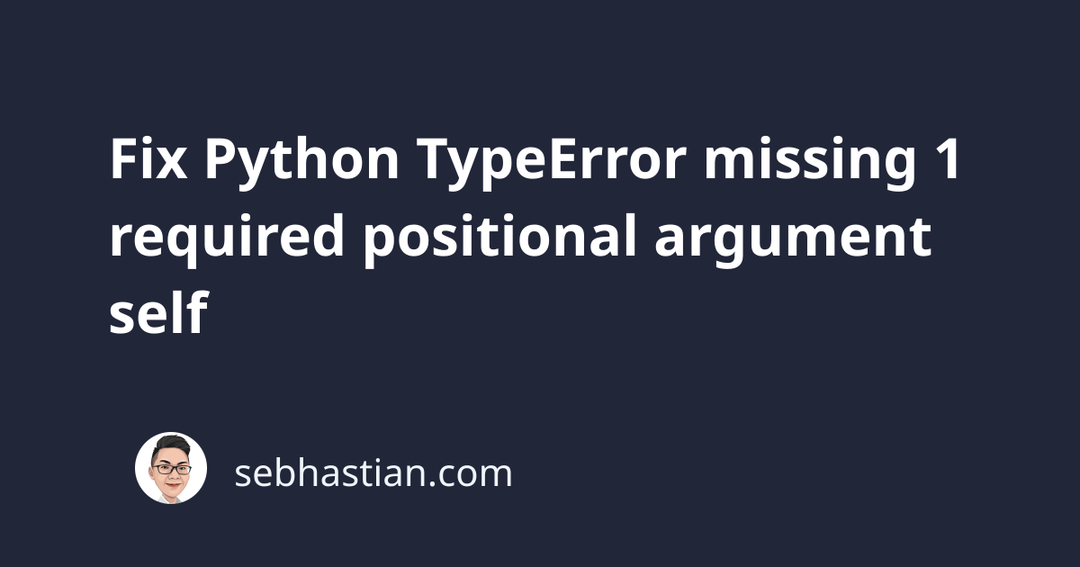
One error that you might encounter when working with Python functions is:
TypeError: missing 1 required positional argument: 'self'
This error usually occurs because you try to call a class method without instantiating an object first.
Let’s see an example of how to reproduce this error and the way to fix it.
Reproducing the error
Suppose you have a class named Car
as follows:
class Car:
def __init__(self, name):
self.name = name
def status(self):
print(f"Car name: {self.name}")
Then, you call the status()
method as follows:
Car.status()
Because you didn’t instantiate an object of the Car
class, the self
variable is not passed to the status()
method.
This causes the following error:
Traceback (most recent call last):
File "main.py", line 8, in <module>
Car.status()
^^^^^^^^^^^^
TypeError: Car.status() missing 1 required positional argument: 'self'
The second syntax form that can cause this error is as follows:
my_car = Car
my_car.status()
The variable my_car
stores a reference to the Car
class and not the Car
object, so it causes the error.
How to fix the error
To fix missing 1 required positional argument: 'self'
, you need to instantiate an object from the class first.
This is done by adding parentheses next to the class name. See the example below:
Car('Toyota').status() # ✅
You can also store the object in a variable as follows:
my_car = Car('Toyota') # ✅
my_car.status()
The self
variable will be passed when you call the status()
method from the instantiated object.
By the way, this is a special form of error that happens because you have missing required positional arguments.
I’ve written another post that explains required positional arguments error in more detail and how to fix it. You can read it here.
And that’s how you solve the error missing 1 required positional argument: 'self'
. Simple and easy! 👍