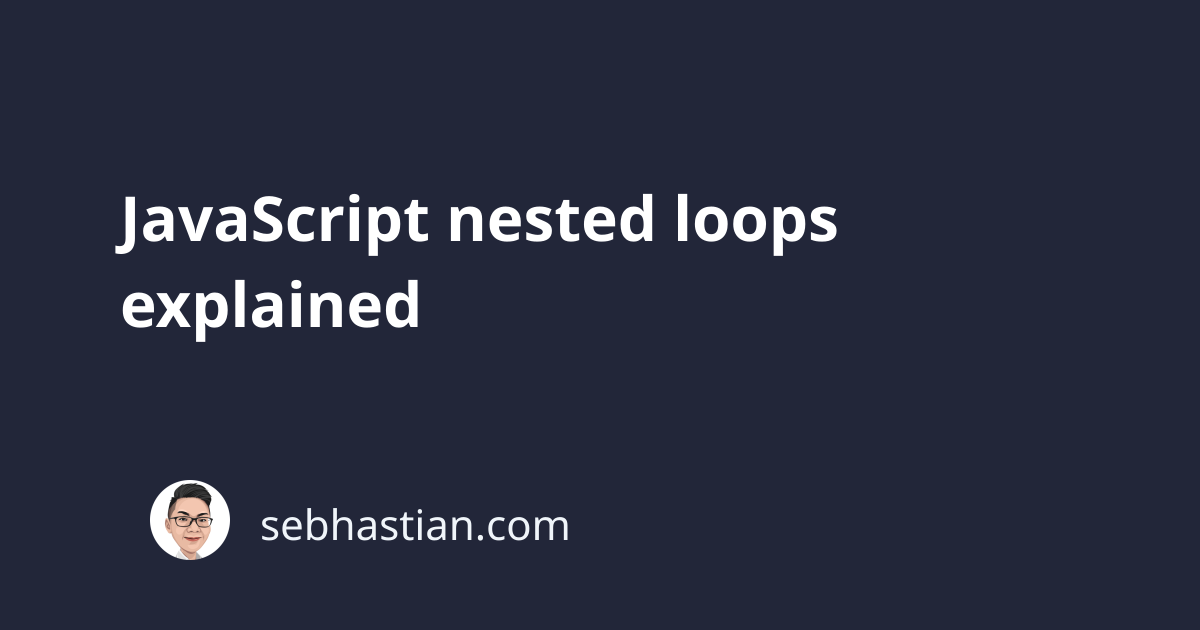
Nested loops is a coding pattern that enables you to perform a looping code inside the first loop. You may have a regular for
loop like this:
for (let i = 0; i < 2; i++) {
console.log("- First level loop");
}
You can create a second level loop that runs inside the first-level loop by creating another for
loop inside the first one as follows:
for (let i = 0; i <= 2; i++) {
console.log("- First level loop");
for (let j = 0; j <= 3; j++) {
console.log("-- Second level loop");
}
}
The code above will generate the following output:
- First level loop
-- Second level loop
-- Second level loop
-- Second level loop
-- Second level loop
- First level loop
-- Second level loop
-- Second level loop
-- Second level loop
-- Second level loop
- First level loop
-- Second level loop
-- Second level loop
-- Second level loop
-- Second level loop
As you can see in the output sample above, the second-level for
loop is executed for each first-level loop
. The i
and j
variables are indicators of the matrix elements used commonly in mathematics.
The nested loops can be done inside a for
loop or a while
loop, although the for
loop is more common:
let i = 0;
while (i <= 2) {
console.log("- First level loop");
i++;
let j = 0;
while (j <= 3) {
console.log("-- Second level loop");
j++;
}
}
You can also create more than two levels of nested loops, but it’s not recommended because it will confuse even seasoned developers.
Iterating through a multi dimensional array with nested loops
The nested loops can also be used to iterate through a two-dimensional array. Suppose you have an array that contains three nested arrays as follows:
let arr = [
[1, 2],
[3, 4],
[5, 6],
];
If you create a single loop, you will loop through the outer array and log the nested arrays as follows:
let arr = [
[1, 2],
[3, 4],
[5, 6],
];
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
The output will be as shown below:
[ 1, 2 ] # arr[0]
[ 3, 4 ] # arr[1]
[ 5, 6 ] # arr[2]
If you want to log each of the elements inside the nested arrays, you need to iterate through the nested arrays by using arr[i].length
for the condition of the loop:
let arr = [
[1, 2],
[3, 4],
[5, 6],
];
for (let i = 0; i < arr.length; i++) {
for (let j = 0; j < arr[i].length; j++) {
console.log(arr[i][j]);
}
}
The output will be as follows:
1 # arr[0][0]
2 # arr[0][1]
3 # arr[1][0]
4 # arr[1][1]
5 # arr[2][0]
6 # arr[2][1]
Nested loops pattern is a bit complicated, and even seasoned developers can be confused with it. To understand what your code does, you need to first break the pattern and visualize each of the loop code. For example, you can first log the output of the first-level loop.
After you understand the first-level loop with confidence, you can start visualizing the second-level loop.