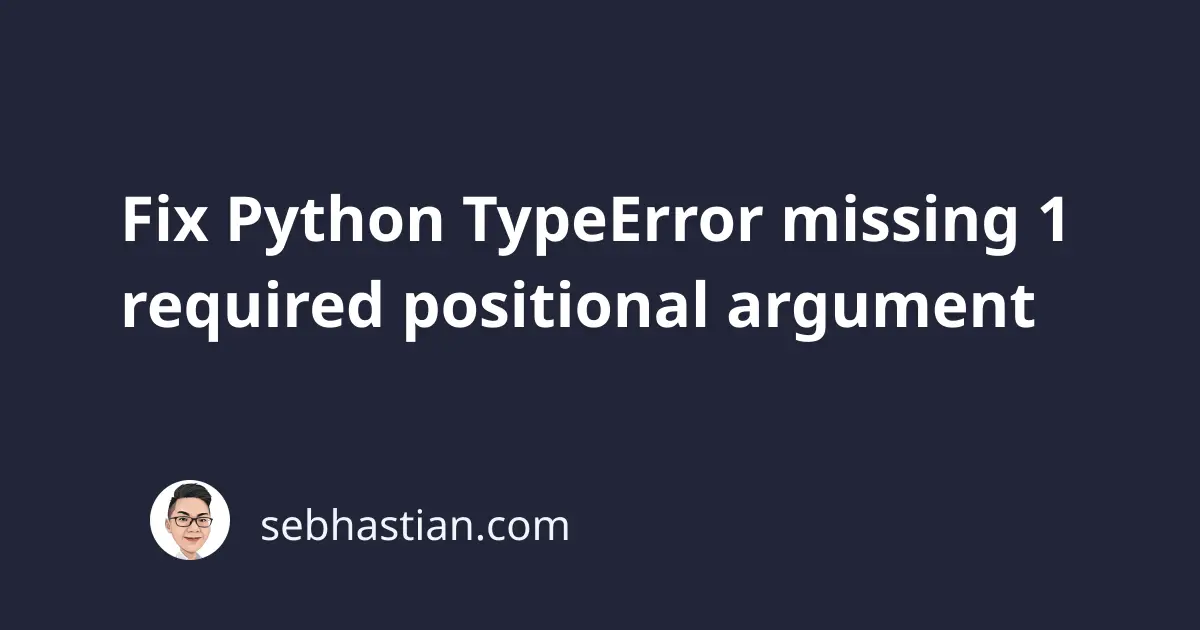
The TypeError missing 1 required positional argument
occurs in Python because of two possible causes:
This article explains why this error occurs and how you can fix it.
1. You called a function without passing the required arguments
A function in Python can have as many parameters as you define in the function definition.
For example, the following function greet()
has one parameter named first_name
:
def greet(first_name):
print(f"Hello, {first_name}")
When you call this function later, you need to provide a value that will be assigned as the first_name
variable. A value that you passed to a parameter is called an argument.
The error happens when you call a function that has a parameter without passing an argument for that parameter.
Let’s say you call greet()
as follows:
greet()
Because there’s no parameter, Python shows the following error:
Traceback (most recent call last):
File "main.py", line 6, in <module>
greet()
TypeError: greet() missing 1 required positional argument: 'first_name'
To fix this error, you need to provide the required argument:
greet("Nathan") # ✅
# Output: Hello, Nathan
If your function has many parameters, then Python will show missing x
required positional arguments error, with x
being the number of arguments you need to specify.
Here’s another example:
def greet(first_name, last_name):
print(f"Hello, {first_name} {last_name}")
greet() # ❌
Output:
Traceback (most recent call last):
File "main.py", line 5, in <module>
greet()
TypeError: greet() missing 2 required positional arguments: 'first_name' and 'last_name'
Again, you need to pass the required positional arguments when calling the function to fix this error.
Alternatively, you can also make the parameters optional by providing default values.
See the example below:
def greet(first_name="Nathan"):
print(f"Hello, {first_name}")
greet() # ✅ Hello, Nathan
The first_name
parameter in the greet()
function has a default value of Nathan
.
The addition of this default value makes the first_name
parameter optional. The default value will be used when the function is called without providing an argument for the parameter.
2. You instantiate an object without passing the required arguments
This error also occurs when you instantiate an object from a class without passing the arguments required by the __init__
method.
The __init__
method of a class is called every time you instantiate an object from a class.
Imagine you have a class named Car
with an __init__
method that sets the car price
as follows:
class Car:
def __init__(self, price):
self.price = price
Next, you try to instantiate an object without passing the argument for price
:
my_car = Car()
You’ll get the following error:
Traceback (most recent call last):
File "main.py", line 5, in <module>
my_car = Car()
^^^^^
TypeError: Car.__init__() missing 1 required positional argument: 'price'
To fix this error, you need to pass the required argument price
when instantiating a Car
object:
my_car = Car(20000)
print(my_car.price) # 20000
You can also provide a default value for the price
parameter to avoid the error:
class Car:
def __init__(self, price=30000):
self.price = price
my_car = Car()
print(my_car.price) # 30000
If the parameter has a default value, then it becomes optional. The default value will be used when the object is instantiated without passing the required argument(s).
To conclude, Python TypeError missing x required positional argument(s) is triggered when you don’t pass the required arguments when calling a function.
The error also appears when you instantiate an object from a class without passing the parameters required by the __init__
method.
To fix the error, you need to pass the x
number of required arguments.
I hope this article is helpful. I’ll see you in other articles! 👋