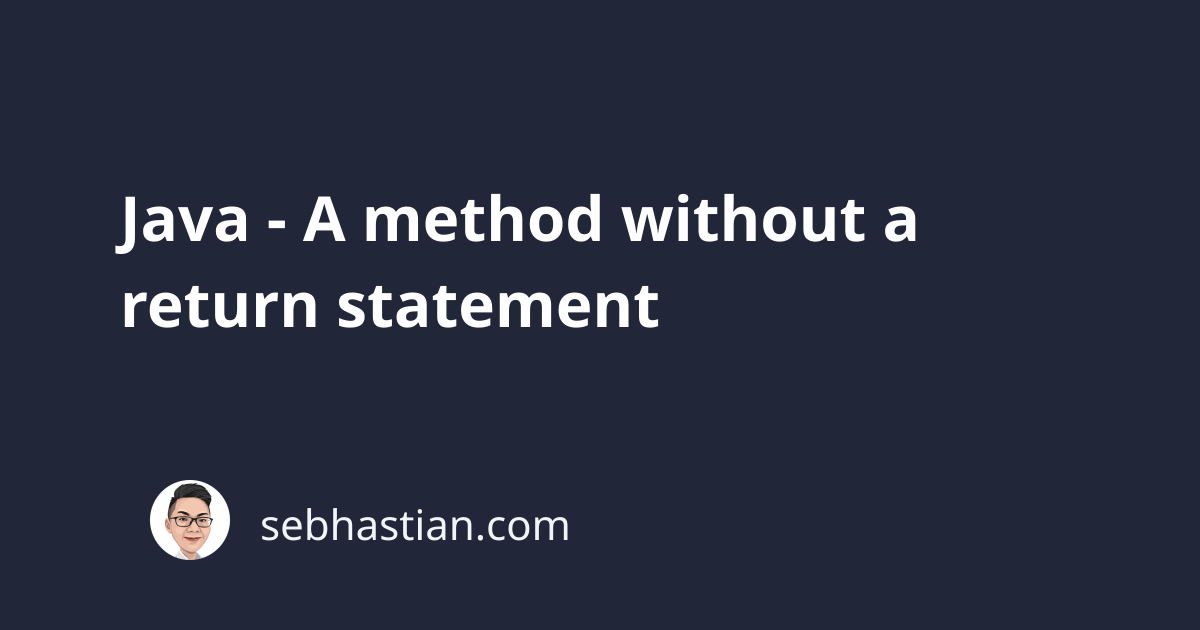
When learning Java, you probably encounter a quiz that asks what will happen when a method has no return
statement.
The quiz would go as follows:
If a method does not have a return
statement, then:
- a. it will produce a syntax error when compiled
- b. it must be a
void
method - c. it cannot be called from outside the class that defined the method
- d. it must be defined to be a
public
method - e. it must be an
int
,double
,float
, orString
method
The answer to the question above is b.
This is because a Java method with a void
return type returns nothing.
For example, the following hello()
method prints a message to the console without returning anything:
void hello() {
System.out.println("Hello World!");
}
When you change the return type to one of the valid Java types, an error will be thrown without a return
statement:
int hello() {
System.out.println("Hello World!");
} // ERROR: Missing return statement
It doesn’t matter if the method is a public
, protected
, private
, or default access level.
A method with a void
return type will work fine when you omit the return
statement.
The right answer to the quiz is b.