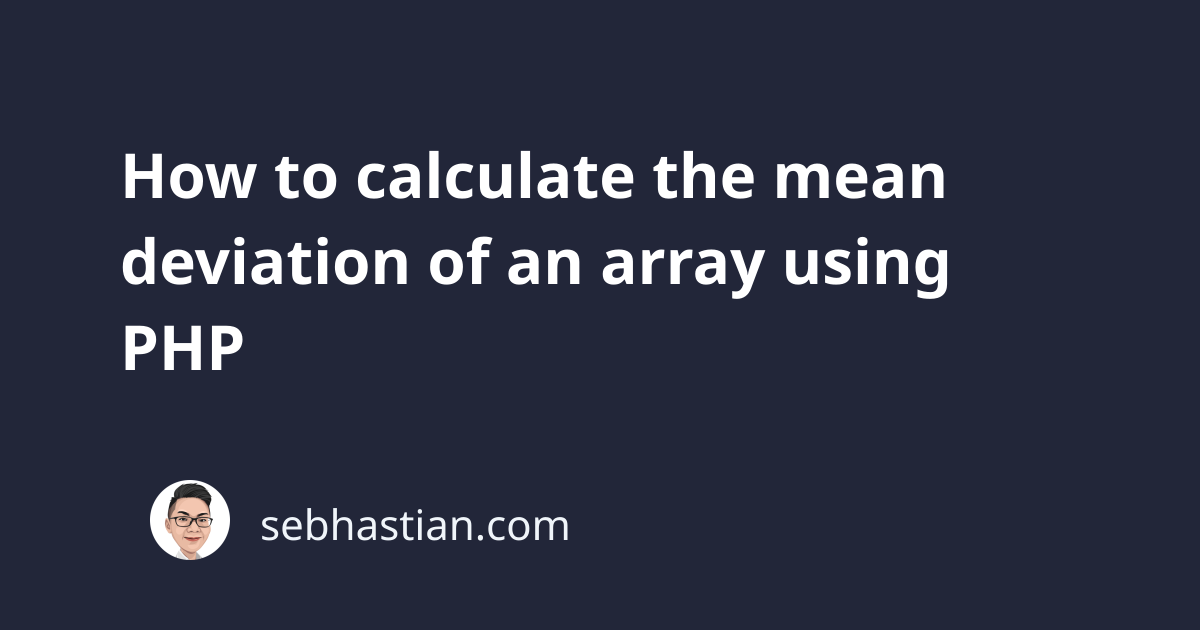
The mean deviation is a statistical measure used to calculate the average distance from the mean of a given data set.
The formula for mean deviation is as follows:
Mean Deviation = Σ|x - μ|N
Here’s what the symbols mean:
- Σ is Sigma, so you need to sum the numbers
- The vertical bars || mean absolute value, so you remove minus signs within the bars
- x is the value from your data set
- μ is the mean value
- N is how many values (the count)
To achieve the formula, you need to first calculate the mean value of your data set.
Next, you need to calculate the distance from the values (x) to the mean (μ) while ignoring the minus signs.
Once you get all the distances, sum them up (Σ) and divide them by the count (N).
Let’s see how these steps are translated to PHP code next.
PHP functions to find the mean deviation
To calculate the mean deviation of an array of numbers in PHP, you need to use several provided functions:
count()
to count the number of elements in the arrayarray_sum()
to calculate the sum of your valuesabs()
to calculate the distance from the values to the mean later
This tutorial will show you how to calculate the mean deviation value from an array of numbers.
Example: find the mean deviation from the following numbers
Suppose you are tasked with finding the mean deviation of the following numbers: 2, 4, 8, 10, 12, 6.
First, you need to count how many numbers are present using the count()
function.
Then, you need to calculate the mean value by adding the numbers and dividing it by the count:
<?php
$numbers = [2, 4, 8, 10, 12, 6];
// 👇 how many numbers are present
$count = count($numbers);
// 👇 calculate the mean
$mean = array_sum($numbers) / $count;
Now that you have the mean value, you can calculate the distance of each value from the mean.
To do so, you need to loop over the array using a foreach
construct, then add the numbers into a variable.
Use the abs()
function to turn negative numbers into positive ones:
// 👇 calculate distance of each value from the mean
$distance_sum = 0;
foreach ($numbers as $value) {
$distance_sum += abs($value - $mean);
}
// 👇 divide $distance_sum by $count
$mean_deviation = $distance_sum / $count;
print $mean_deviation;
Once you have the sum of distance, divide it by the $count
number. Now you have the mean deviation value of your numbers!
Here’s the complete code for calculating mean deviation with PHP:
$numbers = [2, 4, 8, 10, 12, 6];
// 👇 how many numbers are present
$count = count($numbers);
// 👇 calculate the mean
$mean = array_sum($numbers) / $count;
// 👇 calculate distance of each value from the mean
$distance_sum = 0;
foreach ($numbers as $value) {
$distance_sum += abs($value - $mean);
}
// 👇 divide $distance_sum by $count
$mean_deviation = $distance_sum / $count;
print $mean_deviation; // 3
The numbers 2, 4, 8, 10, 12, 6 have a mean value of 7 and a mean deviation value of 3.
You can put the code above in a function so you can reuse it whenever you need to find the mean deviation of an array:
function mean_deviation(array $numbers) {
$count = count($numbers);
$mean = array_sum($numbers) / $count;
$distance_sum = 0;
foreach ($numbers as $value) {
$distance_sum += abs($value - $mean);
}
$mean_deviation = $distance_sum / $count;
return $mean_deviation;
}
$numbers = [2, 4, 8, 10, 12, 6];
// 👇 call the function
print mean_deviation($numbers); // 3
Now you’ve learned how to calculate the mean deviation of a set of numbers using PHP.
Feel free to use and modify the code above for your project. 😉