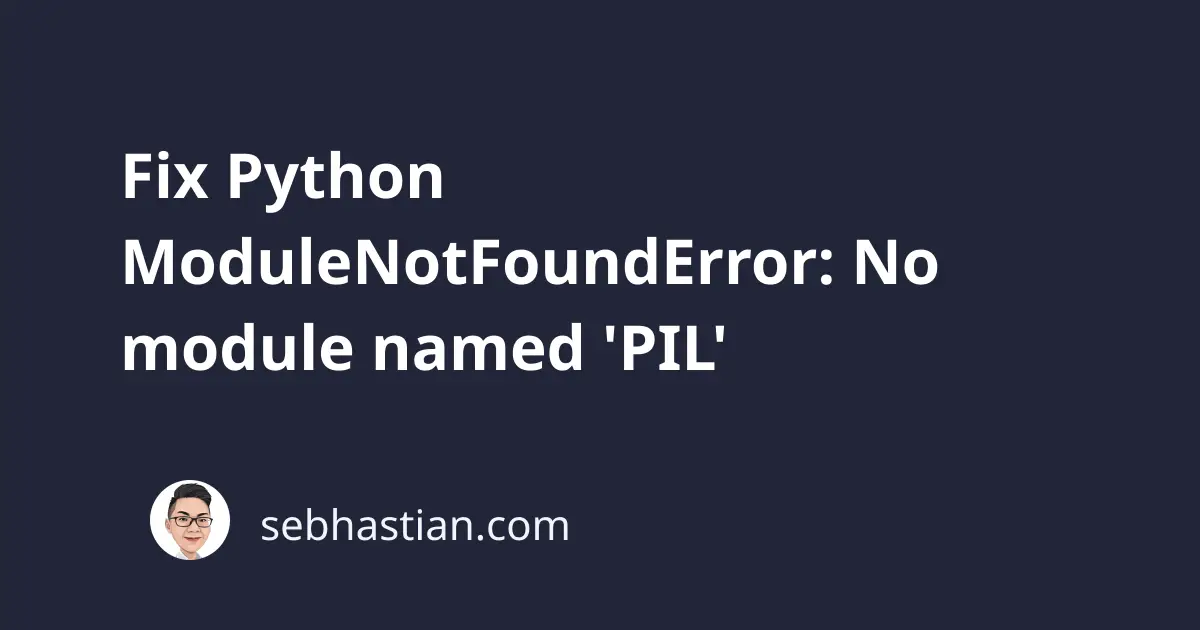
If you use the Python Imaging Library (PIL) in your code, you might encounter this error:
ModuleNotFoundError: No module named 'PIL'
This error occurs when you try to import the PIL
module without installing the Pillow package.
If you already have the Pillow package, then you may have multiple versions of Python installed on your computer, and Python is looking at the wrong folder for the package.
This article shows examples that cause the error and how to fix it.
1. Install the Pillow library
The PIL package has been forked to the Pillow package, so to import PIL
in your code, you need to install Pillow
.
First, you need to uninstall PIL because Pillow and PIL cannot co-exist in the same environment:
pip uninstall PIL
# Install pillow next:
pip install pillow --force-reinstall --no-cache
# Or with pip3:
pip3 install pillow --force-reinstall --no-cache
# If you don't have pip in PATH:
python -m pip install --upgrade pillow
python3 -m pip install --upgrade pillow
# If you use Anaconda or conda
conda install -c conda-forge pillow
# Installing from Jupyter Notebook
!pip install pillow
Once you installed Pillow, you can import PIL with the following code:
from PIL import Image
img = Image.open("test.png")
Please note that you can’t use import PIL
or import Image
. The latest Pillow version requires you to state the import exactly as from PIL import Image
.
If you use the module like this:
import PIL
img = PIL.Image.open("test.png")
You’ll get the following error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
img = PIL.Image.open("test.png")
^^^^^^^^^
AttributeError: module 'PIL' has no attribute 'Image'
You also need to replace import _imaging
as shown below:
# from:
import _imaging
# to:
from PIL.Image import core as _imaging
Now that you have Pillow installed, you should be able to use PIL without any errors.
If that doesn’t work, then you might have multiple Python versions installed on your computer.
2. Check if you have multiple Python versions installed
If you have multiple versions of Python installed on your system, you need to make sure that you are using the specific version where the pillow
module is installed.
You can test this by running the which -a python
and which -a python3
commands from the terminal:
$ which -a python
/usr/local/bin/python
$ which -a python3
/usr/local/bin/python3
/usr/bin/python3
In the example above, there are multiple versions of Python installed on the system:
- Python 2 installed on
/usr/local/bin/python
- Python 3 installed on
/usr/local/bin/python3
and/usr/bin/python3
Each Python distribution is bundled with a specific pip
version. If you want to install a module for Python 2, use pip
. Otherwise, use pip3
for Python 3.
The problem arise when you install pillow
for Python 2 but runs the code using Python 3, or vice versa:
# Install Pillow for Python 2
$ pip install pillow
# Run the code with Python 3
$ python3 main.py
# Or Install Pillow for Python 3
$ pip3 install pillow
# Run the code with Python 2
$ python main.py
If you install Pillow for Python 2, then you need to run the code using Python 2 as well:
$ pip install pillow
$ python main.py
Having multiple Python versions can be confusing, so you need to carefully inspect how many Python versions you have on your computer.
3. No module named PIL in Visual Studio Code (VSCode)
If you use VSCode integrated terminal to run your code, you might get this error even when Pillow is already installed.
This means the Python and pip
versions used by VSCode differ from the one where you install Pillow.
You can check this by running the following code:
import sys
print(sys.executable)
The print
output will show you the absolute path to the Python used by VSCode. For example:
/path/to/python3
Copy the path shown in the terminal and add -m pip install pillow
as follows:
/path/to/python3 -m pip install pillow
This will install pillow
for the Python interpreter used by VSCode. Once finished, try running your code again. It should work this time.
Conclusion
Most likely, you see the No module named PIL
error because you didn’t install the pillow
package from pip
, or you installed the package on a different version of Python.
The steps shown in this article should help you fix this error.
I hope this article is helpful. Happy coding! 👋