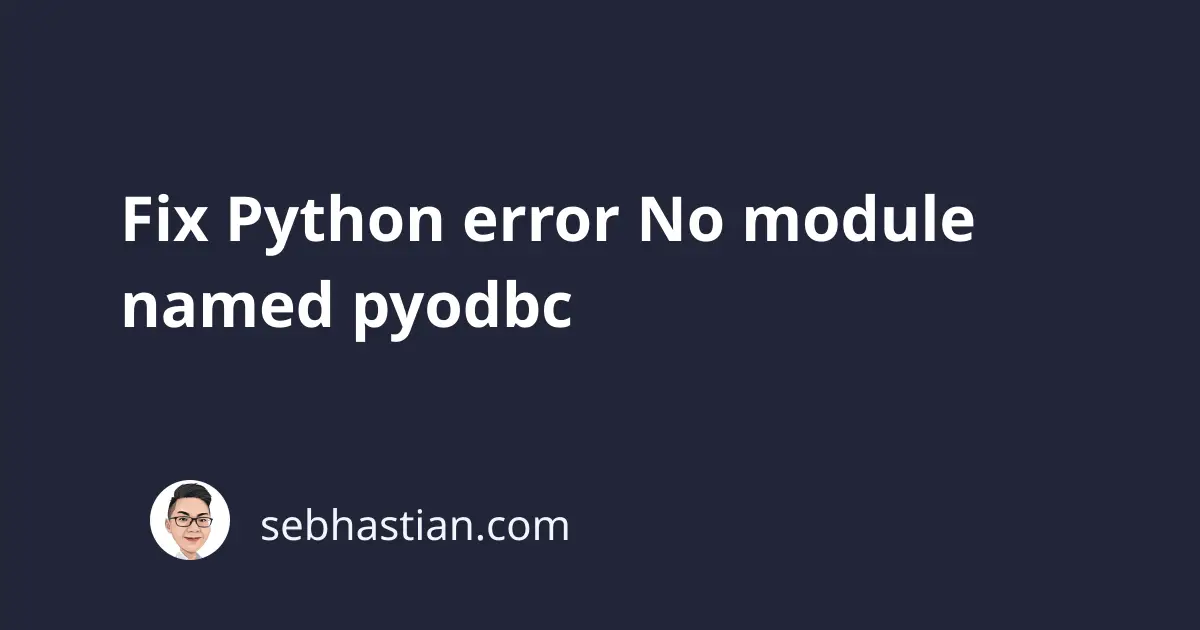
If you use the pyodbc
library in Python, then you might encounter the following error:
ModuleNotFoundError: No module named 'pyodbc'
This error occurs when Python can’t find the pyodbc
module from the pip
packages folder.
If you have installed pyodbc, then it’s possible that you have multiple Python versions, and the version used to run your code can’t find the module.
This tutorial will show you examples that cause this error and how to fix it.
1. Make sure pyodbc is installed
Before you try anything else, make sure that pyodbc is installed by running one of the following commands:
pip install pyodbc
# If you have pip3:
pip3 install pyodbc
# If no pip in PATH:
python -m pip install pyodbc
python3 -m pip install pyodbc
# Windows
py -m pip install pyodbc
Once the package is installed, try importing the module as follows:
import pyodbc
Depending on your Operating System, installing pyodbc might require additional steps.
For example, because I use Apple Macbook with M1 chip, I get this new error after installing:
Traceback (most recent call last):
File "main.py", line 1, in <module>
import pyodbc
ImportError:
symbol not found in flat namespace '_SQLAllocHandle'
To fix this issue, I reinstalled pyodbc with the following command:
pip3 install --no-binary :all: pyodbc --force-reinstall
You need to consult the pyodbc installation doc to see if there’s any extra step that you need to do to install it properly.
If you didn’t see any error, then it means Python is able to find the pyodbc package and import it.
2. Check if you have multiple Python versions installed
If you still see the error, then you may have multiple versions of Python installed on your system.
You can check this by running the which -a python
and which -a python3
commands from the terminal:
$ which -a python
/usr/local/bin/python
$ which -a python3
/usr/local/bin/python3
/usr/bin/python3
In the example above, there are multiple versions of Python installed on the system:
- Python 2 installed on
/usr/local/bin/python
- Python 3 installed on
/usr/local/bin/python3
and/usr/bin/python3
Each Python distribution is bundled with a specific pip
version. If you want to install a module for Python 2, use pip
. Otherwise, use pip3
for Python 3.
The problem arise when you install pyodbc for Python 2 but runs the code using Python 3, or vice versa:
# Install pyodbc for Python 2
$ pip install pyodbc
# Run the code with Python 3
$ python3 main.py
# Or Install pyodbc for Python 3
$ pip3 install pyodbc
# Run the code with Python 2
$ python main.py
If you install pyodbc for Python 2, then you need to run the code using Python 2 as well:
$ pip install pyodbc
$ python main.py
Having multiple Python versions can be confusing, so you need to carefully inspect how many Python versions you have on your computer.
But once you use the connected pip
and python
versions, you should be able to import pyodbc successfully.
3. No module named pyodbc in Visual Studio Code (VSCode)
If you use VSCode integrated terminal to run your code, you might get this error even when pyodbc is already installed.
This means the python
and pip
versions used by VSCode differ from the one where you install pyodbc.
You can check this by running the following code:
import sys
print(sys.executable)
The print
output will show you the absolute path to the Python used by VSCode. For example:
/path/to/python3
Copy the path shown in the terminal and add -m pip install pyodbc
as follows:
/path/to/python3 -m pip install pyodbc
The command will install pyodbc for the Python interpreter used by VSCode. Once finished, try running your code again. It should work this time.
Conclusion
Most likely, you see the No module named pyodbc
error because you didn’t install the pyodbc
package from pip
, or you installed the package on a different version of Python.
The steps shown in this article should help you fix this error.
I hope this article is helpful. Happy coding! 👋