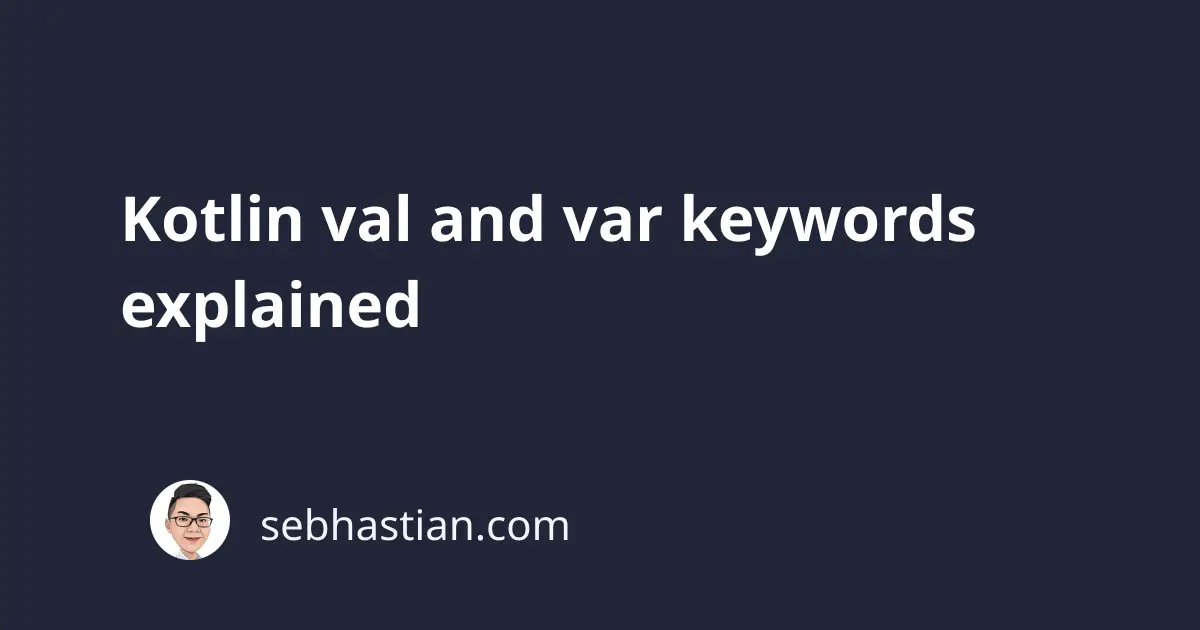
Both val
and var
keywords in Kotlin are used to declare variables.
The only difference between the keywords is that the value of var
keyword can be re-assigned while val
can’t.
To illustrate the difference, consider the following example:
var myNum = 15
myNum = 20
In the above example, the variable myNum
has the initial value of 15
which is then changed to 20
.
When you use the val
keyword to declare the variable, the re-assignment will trigger an error:
val myNum = 15
myNum = 20 // Error: Val cannot be reassigned
But although you can’t change the value of val
variables, the properties of the variable can still be changed if the variable type is an object.
For example, suppose you have a Member
class with a name
property as follows:
class Member(var name: String)
val user = Member("Nathan")
user.name = "Jack"
Even though the user
object is declared using the val
keyword, the name
property of the user
object can still be changed.
But this is because the name
property is defined using the var
keyword. If you use the val
keyword for the property, then changing the property will trigger the same error:
class Member(val name: String)
val user = Member("Nathan")
user.name = "Jack" // Error: Val cannot be reassigned
To conclude, any variable declared using val
is immutable (cannot be reassigned) while var
variables are mutable (can be reassigned)
When your variable is an object, the keyword used to declare any property will determine if the property can be reassigned.