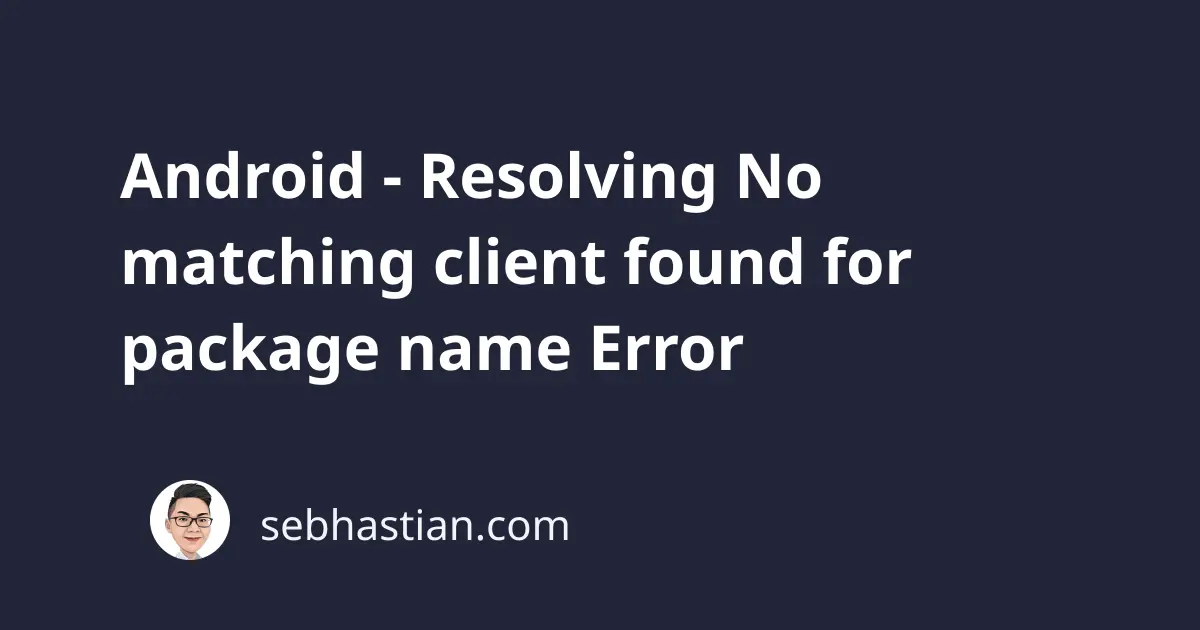
In Android development, the error No matching client found for package name
is triggered when you have a google-services.json
with a package_name
property that doesn’t match any found in your Android project.
For example, my Android project has a package name of org.metapx.kotlinfirebase
in the AndroidManifest.xml
file:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.metapx.kotlinfirebase">
But in my google-services.json
file, the package_name
property is defined as kotlinfirebas
:
{
"client": [
{
"client_info": {
"mobilesdk_app_id": "...",
"android_client_info": {
"package_name": "org.metapx.kotlinfirebas"
}
}
}
]
}
The typo above will cause the Android application build to fail:
Execution failed for task ':app:processDebugGoogleServices'.
> No matching client found for package name 'org.metapx.kotlinfirebase'
To fix the error, you need to make sure that the package_name
property of the google-services.json
file matches the actual package name of your Android project.
You can look into the AndroidManifest.xml
file to find your project’s package name.
No matching client found for different flavors
A single android application can have multiple buildTypes
or productFlavors
.
When you add a build type or flavor that adds an applicationIdSuffix
to the package name, you need to make sure that you have two different google-services.json
files for each package name.
For example, there’s a debug
build type in my Android project defined as follows:
buildTypes {
release {
minifyEnabled false
}
debug {
applicationIdSuffix ".dev"
debuggable true
}
}
This means that you need to have a second google-services.json
file that has the package_name
value of org.metapx.kotlinfirebase.dev
as shown below:
{
"client": [
{
"client_info": {
"mobilesdk_app_id": "...",
"android_client_info": {
"package_name": "org.metapx.kotlinfirebase.dev"
}
}
}
]
}
Also, remember that you need to place the google-services.json
file on the build type or flavor’s directory.
Android looks for the google-services.json
file in app
and its sub-directories as shown below:
Androidapp/app/google-services.json
Androidapp/app/src/google-services.json
Androidapp/app/src/debug/google-services.json
Androidapp/app/src/Debug/google-services.json
# Androidapp/app/src/buildType/google-services.json
# Androidapp/app/src/productFlavor/google-services.json
As you add more build types or product flavors, the list of directories that Android scans to look for the google-services.json
file will increase.
To summarize, the error No matching client found for package name
happens when you have one or more package names that don’t have a matching package_name
value in the google-services.json
file.
You need to make sure that the package_name
properties of your google-services.json
files match the actual package names of your builds and flavors.