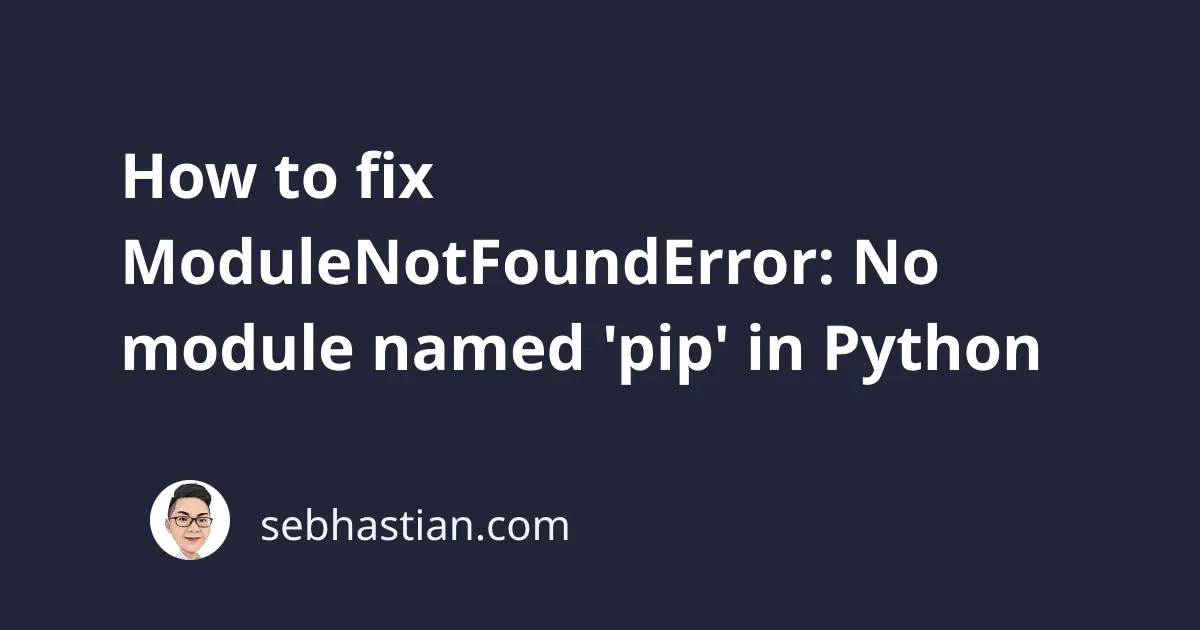
One error that you might encounter while using Python is:
ModuleNotFoundError: No module named 'pip'
This error occurs when the pip
module is not available in your Python environment.
This tutorial shows an example that causes this error and how to fix it.
How to reproduce the error
Suppose you want to import the pip
module in your source code as follows:
import pip
print(pip.__version__)
But you get the following error when running the code:
Traceback (most recent call last):
File "main.py", line 1, in <module>
import pip
ModuleNotFoundError: No module named 'pip'
This error occurs when the pip
module is not available in your Python environment.
The pip
module is usually bundled with Python, so it should be available when you installed the Python program.
But if you see this error, then Python might have skipped building the pip
module when you install it.
How to fix this error
To resolve this error, you need to install the pip
module using the ensurepip
module.
Try to run one of the commands below:
python -m ensurepip
# For Python 3:
python3 -m ensurepip
# Windows:
py -m ensurepip
# For Linux, you can also use:
sudo apt install python3-pip
Once the module is installed, run one of the following commands to see if pip
is available:
pip -V
pip3 -V
# If pip not available in PATH, try:
python -m pip -V
python3 -m pip -V
If the command works, then you should be able to import pip
in your source code without receiving the error.
Alternative ways to install pip
If the command above doesn’t work, you can try using the get-pip.py
script to install the module.
First, download the script from https://bootstrap.pypa.io/get-pip.py into your computer. You need to open the context menu with right-click and select the ‘Save As..’ option.
Next, run the get-pip.py
script using Python from your terminal as follows:
python get-pip.py
# For Python 3:
python3 get-pip.py
# Windows:
py get-pip.py
You should have a similar output as follows:
You should be able to run pip
or pip3
from the terminal now.
If you still can’t access pip
, then try to install the module using commands specific to your Operating System:
# For Debian / Ubuntu
sudo apt update
sudo apt install python3-venv python3-pip
# For Fedora
sudo dnf update
sudo dnf install python3-pip python3-wheel
# For CentOS / RHEL
sudo yum update
sudo yum install python3 python3-pip
If you’re using Windows or macOS, you need to reinstall Python using the official installer, which should also take care of adding pip
to the system PATH.
Adding pip to PATH
If you can’t run pip -V
but able to run python -m pip -V
, that means the path to pip is not added to your PATH system.
In Windows, you can do this using the set PATH
command.
Get the location of Python using the where python
command as follows:
$ where python
Output:
where python
C:\Users\nsebhastian\AppData\Local\Programs\Python\Python310\python.exe
C:\Users\nsebhastian\AppData\Local\Microsoft\WindowsApps\python.exe
Next, add the location to python.exe
to your PATH as follows:
set PATH=%PATH%;C:\Users\nsebhastian\AppData\Local\Programs\Python\Python310
That should allow the command prompt to find the pip
module for the current session. If you want to add the location permanently to the PATH, use the setx
command instead of set
.
For Linux and macOS, run the export PATH
command followed by the $PATH
variable:
export PATH="$PATH:/usr/local/bin/python"
You need to add the absolute path to the Python location as well.
Conclusion
The ModuleNotFoundError: No module named 'pip'
occurs in Python when the pip
module is not available in the current Python environment.
To resolve this error, run the ensurepip
or get-pip.py
script that will install pip
to your system.
I hope this tutorial is helpful. See you in other tutorials! 👍