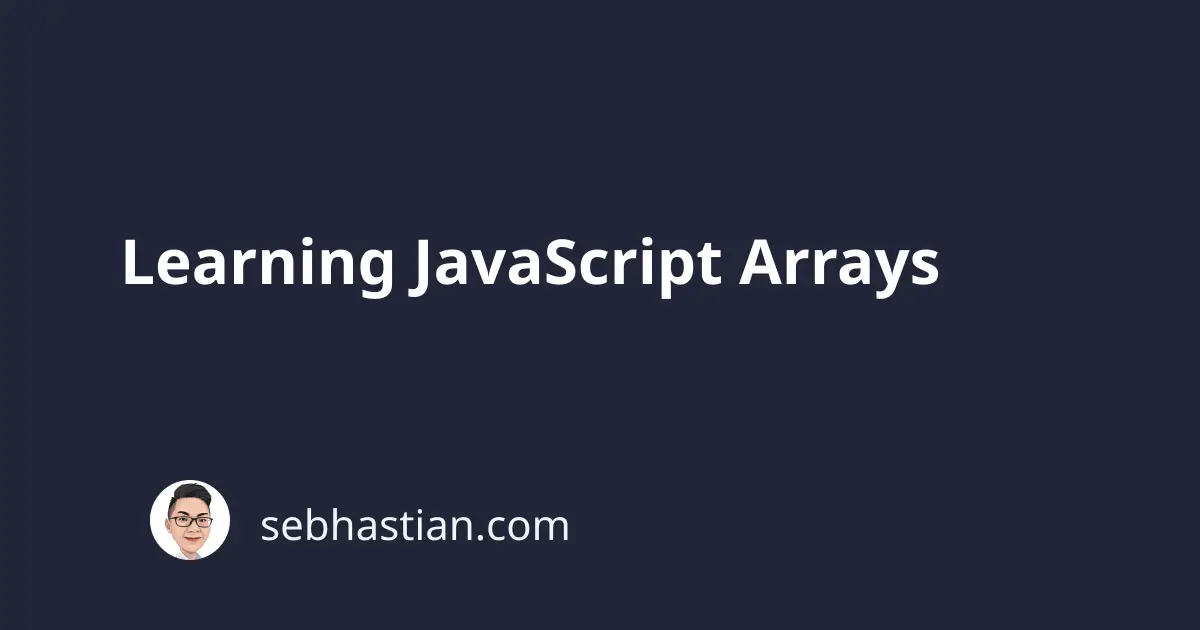
Many times when you’re writing an application, you will encounter a list of data that need to be used. For example: a list of items inside a shopping cart or a list of users email.
To deal with list, we can use an array. In JavaScript, array is a data structure that is used to hold a group of data in one definition.
You can think of array as a set of empty boxes that can hold values in an orderly fashion.
An array
is written as a list of values between square brackets and separated by commas. To access an array value, you have to call it using the arrayName[index]
syntax
// example of an array
let listOfNumbers = [7, 8, 9, 0];
console.log(listOfNumbers[0]); // array index 0 is 7, so it will print out 7
console.log(listOfNumbers[3]); // print out 0
listOfNumbers[2] = 3; // replace 9 with 3
An array can contain any data type you can think of, from strings, numbers, objects, and even another array.
// put every imaginable thing here!
let dogObject = { name: "Russ" };
let listOfRandoms = [7, "cow", dogObject, [1, 2, 3], 9.5];
// try console.log() and see what is being printed out!
Array has many powerful data manipulation features that are used commonly for data problems. Here I will list some of the most common methods of dealing with array data.
Array length
Count how many data an array is holding at the moment in number.
let listOfNumbers = [7, 8, 9, 0];
console.log(listOfNumbers.length); // print out 4
Adding new data to an array
At the beginning of array
Use unshift
to add at the beginning of an array
let listOfNumbers = [7, 8, 9, 0];
listOfNumbers.unshift(3, 3);
console.log(listOfNumbers); // [3,3,7,8,9,0]
At the end of an array
Use push
and like unshift, you can add more than one value.
let listOfNumbers = [7, 8, 9, 0];
listOfNumbers.push(3);
console.log(listOfNumbers); // [3,3,7,8,9,0]
Removing an item from array
From the last index
Use the pop
method. No parameter accepted.
let listOfNumbers = [7, 8, 9, 0];
listOfNumbers.pop();
console.log(listOfNumbers); // [7,8,9]
From the beginning
let listOfNumbers = [7, 8, 9, 0];
listOfNumbers.shift();
console.log(listOfNumbers); // [8,9,0]
At a specific index
listOfNumbers.splice(1, 2); // from index 1, remove 2 items
Join multiple arrays
let a = [7, 8];
let b = [1, 2];
a.concat(b); // [7,8,1,2]
Search for a value
indexOf
will return the index of the value put in the parameter, while lastIndexOf
will give you the last index of the matching values.
let a = [7, 8, 9, 7];
a.indexOf(7); // return 0
a.lastIndexOf(7); // return 3
a.indexOf(0); // return -1 because no match was found. Same with lastIndexOf
Get a portion of an array
The slice
keyword can have 2 parameters. the starting
index and the end
index. End index is not sliced. Slice also returns a copy of the array, which means the original array won’t be affected.
let a = [7, 8, 9, 7];
a.slice(2); // 9,7
a.slice(1, 3); // 8,9 not including the last 7
I might update this list in the future, please do let me know if I miss anything useful.