered in the process
The code below shows how you can use the fs.access()
method to check for the existence of the file.txt
file:
const fs = require("fs");
const path = "./file.txt";
fs.access(path, function (error) {
if (error) {
console.log("DOES NOT exist:", path);
console.error(error);
} else {
console.log("exists:", path);
}
});
Finally, you can pass the mode
as the second parameter right after the path
parameter:
const fs = require("fs");
const path = "./file.txt";
fs.access(path, fs.constants.R_OK | fs.constants.W_OK, function (error) {
if (error) {
console.error("no access:", path);
console.error(error);
} else {
console.log("can read/write:", path);
}
});
Conclusion
Now you know all about the four methods to check if a file exists using NodeJS. It’s recommended to use fs.existsSync()
if you only need to check for the existence of a file.
When you need to check for specific permissions as well, you can use either fs.accessSync()
or fs.access()
method. Don’t use fs.exists()
method because it’s already marked as deprecated.