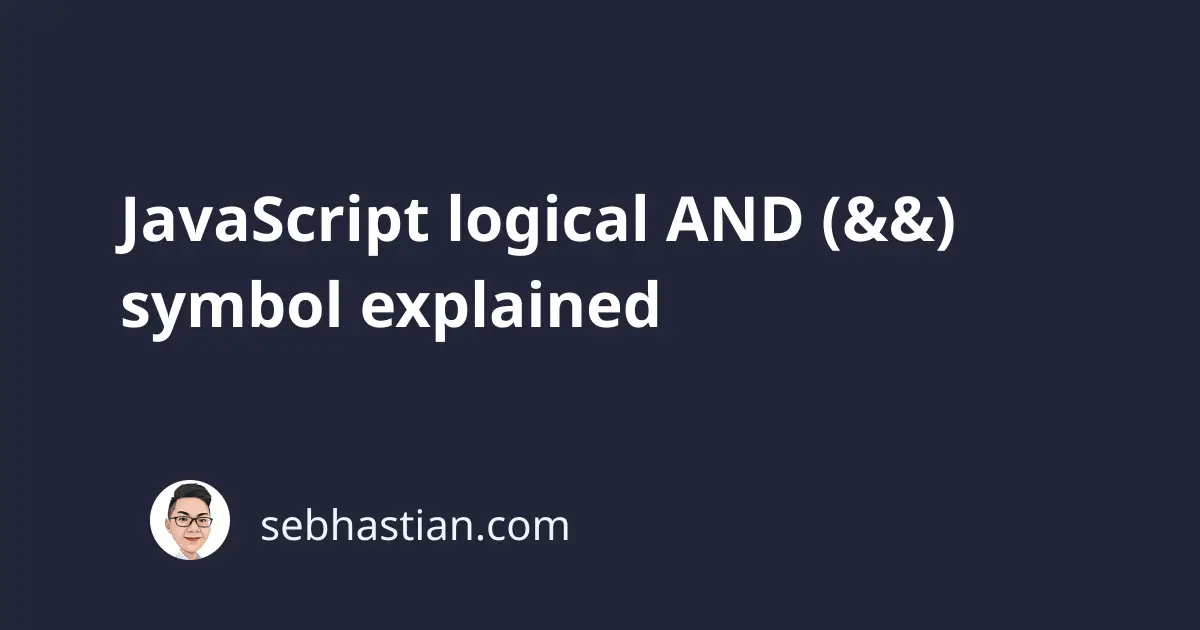
JavaScript logical AND operator is marked with two ampersand symbols (&&
).
The operator is used to perform logical conjunction of the values you added as its operands.
The logical AND operator returns true
only when all operand values evaluate as true
.
Consider the following example code:
const resultOne = 3 > 0 && 2 > 0;
console.log(resultOne); // true
const resultTwo = 1 < 0 && 10 > 2;
console.log(resultTwo); // false
The logical AND operator evaluates the values placed as its operand from left to right.
It immediately returns the first falsy
value it encounters, or truthy
when all values evaluate to true
.
In the above example, The numbers 3
and 2
are both greater than 0
, so the expression 3 > 0
and 2 > 0
evaluates to true
.
But in the second example, the number 1
is not less than 0
, so 1 < 0
evaluates to false
. The AND operator stops any further evaluation and returns the value false
to you.
The AND operator works with any kind of expression, meaning you can use a single value as shown below:
true && false; // false
true && true; // true
1 && 0; // 0
1 && null; // null
1 && undefined; // undefined
You can also use the AND operator as many times as you need:
true && true && true && true; // true
true && true && true && false; // false
The AND operator will return any truthy value returned by the last operand.
If you put a string
or number
as the value of the last operand, it will be returned as shown below:
true && "A" && true; // true
true && true && "A"; // "A"
true && true && 2000; // 2000
// falsy value will be returned first
true && false && "A"; // false
true && 0 && 2000; // 0
And that’s how the logical AND (&&
) operator works in JavaScript. 😉