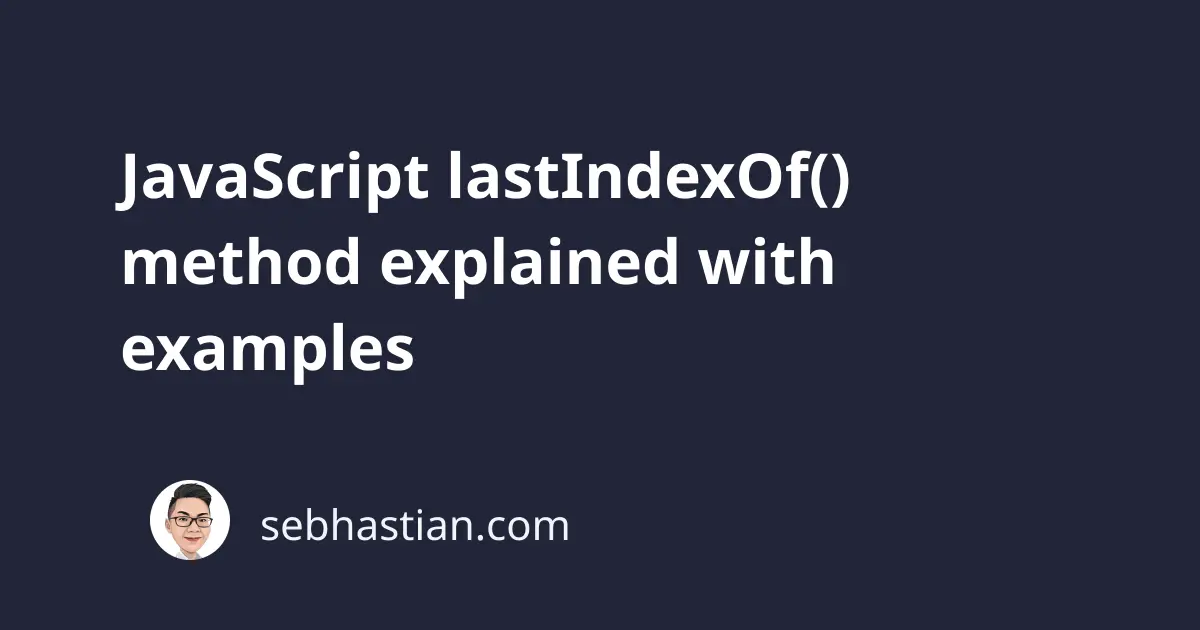
The JavaScript lastIndexOf()
method is a method of JavaScript String
and Array
prototype objects.
The function is used to return the index (position) of the last occurrence of the given argument.
The index of both JavaScript strings and arrays start from 0
, and here are some examples of using the lastIndexOf()
method:
// A string
let aString = "Good morning! It's a great morning to learn programming.";
let index = aString.lastIndexOf("morning");
console.log(index); // 27
// An array
let anArray = ["day", "night", "dawn", "day"];
let valueIndex = anArray.lastIndexOf("day");
console.log(valueIndex); // 3
As you can see from the examples above, the lastIndexOf()
method is available for string
and array
type values in JavaScript.
When the value you search for is not found in the string or array, the method will return -1
:
let aString = "Nathan Sebhastian";
let index = aString.lastIndexOf("morning");
console.log(index); // -1
let anArray = ["day", "night", "dawn", "day"];
let valueIndex = anArray.lastIndexOf("dusk");
console.log(valueIndex); // -1
The lastIndexOf()
method is also case sensitive, so you need to have the letter case in the argument match the actual string.
The above example returns -1
because Nathan
is different from nathan
:
let aString = "Nathan Sebhastian";
let index = aString.lastIndexOf("nathan");
console.log(index); // -1
The lastIndexOf()
method will search your string or array backward.
This means the method will look for matching values from the last element and ends with the first element.
Finally, the method also accepts a second parameter to define the start position of the search.
The following example shows how to start the lastIndexOf()
search at index 4
:
let aString = "Hello World!";
let index = aString.lastIndexOf("World", 4);
console.log(index); // -1
Because a second argument 4
is passed to the lastIndexOf()
method, JavaScript will search the value from index 4
to index 0
only.
While the World
string is available inside the aString
variable, the index starts at 6
. Since it’s outside the search range of the lastIndexOf()
method, the value is not found and -1
is returned.
The same rule applies when you search for a value in an array:
let anArray = [8, 3, 4, 8, 2];
let index = anArray.lastIndexOf(8, 2);
console.log(index); // 0
In the abov example, the lastIndexOf()
method returns 0
for the index of the value 8
.
This is because the second argument 2
makes the method search from index 2
only. Since the last index of the value(8
) is 3
, it stays outside of the method search range.
And that’s how the lastIndexOf()
method works in JavaScript. 😉