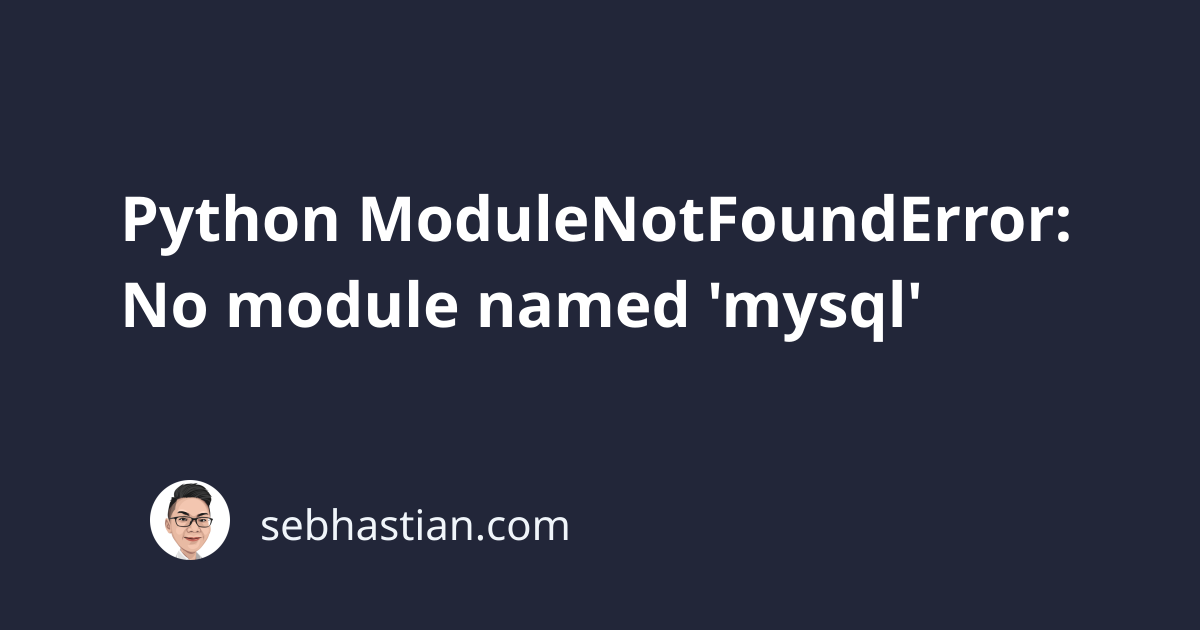
One error that you might encounter when running Python code that uses MySQL database is:
ModuleNotFoundError: No module named 'mysql'
This error occurs because the Python compiler can’t find a package named mysql
in your Python environment.
Let’s see an example that causes this error and how to fix it.
How to reproduce this error
Suppose you try to connect to a MySQL database using Python with the following code:
import mysql.connector
# Connect to MySQL database server
db = mysql.connector.connect(
host="127.0.0.1",
port=3306,
user="root",
password="root")
If you didn’t have the mysql-connector-python
module installed on your system, you’ll get the following error:
Traceback (most recent call last):
File "main.py", line 1, in <module>
import mysql.connector
ModuleNotFoundError: No module named 'mysql'
This is because mysql.connector
is a third-party module that’s not included in Python by default.
How to fix this error
To resolve this error and get the mysql.connector
module working, you need to install the mysql-connector-python
package using pip
as follows:
pip install mysql-connector-python
# For pip3:
pip3 install mysql-connector-python
You can also install the MySQL Connector/Python library through other distributions as listed here.
Once the installation is finished, try to run the script with the python3
command again. It should work this time.
Sometimes, you may also encounter a new error saying invalid syntax
as follows:
python main.py
Traceback (most recent call last):
File "main.py", line 1, in <module>
import mysql.connector
#.....
f"This connection is using {tls_version} which is now "
^
SyntaxError: invalid syntax
The error above is because MySQL Connector/Python library has dropped the support for Python 2 in version 8.0.23. You can see v8.0.23 changelog for more information.
