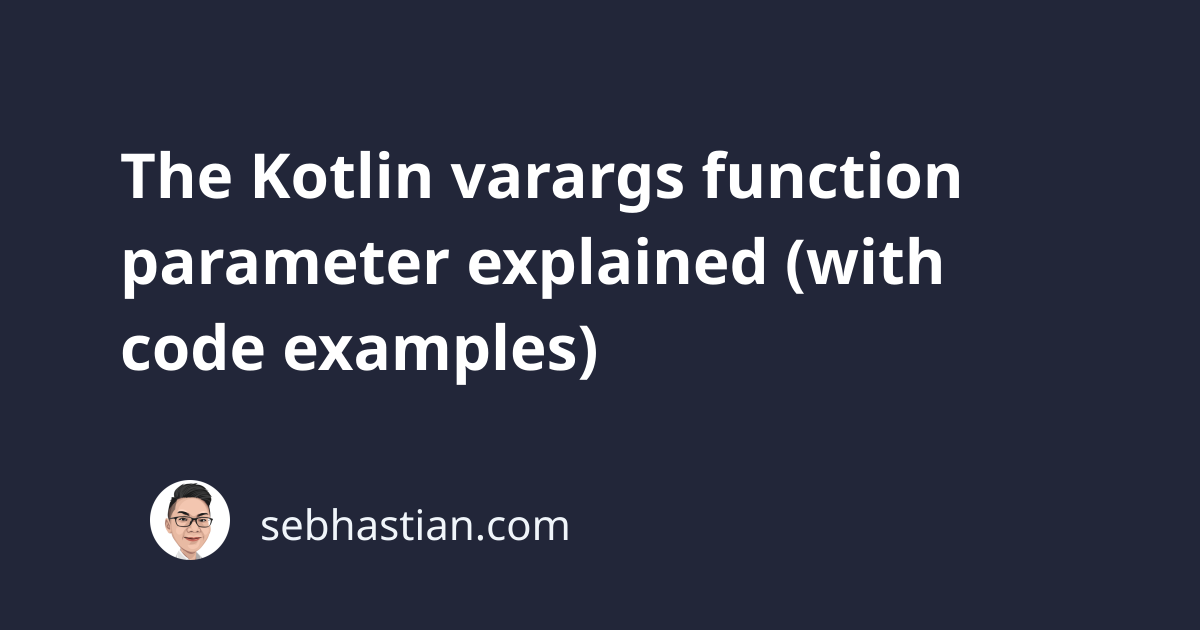
A variable number of arguments (also known as varargs) is a feature of the Kotlin language that allows you to pass a number of values as a single argument variable to a function.
When defining a function, you usually need to specify the name of each parameter that the function can receive inside the function parentheses.
The following example shows a simple Kotlin function that sums two numbers together:
fun sumNumbers(a: Int, b: Int): Int {
return a + b
}
sumNumbers(2, 3) // 5
Now when you want to sum more numbers, you need to add another parameter to the function definition.
The following example adds another parameter c
to the sumNumbers
function:
fun sumNumbers(a: Int, b: Int, c: Int): Int {
return a + b + c
}
sumNumbers(2, 3, 4)
This can be a problem because if we have more numbers to sum, then we need to add more parameters to the function itself.
One way of solving this problem might be to convert the numbers into an array.
That way, you can pass a single parameter of an IntArray
type to the function and call the sum()
function rather than passing them one by one.
Consider the following code example:
fun sumNumbers(numbers: IntArray): Int {
return numbers.sum()
}
val numArray = intArrayOf(1, 2, 3, 4)
sumNumbers(numArray) // 1+2+3+4 = 10
A varargs is similar to how an array works. It allows you to pass a single variable that contains multiple values.
But when using a vararg, you don’t need to create an array. You can pass your values separated by a comma, just like normal arguments.
To define a variable of arguments, you need to add the vararg
keyword before the parameter name as shown below:
fun sumNumbers(vararg numbers: Int): Int {
return numbers.sum()
}
sumNumbers(1, 2, 3, 4, 5) // 15
Notice how the vararg
keyword is added before the numbers
parameter and the values to sum are passed just like regular arguments during the sumNumbers()
call.
This is the power of varargs in Kotlin. It allows you to define a single variable as a function parameter that can accept more than one value.
A vararg
argument will be available inside the function as if it was an Array
type data.
In the example above, you can call the same sum()
function that’s available for IntArray
type.
Here’s another example of concatenating many String
values as one:
fun concatWords(vararg words: String): String {
return words.joinToString(separator = ".. ")
}
concatWords("Banana", "Apple") // Banana.. Apple
A vararg
argument is usually declared as the last parameter in the function definition, with other parameters preceding it.
In the example below, the name
and age
parameter will have the first and second position from the function call’s arguments:
fun concatWords(name: String, age: Int, vararg words: String) {
println("$name is $age years old")
println("Favorite words:")
println(words.joinToString(separator = "// "))
}
concatWords("Nathan", 28, "Love", "Loyalty")
/*
Output:
Nathan is 28 years old
Favorite words:
Love// Loyalty
*/
You can place the vararg
argument before the other parameters, but you will need to pass arguments for subsequent parameters using named arguments syntax.
Consider the following example. Note that the name
and age
arguments are passed after the words
argument in the function call:
fun concatWords(vararg words: String, name: String, age: Int) {
println("$name is $age years old")
println("Favorite words:")
println(words.joinToString(separator = "// "))
}
concatWords("Love", "Loyalty", name = "Nathan", age = 28)
If you do have an Array
type already and want to pass it into the vararg
argument, you can do so by using the spread operator *
before the Array
value.
The example below shows how to pass an IntArray
into a vararg
argument:
val numArray = intArrayOf(1, 2, 3, 4, 5)
fun sumNumbers(vararg numbers: Int): Int {
return numbers.sum()
}
sumNumbers(*numArray) // 15
The spread operator *
pulls the array values out so that the values are passed like multiple arguments.
Finally, a Kotlin function can only have one vararg
parameter.
You can’t add two or more vararg parameters because the Kotlin compiler will throw an error during compilation time.
Nice work on learning about Kotlin varargs! 😉