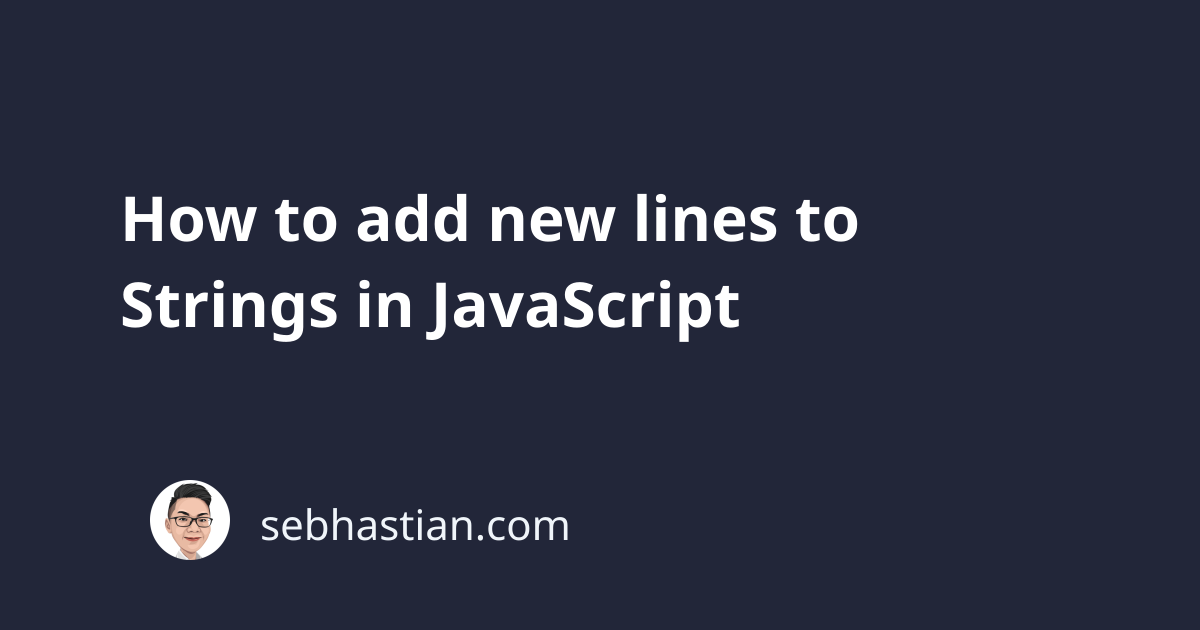
There are two ways to add a new line in JavaScript depending on the output you wish to achieve.
This tutorial will show you how to add a new line both for the JavaScript console and the DOM.
Adding new lines to the console output
To create a multi line strings when printing to the JavaScript console, you need to add the new line character represented by the \n
symbol.
Add the new line character in the middle of your string like this:
let str = "Hello World!\nThis is my string";
console.log(str);
The \n
symbol will create a line break in the string, creating a multi line string as shown below:
Hello World!
This is my string
Alternatively, you can also add a new line break using Enter
when you use the template literals syntax.
A template literals string is enclosed within backticks (``) instead of quotes (’’) like this:
let str = `Hello World!
This is my string
Adding new line using template string`;
console.log(str);
The output of the code above will be as follows:
Hello World!
This is my string
Adding new line using template string
You can add as many line breaks as you need in your string.
Next, let’s learn how to add new lines to the DOM output
Adding new lines to the DOM output
When you need to output a new line for a web page, you need to manipulate the DOM and add the <br>
HTML element so that the text will be printed below.
For example, you can write a JavaScript command as follows:
document.write("Hello World!<br/>This is my string");
The output to the <body>
tag will be as follows:
<body>
Hello World!
<br />
This is my string
</body>
Knowing this, you can manipulate the text of an HTML element to add a new line by changing the innerHTML
property and adding the <br/>
element.
The code in <script>
tag below shows how you add a new line to a <div>
with the id myDiv
:
<body>
<div id="myDiv"></div>
<script>
let element = document.getElementById("myDiv");
element.innerHTML = "Hello World!<br/>This is my string";
</script>
</body>
The output to the <div>
tag will be as follows:
<div id="myDiv">
Hello World!
<br />
This is my string
</div>
When generating DOM output, you can’t use the line breaks created by pressing Enter
or the \n
symbol.
Without <br>
tags, the string will appear on the same line.
And that’s how you can add a new line using JavaScript for different outputs. Nice work! 👍