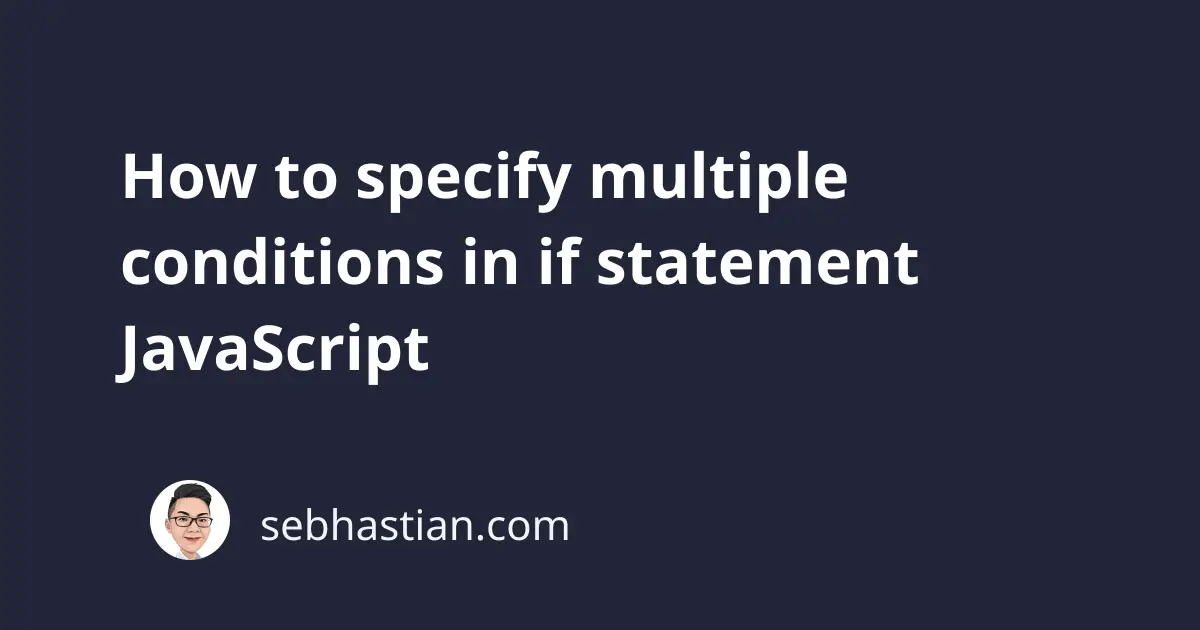
The most common JavaScript if
statement usually tests only one condition before executing code in the if
body.
To define multiple conditions in the statement header, you need to use the logical AND operator or the logical OR operator.
Using logical AND operator
The logical AND &&
operator can be used when all conditions need to be satisfied to run the if
block.
For example, suppose you have a variable named age
that needs to be a number type and the value is equal to or greater than 17. You can use the logical AND operator to specify two conditions as follows:
let age = "15";
if (typeof age === "number" && age >= 17) {
console.log("All conditions passed.");
} else {
console.log("You need a number equal to or higher than 17");
}
In the if
statement above, the first condition checks whether the age
variable is of number
type, and the second condition tests whether the variable’s value is equal to or greater than 17.
Because we used a logical AND operator, both conditions must return true
for the if
block to run.
You can specify as many conditions as you want by adding another logical AND operator to the statement.
The code below also tests if the age
variable is less than 55:
let age = "15";
if (typeof age === "number" && age >= 17 && age < 55) {
console.log("All conditions passed.");
} else {
console.log("You need a number equal to or higher than 17");
console.log("And less than 55");
}
If you have one condition that returns false
, then the if
block will be skipped.
Next, let’s see how to specify multiple conditions using the logical OR operator.
Using logical OR operator
The logical OR ||
operator will run the if
block when at least one condition returns true
between several conditions.
Let’s see another example. Suppose you’re writing a program for a bank, and you have a special offer for a customer that has at least 2000
USD in deposits or has spent at least 1000
USD.
Here’s how you check if a customer passes one of these conditions:
let customerDeposits = 1000;
let customerSpendings = 200;
if (customerDeposits >= 2000 || customerSpendings >= 1000) {
console.log("Congratulations! You're eligible for a special offer!");
} else {
console.log("Sorry, you're not eligible yet!");
}
Here, the if
block runs when at least one condition returns true
. Otherwise, the if
block will be skipped.
Use parentheses to prioritize specific condition
When you have multiple conditions in an if
statement, you might also want to prioritize specific condition(s) to control the way the conditions are tested.
This is especially useful when you have both logical AND and OR in your code as follows:
if (false && false || true) {
console.log("Execute the IF block");
} else {
console.log("Execute the ELSE block");
}
The logical AND operator takes precedence over the OR operator. In the above example, the false && false
condition will be tested first, which returns true
.
Because true || true
returns true
, the if
block is executed.
You can change the execution order by placing parentheses next to the &&
operator like this:
if (false && (false || true)) {
console.log("Execute the IF block");
} else {
console.log("Execute the ELSE block");
}
The parentheses cause JavaScript to test (false || true)
first. The condition returns true
, and because false && true
returns false
, the else block is executed.
Now you’ve learned how using parentheses can change the order of the tests. Nice work!
Conclusion
To specify multiple conditions in an if
statement, you can use the logical AND operator or the logical OR operator.
If you need all conditions to be satisfied, use the logical AND operator. If you only need at least one condition to be satisfied, use the logical OR operator.
You can use parentheses to prioritize certain conditions, changing the order of the tests.
And that’s all for this article. Happy coding! 👍