If you’re using Python 2, then the latest version of MySQL Connector/Python library that you can use is version 8.0.22.
You need to uninstall the current library and then install the exact version using pip
as shown below:
pip uninstall mysql-connector-python
pip install mysql-connector-python==8.0.22
Now the library should be able to run both in Python 2 or Python 3 environment.
Install commands for other environments
The install command might differ depending on what environment you used to run Python code.
Here’s a list of common install commands in popular Python environments:
# if you don't have pip in your PATH:
python -m pip install mysql-connector-python
python3 -m pip install mysql-connector-python
# Windows
py -m pip install mysql-connector-python
# Anaconda
conda install -c anaconda mysql-connector-python
# Jupyter Notebook
!pip install mysql-connector-python
Once the module is installed, you should be able to run the code without receiving this error.
Other common causes for this error
If you still see the error even after installing the module, it means that the mysql
module can’t be found in your Python environment.
There are several reasons why this error can happen:
- You may have multiple versions of Python installed on your system, and you are using a different version of Python than the one where MySQL Connector is installed.
- You might have MySQL Connector installed in a virtual environment, and you are not activating the virtual environment before running your code.
- Your IDE uses a different version of Python from the one that has MySQL Connector
Let’s see how to fix these errors.
Handling multiple versions of Python
If you have multiple versions of Python installed on your system, you need to make sure that you are using the specific version where the MySQL Connector module is available.
You can test this by running the which -a python
or which -a python3
command from the terminal:
$ which -a python3
/opt/homebrew/bin/python3
/usr/bin/python3
In the example above, there are two versions of Python installed on /opt/homebrew/bin/python3
and /usr/bin/python3
.
Suppose you run the following steps in your project:
- Install MySQL Connector with
pip
using/usr/bin/
Python version - Install Python using Homebrew, you have Python in
/opt/homebrew/
- Then you
import mysql.connector
in your code
The steps above will cause the error because MySQL Connector is installed in /usr/bin/
, and your code is probably executed using Python from /opt/homebrew/
path.
To solve this error, you need to run pip install mysql-connector-python
command again so that MySQL Connector is installed and accessible by the new Python version.
Next, you can also have MySQL Connector installed in a virtual environment.
Handling Python virtual environment
Python venv
package allows you to create a virtual environment where you can install different versions of packages required by your project.
If you are installing mysql-connector-python
inside a virtual environment, then the module won’t be accessible outside of that environment.
You can see if a virtual environment is activated or not by looking at your command prompt.
When a virtual environment is activated, the name of that environment will be shown inside parentheses as shown below:
In the picture above, the name of the virtual environment (base)
appears when the Conda virtual environment is activated.
You need to turn off the virtual environment so that pip
installs to your computer.
When your virtual environment is created by Conda, run the conda deactivate
command. Otherwise, running the deactivate
command should work.
Handle IDE using a different Python version
Finally, the IDE from where you run your Python code may use a different Python version when you have multiple versions installed.
For example, you can check the Python interpreter used in VSCode by opening the command palette (CTRL + Shift + P
for Windows and ⌘ + Shift + P
for Mac) then run the Python: Select Interpreter
command.
You should see all available Python versions listed as follows:
You need to use the same version where you installed MySQL Connector so that the module can be found when you run the code from VSCode.
Once done, you should be able to import MySQL Connector into your code.
Conclusion
In summary, the ModuleNotFoundError: No module named 'mysql'
error occurs when the MySQL Connector module is not available in your Python environment. To fix this error, you need to install MySQL Connector using pip
.
If you already have the module installed, make sure you are using the correct version of Python, deactivate the virtual environment if you have one, and check for the Python version used by your IDE.
By following these steps, you should be able to import mysql
module in your code successfully.
I hope this tutorial is helpful. Happy coding! 👍