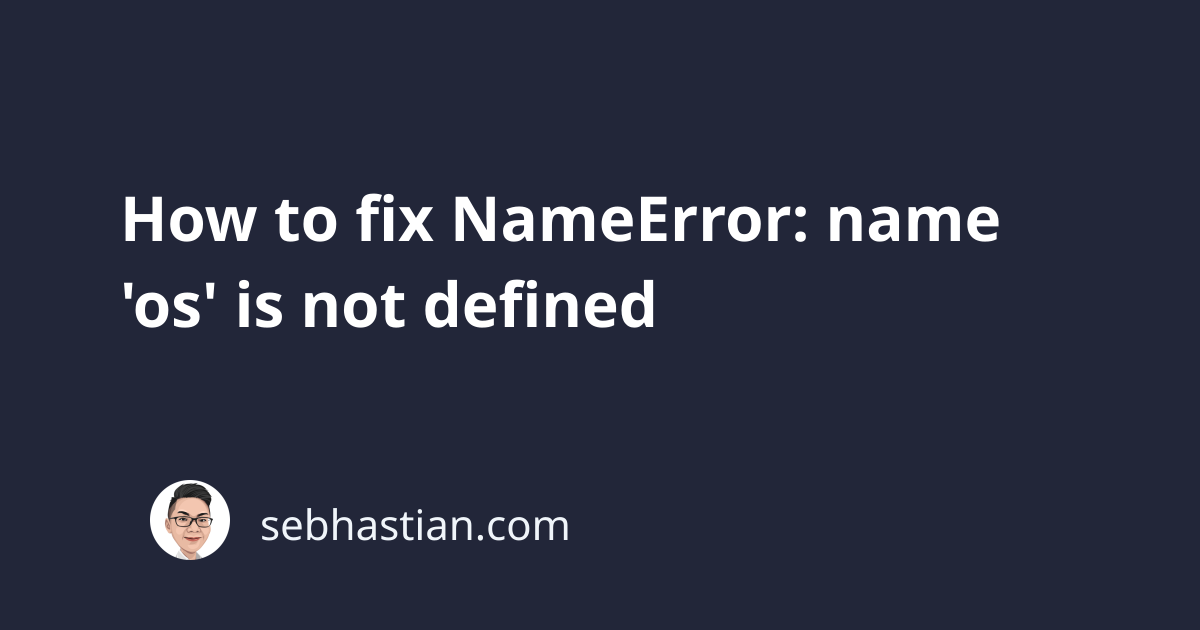
While running Python applications, you might encounter the following error:
NameError: name 'os' is not defined
This error occurs when Python can’t find the os
module in the current scope of your code.
This tutorial shows you examples that cause this error and how to fix it.
How to reproduce this error
Suppose you call the path.join()
function of the os
module as shown below:
user_path = os.path.join("/users", "1002")
The output will be:
Traceback (most recent call last):
File "main.py", line 1, in <module>
user_path = os.path.join("/users", "1002")
NameError: name 'os' is not defined
This error occurs because the os
module needs to be imported into your source code before you can use its functions and attributes.
How to fix this error
To resolve this error, you only need to import the os
module in your source code as follows:
import os
user_path = os.path.join("/users", "1002")
Notice that we receive no error this time, and the path.join()
function works.
Error in Django application
If you recently upgraded your django
package, it has been known that this error occurs because the import os
code in the settings.py
has been replaced with pathlib
.
The settings.py
used to import the os
module as follows:
import os
BASE_DIR = os.path.dirname(...)
But the most recent version of Django used Path
instead of os
as shown below:
from pathlib import Path
BASE_DIR = Path(__file__).resolve().parent.parent
Some Django tutorials still use the os
module, so you might get this error when you copy the code from older tutorials.
If you need to use the os
module in settings.py
, then you can add the import os
statement at the top of the file as follows:
import os
from pathlib import Path
BASE_DIR = Path(__file__).resolve().parent.parent
That should resolve the error when you run the Django application.
Make sure you’re not importing os in a nested scope
To avoid this error, make sure you’re not importing the os
module in a nested scope, such as inside an if-else
, try-except
, or a function block.
For example, suppose you import the module in a function:
def get_os_info():
import os
print(os.name)
user_path = os.path.join("/users", "1002") # ❌
The os
module is imported inside the get_os_info()
function so you’ll get the error when you call os
outside of the function.
To resolve this error, you need to move the import
statement outside the function block:
import os
def get_os_info():
print(os.name)
user_path = os.path.join("/users", "1002") # ✅
get_os_info() # ✅
By moving the import
statement outside the function body, the os
module can be used in the nested and main scope of the file.
Conclusion
The name 'os' is not defined
error occurs when you use the os
module functions and attributes without importing the module.
To resolve this error, import the os
module at the top of your file.
I hope this tutorial helps. Happy coding! 👋