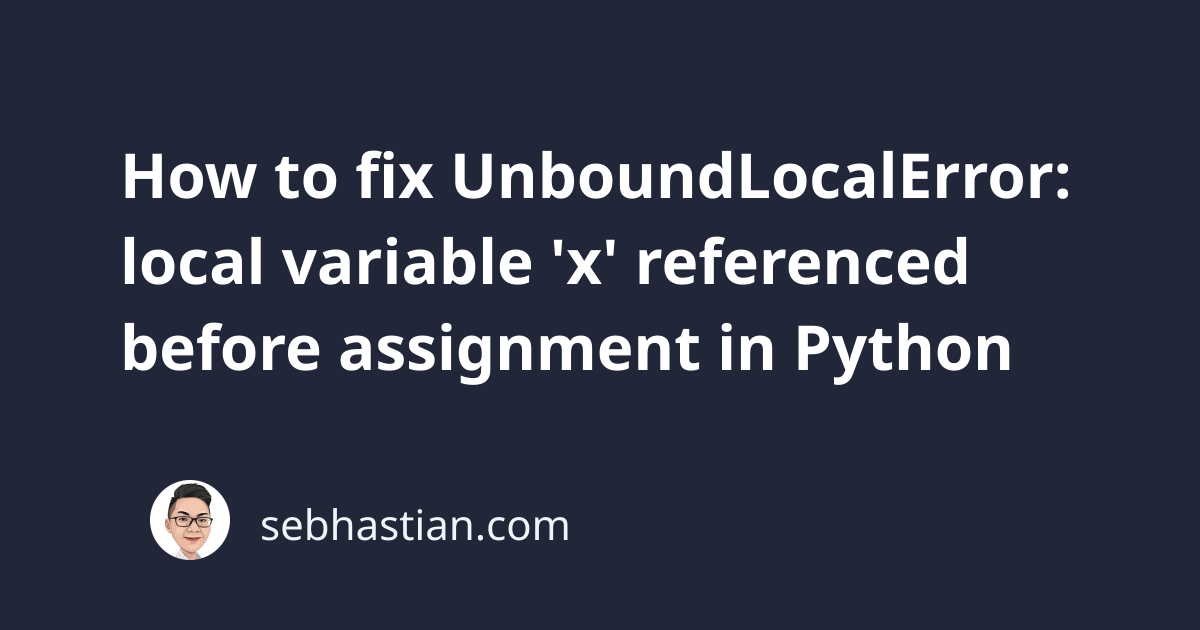
One error you might encounter when running Python code is:
UnboundLocalError: local variable 'x' referenced before assignment
This error commonly occurs when you reference a variable inside a function without first assigning it a value.
You could also see this error when you forget to pass the variable as an argument to your function.
Let me show you an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you have a variable called name
declared in your Python code as follows:
name = "Nathan"
Next, you created a function that uses the name
variable as shown below:
name = "Nathan"
def greet():
name = "Mr. " + name
print("Hello, " + name)
greet()
When you execute the code above, you’ll get this error:
File "main.py", line 4, in greet
name = "Mr. " + name
UnboundLocalError: local variable 'name' referenced before assignment
This error occurs because you both assign and reference a variable called name
inside the function.
Python thinks you’re trying to assign the local variable name
to name
, which is not the case here because the original name
variable we declared is a global variable.
How to fix this error
To resolve this error, you can change the variable’s name inside the function to something else. For example, name_with_title
should work:
def greet():
name_with_title = "Mr. " + name
print("Hello, " + name_with_title)
As an alternative, you can specify a name
parameter in the greet()
function to indicate that you require a variable to be passed to the function.
When calling the function, you need to pass a variable as follows:
name = "Nathan"
def greet(name):
name = "Mr. " + name
print("Hello, " + name)
greet(name)
This code allows Python to know that you intend to use the name
variable which is passed as an argument to the function as part of the newly declared name
variable.
Still, I would say that you need to use a different name when declaring a variable inside the function. Using the same name might confuse you in the future.
Here’s the best solution to the error:
name = "Nathan"
def greet(name):
name_with_title = "Mr. " + name
print("Hello, " + name_with_title)
greet(name)
Now it’s clear that we’re using the name
variable given to the function as part of the value assigned to name_with_title
. Way to go!
Conclusion
The UnboundLocalError: local variable 'x' referenced before assignment
occurs when you reference a variable inside a function before declaring that variable.
To resolve this error, you need to use a different variable name when referencing the existing variable, or you can also specify a parameter for the function.
I hope this tutorial is useful. See you in other tutorials.