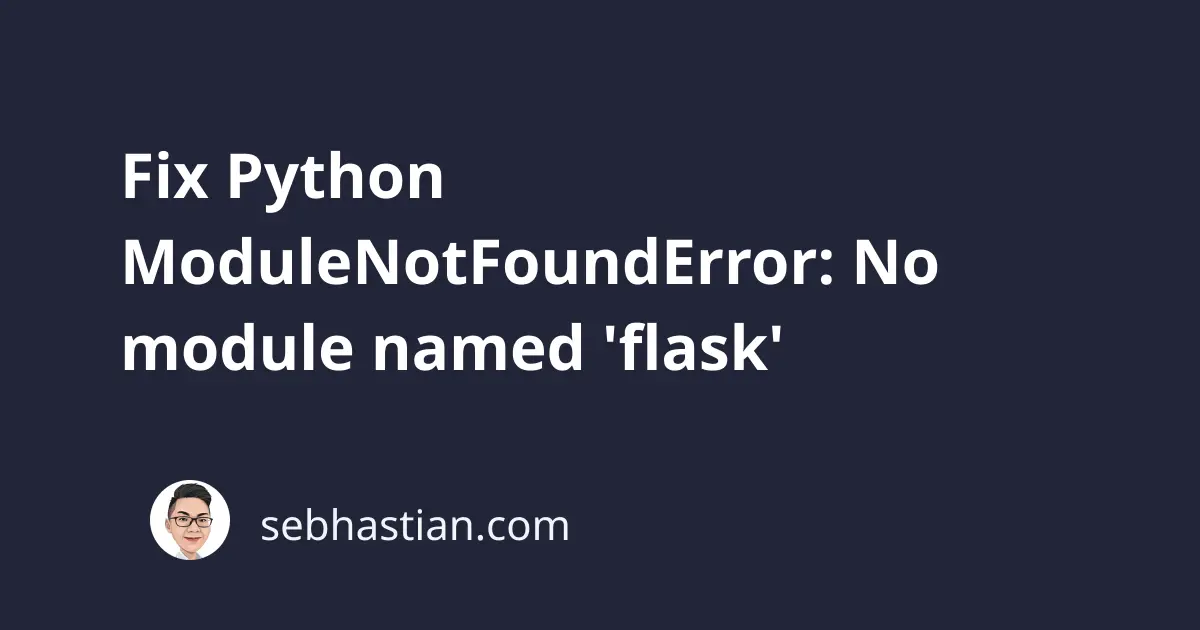
The ModuleNotFoundError: No module named 'flask'
error happens in Python when the flask
module can’t be imported. You may forget to install the flask module or it can’t be found in your Python environment.
To fix this error, you need to make sure that the flask
module exists and can be found. This article will show you how.
Installing flask
Suppose you have a script named app.py
with the following content:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "<p>Hello, World!</p>"
When you run the script using Python, the following error shows up:
Traceback (most recent call last):
File ...
from flask import Flask
ModuleNotFoundError: No module named 'flask'
To fix this error, you need to install the flask
module using pip, the package installer for Python.
Use one of the following commands depending on where you do the installation:
# Use pip to install flask
pip install flask
# Or pip3
pip3 install flask
# If pip isn't available in PATH
python -m pip install flask
# Or with python3
python3 -m pip install flask
# For Windows without pip in PATH
py -m pip install flask
# for Anaconda
conda install -c anaconda flask
# for Jupyter Notebook
!pip install flask
Alternatively, you can install it using the requirements.txt
file if you have one in your project.
You can use the requirements.txt
file with pip
as follows:
pip install -r requirements.txt
# or
pip3 install -r requirements.txt
Once installed, run the script that imports the flask
module again to see if the error has been resolved.
If you still see the error, that means the Python interpreter you used to run the script can’t find the module you installed.
One of the following scenarios may happen in your case:
- You have multiple versions of Python installed on your system, and you are using a different version of Python than the one where
flask
is installed. - You might have
flask
installed in a virtual environment, and you are not activating the virtual environment before running your code. - Your IDE uses a different version of Python from the one that has
flask
installed
Let’s see how to fix these errors.
Case#1 - You have multiple versions of Python
If you have multiple versions of Python installed on your system, you need to make sure that you are using the specific version where the flask
package is installed.
You can test this by running the which -a python
or which -a python3
command from the terminal:
$ which -a python
/opt/homebrew/bin/python
/usr/bin/python
In the example above, there are two versions of Python installed on /opt/homebrew/bin/python
and /usr/bin/python
.
Suppose you run the following steps in your project:
- Install
flask
withpip
using/usr/bin/
Python version - Install Python using Homebrew, now you have Python in
/opt/homebrew/
- Then add
from flask import Flask
in your code
The steps above will cause the error because flask
is installed in /usr/bin/
, and your code is probably executed using Python from /opt/homebrew/
path.
To solve this error, you need to run pip install flask
command again so that the package is installed and accessible by the new Python version.
Finally, keep in mind that you can also have pip
and pip3
available on your computer.
The pip
command usually installs module for Python 2, while pip3
installs for Python 3. Make sure that you are using the right command for your situation.
Next, you can also have the package installed in a virtual environment.
Case#2 - You are using Python virtual environment
Python venv
package allows you to create a virtual environment where you can install different versions of packages required by your project.
If you are installing flask
inside a virtual environment, then the module won’t be accessible outside of that environment.
Even when you never run the venv
package, Python IDE like Anaconda and PyCharm usually create their own virtual environment when you create a Python project with them.
You can see if a virtual environment is activated or not by looking at your command prompt.
When a virtual environment is activated, the name of that environment will be shown inside parentheses as shown below:
In the picture above, the name of the virtual environment (base)
appears when the Conda virtual environment is activated.
To solve this, you can either:
- Turn off the virtual environment so that
pip
installs to your computer - Install the
flask
in the virtual environment withpip
You can choose the solution that works for your project.
When your virtual environment is created by Conda, run the conda deactivate
command. Otherwise, running the deactivate
command should work.
To activate your virtual environment, use one of the following commands:
# For Conda:
conda activate <env_name>
# For venv:
source <env_name>/bin/activate
For Pycharm, you need to follow the Pycharm guide to virtual environment.
Case#3 - IDE using a different Python version
Finally, the IDE from where you run your Python code may use a different Python version when you have multiple versions installed.
For example, you can check the Python interpreter used in VSCode by opening the command palette (CTRL + Shift + P
for Windows and ⌘ + Shift + P
for Mac) then run the Python: Select Interpreter
command.
You should see all available Python versions listed as follows:
You need to use the same version where you installed flask
so that the module can be found when you run the code from VSCode.
If you use Pycharm, follow the Pycharm configuring Python interpreter guide.
Once done, you should be able to run the from flask import Flask
script in your code.
Conclusion
To conclude, the ModuleNotFoundError: No module named 'flask'
error occurs when the flask
package is not available in your Python environment.
To fix this error, you need to install flask
using pip
.
If you already have the module installed, make sure you are using the correct version of Python, activate the virtual environment if you have one, and check for the Python version used by your IDE.
By following these steps, you should be able to import flask
modules in your code successfully.