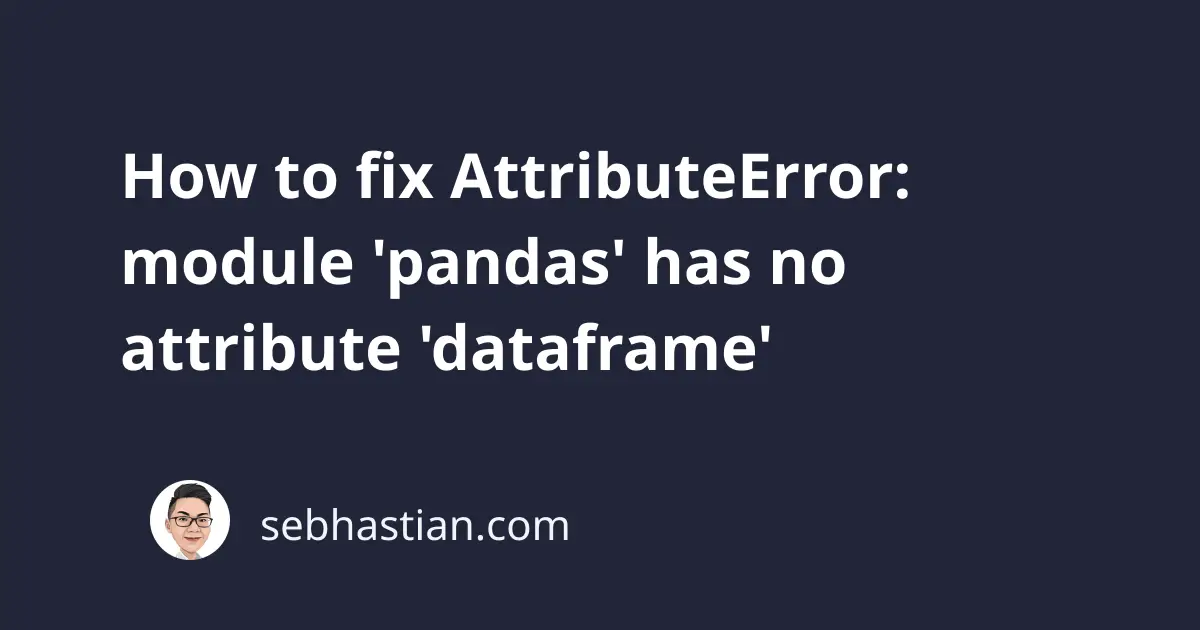
When creating a DataFrame object using pandas, you might encounter the following error:
AttributeError: module 'pandas' has no attribute 'dataframe'
This error occurs when Python can’t find a reference to the dataframe
attribute in the pandas
module.
There are three common causes for this error:
- Incorrectly typed
DataFrame
asdataframe
- You defined a
pandas
orpd
variable somewhere in your code - You named a file as
pandas.py
This tutorial shows how you can fix the causes of this error in practice.
1. You mistyped the DataFrame
attribute
Suppose you try to create a DataFrame object with the following code:
import pandas as pd
df = pd.dataframe({"distance": [3.6, 18.3, 21.5, 25.2]})
You’ll get the following error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
df = pd.dataframe({"distance": [3.6, 18.3, 21.5, 25.2]})
AttributeError: module 'pandas' has no attribute 'dataframe'.
Did you mean: 'DataFrame'?
This error occurs because you incorrectly typed the DataFrame
attribute as dataframe
with all lower cases.
The typing of the attribute must be exactly camel case as DataFrame
to avoid this error:
import pandas as pd
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
Once you fixed the typo, the error should now disappear.
2. You declared a pd
or pandas
variable
When you import the pandas
library and alias it as pd
, then the name pd
becomes a variable that binds to the pandas
module:
import pandas as pd
If you then declared a variable named pd
below the import
statement, then Python will overwrite the bindings, causing an error:
import pandas as pd
pd = "Hello!"
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
In the code above, the name pd
binds to a string instead of the pandas
module, and the error gets triggered when you call pd.DataFrame()
below it.
To resolve this error, you need to rename the variable pd
to something else as follows:
import pandas as pd
my_str = "Hello!" # Rename pd as my_str
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
Once you renamed the variable, the name pd
stays pointed to the pandas
module so the error doesn’t occur.
If you didn’t alias the pandas
module as pd
, then you can’t have a variable named pandas
somewhere below the import
statement.
3. You have a file named pandas.py
If you have a file named pandas.py
, then the statement import pandas
will import the pandas.py
file you have instead of the pandas library installed using pip
.
To resolve this error, you need to make sure you have no file named pandas.py
in your Python project.
You can rename the file to anything other than pandas.py
, and the error should be resolved.
Conclusion
You’ve now learned the three common causes for the AttributeError: module 'pandas' has no attribute 'dataframe'
as well as how to resolve them.
I hope this tutorial helps. Until next time! 🙌