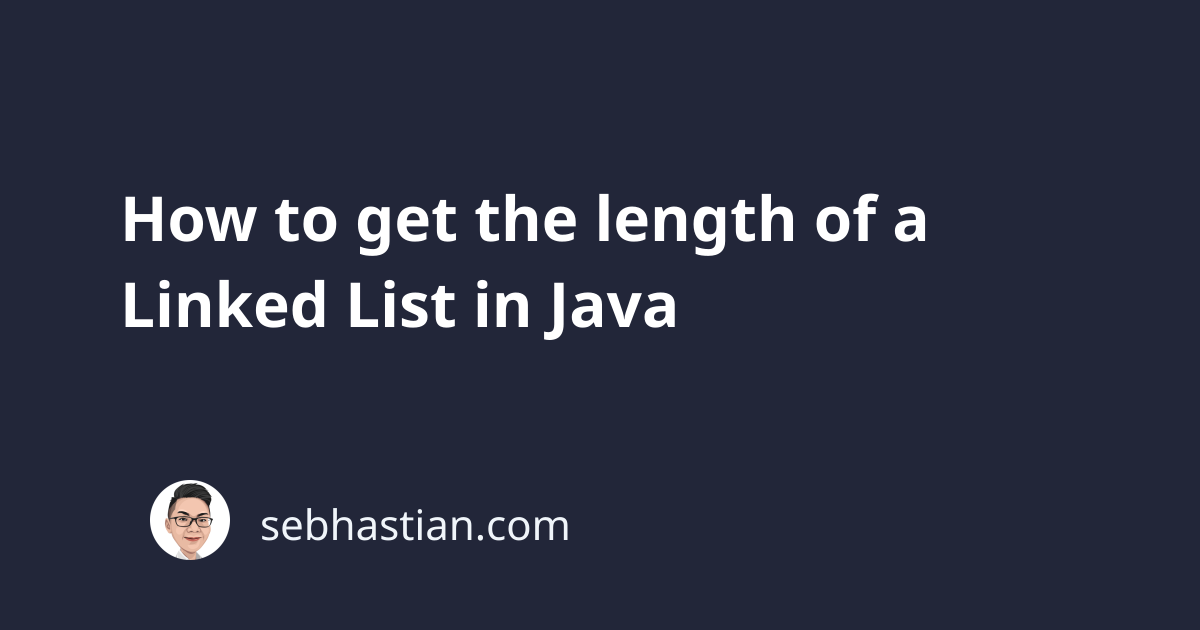
The Java LinkedList
class comes with the size()
method that you can use to get the length of its instance.
For example, suppose you create a Linked List instance named myList
as follows:
LinkedList<String> myList = new LinkedList();
myList.add("January");
myList.add("February");
myList.add("March");
int length = myList.size();
System.out.println(length); // 3
The .size()
method can be called from any LinkedList
instance, and it will return the current length of the instance.
When you add or remove an element from the instance, the size()
method will adjust the return value to match the new length:
LinkedList<Integer> myList = new LinkedList();
myList.add(101);
myList.add(102);
myList.add(103);
System.out.println(myList.size()); // 3
myList.add(104);
myList.add(105);
System.out.println(myList.size()); // 5
And that’s how you get the length
of a Linked List instance in Java. 😉