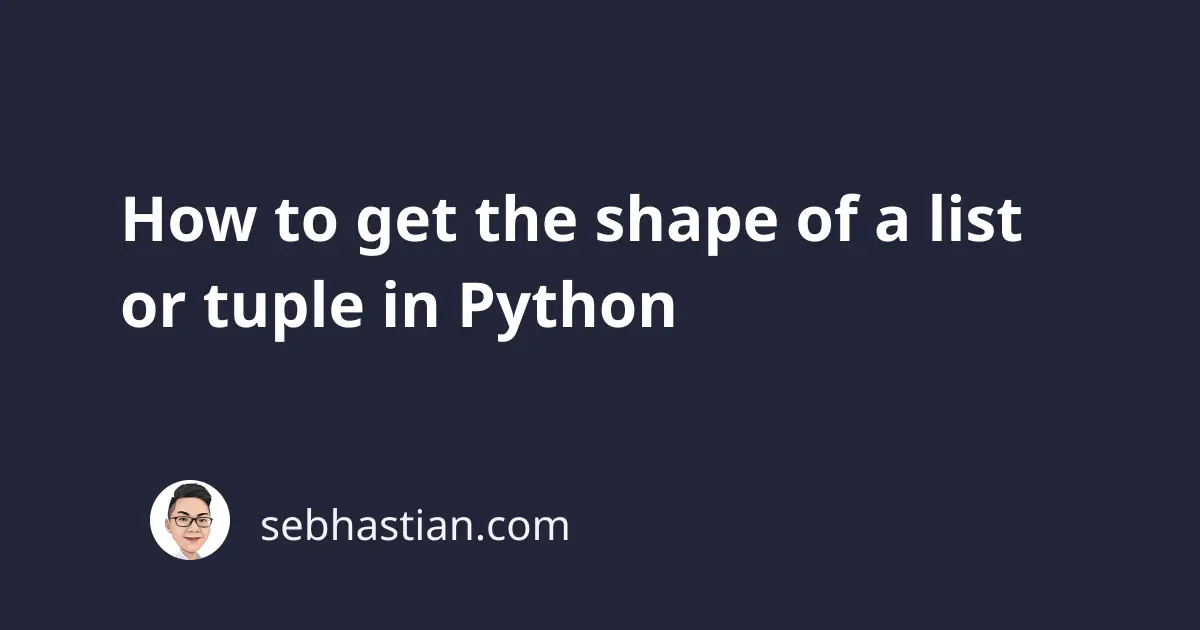
In Python, lists and tuples are collection objects that can contain multiple values.
Sometimes, you need to get the number of elements contained in these objects. When using the NumPy library, you can find the shape of an array using the .shape
property:
import numpy as np
my_array = np.array([[1,2,3], [1,2,3]])
print(my_array.shape)
Output:
(2, 3)
The shape
property returns a tuple showing how many rows and columns your array has. In this case, we have 2 rows and 3 columns for each row.
But this property doesn’t exist in a list or tuple object. To get the shape of these objects, you need to use the len()
function.
The len()
function returns the number of items stored in a tuple or a list as shown below:
my_list = [1, 2, 3]
print(len(my_list))
Output:
3
This is sufficient when you have a one-dimensional list or tuple, but not enough when you have a multi-dimensional list.
Unlike the NumPy array, a list or tuple can have any number of elements in a row. You can have 3 items in the first row, then 2 items in the next.
For example, suppose you have a 2 dimensional list as follows:
my_list = [[1, 2, 3], [4], [5, 6]]
To count how many rows you have in the list, you can use the len()
function and pass the list:
print(len(my_list))
# Output: 3
But to get how many items in each row, you need to use a list comprehension (or tuple comprehension) and call len()
for each item in the list.
Here’s an example of finding the rows and columns for my_list
:
my_list = [[1, 2, 3], [4], [5, 6]]
rows = len(my_list)
columns = [len(item) for item in my_list]
# Combine the rows and columns in one list
shape = [rows, columns]
print(shape)
Output:
[3, [3, 1, 2]]
As you can see, now the shape
variable contains the number of rows in my_list
, followed by a list containing the number of columns for each row.
If you know that each nested list will have an equal number of items, then you can use len()
to count the number of items in the first list as shown below:
my_list = [[1, 2, 3], [5, 6, 7]]
rows = len(my_list)
columns = len(my_list[0])
# Combine the rows and columns as a tuple
shape = (rows, columns)
print(shape)
Output:
(2, 3)
This way, you’ll get a tuple containing two values, just like the shape
property in a NumPy array.
Conclusion
The Python list and tuple objects are different from the NumPy array object. When you need to get the shape of a list or a tuple, you need to use the len()
function to get the rows and columns defined in the object.
A NumPy array must have the same number of values in each row or it will raise an error, but a list or a tuple can have any number of values, so getting the shape of a list or tuple is a bit tricky.
You can use the example in this tutorial to help you get the shape of a list or a tuple. Happy coding! 🙌