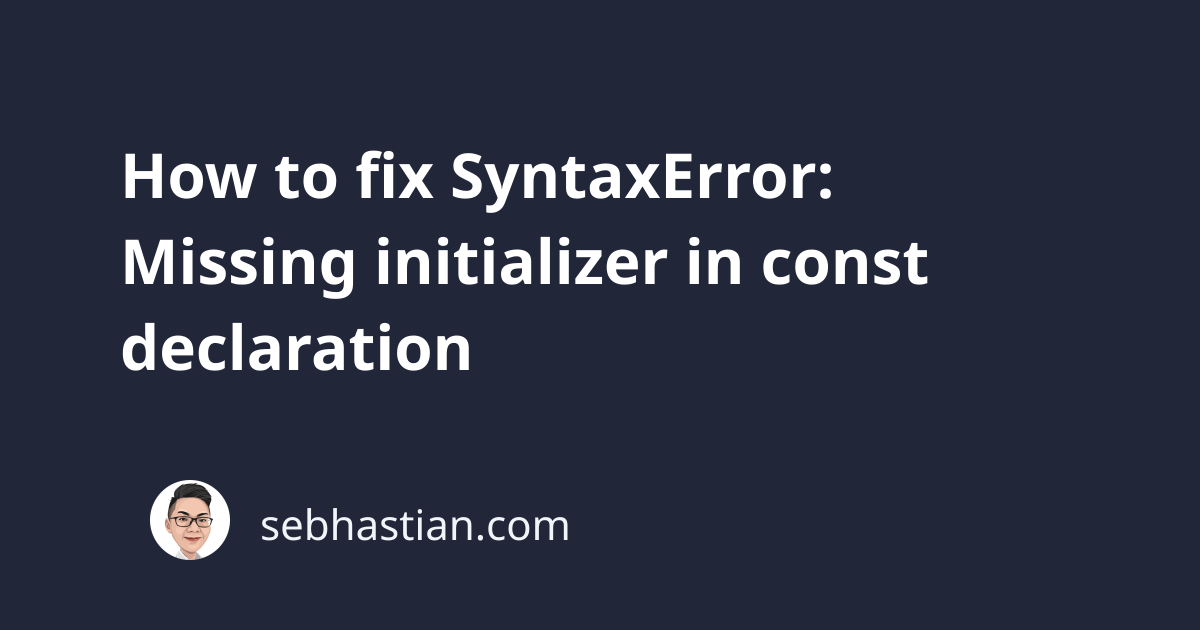
When you run JavaScript code, you might encounter an error as follows:
SyntaxError: Missing initializer in const declaration
This error occurs when you declare a constant variable without giving it an initial value. Here is some example code that causes this error:
const AGE; // ❌ Missing initializer in const declaration
When you declare a constant variable, you need to immediately assign a value to that variable as shown below:
const AGE = 27; // ✅
If you want to declare a variable without initializing a value, you can only create a variable using the let
or var
keyword.
This error makes sense because you can’t change the value of a constant variable once you declared it.
If you declare a constant without any value, the variable would have the value undefined
. This error wants to prevent you from creating a useless constant variable.
The same error also happens when you use TypeScript in your program. Suppose you declare a constant as follows:
const AGE: number;
When you run the code using the TypeScript compiler, you’ll get the same error.
You need to assign a value to the variable after the type declaration:
const AGE: number = 29;
After you initialize the constant value, the error should disappear.
I hope this tutorial helps. Happy coding! 👍