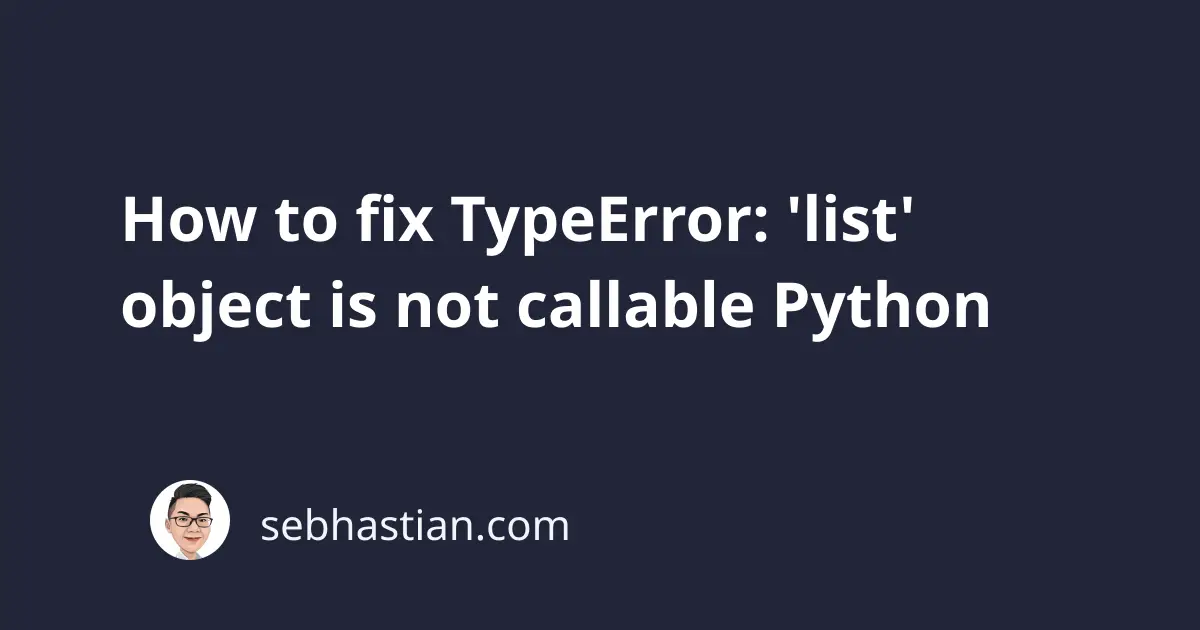
When running Python code, you might encounter the following error:
TypeError: 'list' object is not callable
This error occurs when you attempt to access an item in a list using parentheses ()
or round brackets. This causes Python to think you’re trying to call a function instead of accessing a list item.
After doing some research, I found two common causes for this error:
- You’re using parentheses to access a list item
- You replaced the
list()
function with a real list
In this tutorial, I will show you how to fix this error in both scenarios.
1. You’re using parentheses to access a list item
Suppose you create a list in Python as follows:
my_list = [1, 2, 3]
Next, you try to print the first element in the list by accessing the list as follows:
my_list = [1, 2, 3]
print(my_list(0))
When running the code, you’ll get the following error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(my_list(0))
^^^^^^^^^^
TypeError: 'list' object is not callable
The error message tells you everything you need to know already. The TypeError
exception occurs when you run an operation or function to an object that doesn’t support the operation.
In this case, the list object doesn’t support a call operation using round brackets ()
because it’s not callable. Only function-like objects are callable in Python.
To resolve this error, you need to edit your code and use square brackets when accessing items from a list.
This example shows the right way to access a list item:
my_list = [1, 2, 3]
print(my_list[0])
print(my_list[1])
print(my_list[2])
Output:
1
2
3
Notice that the print()
function retrieved the list items successfully, and you didn’t receive an error this time.
2. You replaced the list()
function with a real list
Another common cause of this error is that you assigned a list under a list
variable.
For example, suppose you create two list objects where the second list is created from a tuple that you convert to a list:
list = [1, 2, 3]
second_list = list((5, 6, 7))
The name list
is reserved by Python for the built-in list()
function, which allows you to convert an object to a list.
By assigning a list object to the same name, you unintentionally replaced the function with a list.
To prevent this error, you need to use another name for the first list:
first_list = [1, 2, 3]
second_list = list((5, 6, 7))
The error is now resolved, and you can convert the tuple to a list successfully.
Conclusion
Python shows the TypeError: 'list' object is not callable
when you attempt to call a list object using round brackets ()
as if it were a function.
To resolve this error, make sure you’re accessing a list using square brackets []
and you don’t use list
as the variable name of an actual list.
I hope this tutorial helps. See you in other tutorials! 👋