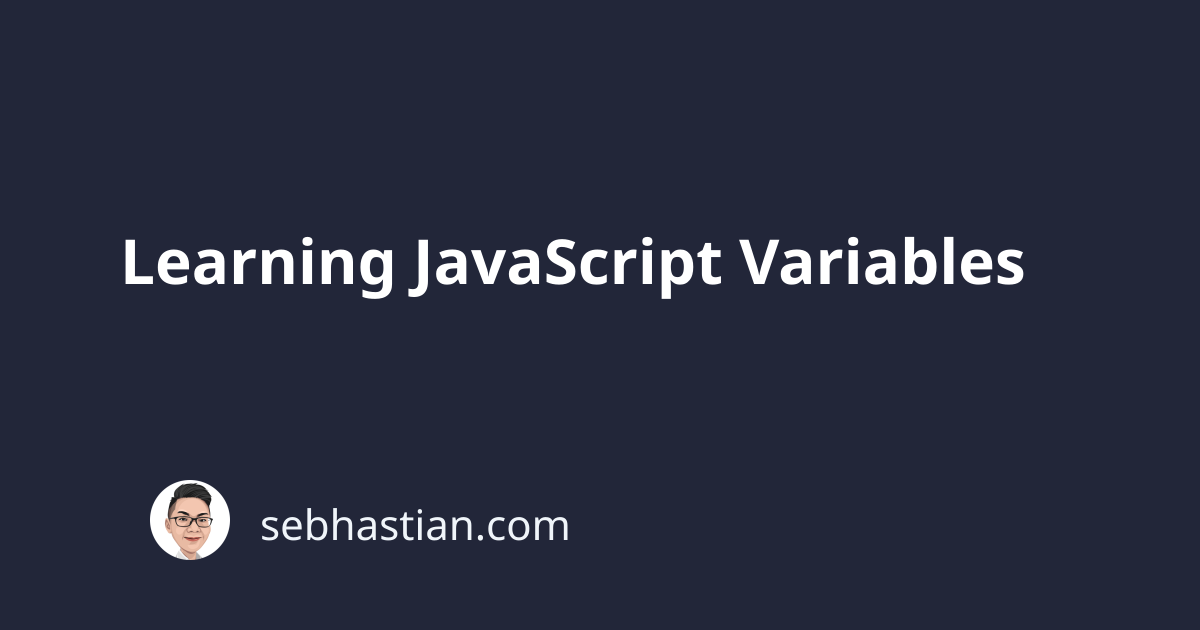
Remember the part where I explained literals and identifiers? Well a variable is an identifier with literal value assigned to it.
It’s like a virtual box that can hold values you put into it. We’ll learn them by examples in this section.
A variable is a tool to retain certain values that will be used in a program in the future.
The purpose of variable is to keep us, programmers, from repeating our code over and over.
Variables in JavaScript are untyped — it means you can assign a value of any type to a variable, and then you can assign another value with a different type into the same variable.
Variables in JavaScript must be declared with variables keyword before we can use it.
There are 3 keywords you can use to create variables: let
, var
and const
.
Note: The following section contains sample code that you can run on your JavaScript development environment. If you are new to JavaScript and would like try and run the code samples, follow the guide on JavaScript Development Environment Guide
Using let
keyword
let
keyword indicates that the statement will create a binding between variable name and value:
let name = "Nathan"; // bind variable name "name" to string value "Nathan"
console.log(name);
// Nathan
name = "Sebhastian"; // can you guess this one?
console.log(name);
// Sebhastian
As you can see, the name variable I created was first assigned the value “Nathan”, and then changed to “Sebhastian”. You can also declare multiple variables using the same var
keyword:
let firstName = "Nathan", // don't forget this comma
lastName = "Sebhastian";
console.log(firstName);
console.log(lastName);
If you don’t specify any value during variable declaration, the value of the variable will be undefined until your code store a new value into it.
let age;
console.log(age);
// undefined
age = 25;
console.log(age);
// 25
That’s pretty much explain let
keyword.
The var
keyword
var
keyword is used declare variable with a global scope. This keyword is the only keyword we can use to declare variable before ES2015 was released.
As of today, JavaScript programmers tend to avoid using var
if they could, since var
can introduce bugs that can be avoided using let
keyword.
You’ll learn about it more in JavaScript functions tutorial.
var firstName = "Nathan", // you will hardly notice any difference in the result.
lastName = "Sebhastian";
console.log(firstName);
console.log(lastName);
The bottom line you need to remember is this: let
is new and recommended way to declare variable since ES2015.
The const
keyword
Variables declared using const
keyword CANNOT be changed after its declaration.
This variable is used for special situations where the value of the variable need to remain the same throughout runtime.
const name = "Nathan";
name = "Jeremy"; // will throw TypeError: Assignment to constant variable.
That will be everything you need to know about variables for now. Let’s move on to data types.