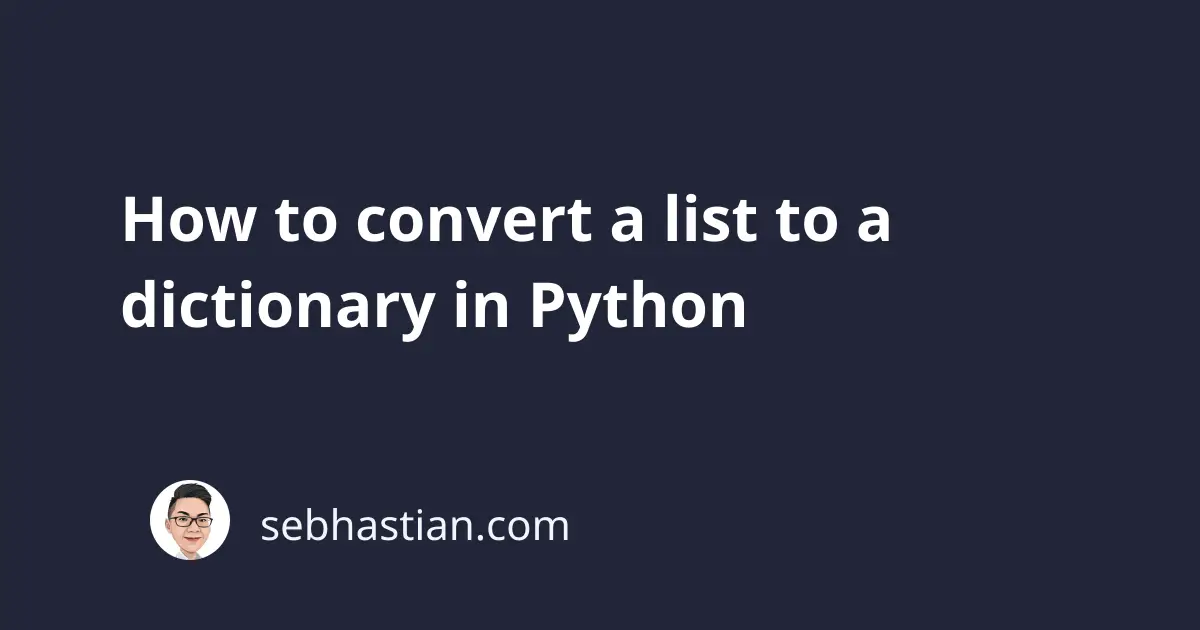
To convert a list to a dictionary, you can use the dict.fromkeys()
method, the zip()
function, or a dictionary comprehension syntax.
In Python, a list is a collection that allows you to store data in a specific order. Here’s an example:
person = ["Jane", 29, "German"]
A dictionary is like a list, but with the added ability to define your own index keys:
person = {
"name": "Jane",
"age": 29,
"nationality": "German"
}
In dictionaries, the index keys can act as labels for the value it stores, giving you more context about the data.
Depending on the end result you want to achieve, there are three ways you can convert a list to a dictionary:
1. Use the dict.fromkeys()
method
The dict.fromkeys()
method creates a new dictionary from the arguments you send to the method.
This method accepts two arguments:
- An iterable you want to convert as keys
- The value you want to set for each key
The weakness of this method is that you can only set the same value for all the keys.
Here’s an example of using this method:
person = ["Jane", 29, "German"]
dic = dict.fromkeys(person, 'a')
print(dic)
Output:
{'Jane': 'a', 29: 'a', 'German': 'a'}
The list values become the keys of the dictionary, and you can’t set different values for each key.
If you don’t define the value, then Python would use None
as the default value.
2. Using the zip()
function
Suppose you have two different lists where one stores the values and the other stores the keys:
keys = ["name", "age", "nationality"]
values = ["Jane", 29, "German"]
You can combine these two lists as one dictionary using the zip()
function.
The zip()
function accepts multiple list objects and returns a single zip object that paired the first item in each list together.
You can convert the zip object to a dictionary using the dict()
function. Let’s see an example:
keys = ["name", "age", "nationality"]
values = ["Jane", 29, "German"]
dic = dict(zip(keys, values))
print(dic)
print(dic["name"])
print(dic["age"])
print(dic["nationality"])
Output:
{'name': 'Jane', 'age': 29, 'nationality': 'German'}
Jane
29
German
As you can see, the zip()
function succeeded in pairing the items in keys
and values
lists as a dictionary key-value pair.
3. Using a dictionary comprehension syntax
If you have one list that contains keys and values, then you can use a dictionary comprehension syntax to separate the data as key-value pairs.
Suppose you have a list like this:
person = ["name", "Jane", "age", 29, "nationality", "German"]
Then you can convert the list to a dictionary with the code below:
person = ["name", "Jane", "age", 29, "nationality", "German"]
dic = {
person[idx]: person[idx+1] for idx in range(0, len(person), 2)
}
print(dic)
Output:
{'name': 'Jane', 'age': 29, 'nationality': 'German'}
The dictionary comprehension loops through the person
list and creates a key-value pair for the dictionary.
Inside the comprehension, the range()
function is used to generate a sequence of indices that correspond to the keys in the dictionary.
The range() function starts at 0
and goes up to the length of the person list in steps of 2
(i.e. 0, 2, 4, …).
This ensures that the idx
values correspond to the keys in the person list.
Finally, the key-value pairs are created using the person[idx]: person[idx+1]
code, where person[idx]
becomes the key and person[idx+1]
becomes the value.
Conclusion
The three solutions provided in this tutorial show you how to convert a list to a dictionary in Python.
The solution you use depends on the structure of the list you’re given.
If you have two different lists, then using the zip()
function might be a great idea.
If you have a single list that contains keys and values, using a dictionary comprehension syntax is a wise choice.