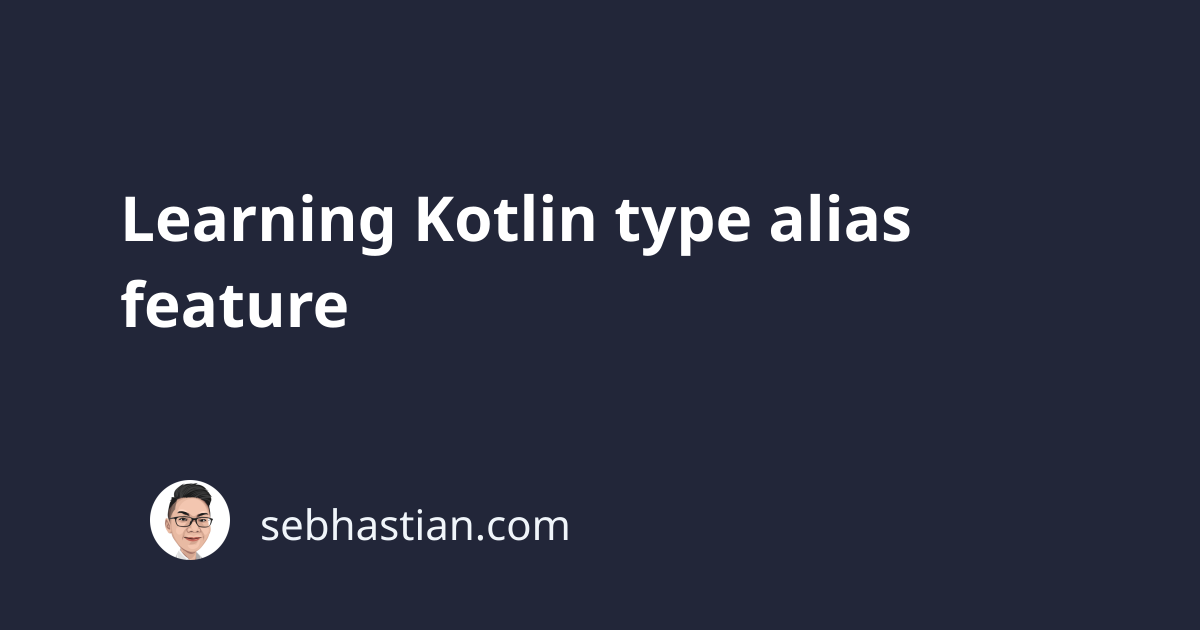
The Kotlin type alias feature allows you to define an alternative name for your types, no matter if the type is built-in or custom.
To create a type alias in Kotlin, you need to use the typealias
keyword followed by the alias name and the alias type.
typealias [alias name] = [alias type]
For example, you can alias the String
type as Str
as shown below:
typealias Str = String // Str type equals String
val myString: Str = "Nathan"
Kotlin’s type alias feature allows you to create alternative, shorter names for long type names.
The typealias
keyword can be used for Kotlin classes, functions, and generic types.
Below is an example of a Kotlin class
alias:
class UniqueIdentifier(var value: String)
typealias Uid = UniqueIdentifier
val userId = Uid("0001")
Next, you can also create an alias of function types:
typealias Length = (String) -> Int
val checkLength: Length = { it.length }
println(checkLength("Nathan")) // 6
The checkLength
variable is essentially a function that accepts a String
parameter and returns an Int
value.
When developing Android applications, you can create an alias of Android R
properties:
typealias Id = R.id
typealias Layout = R.layout
typealias Drawable = R.drawable
//...
setContentView(Layout.activity_main)
val button = findViewById<Button>(Id.button_id)
Type alias can also be used in place of import
as follows:
typealias Text = android.widget.TextView
// equals to..
import android.widget.TextView as Text
But it’s not recommended to use typealias
in place of import
unless you have a strong reason.
Kotlin type aliases don’t introduce new types because Kotlin replaces any alias with the real type during compile time.
This means the generated Java code executed during runtime uses the original type.
Error when using typealias
When running the examples below, you might see Kotlin throw an error saying:
Nested and local type aliases are not supported
This is an issue with Kotlin that has been resolved in the latest version. You need to make sure that the Kotlin library you use is >=1.6.10
.
Also, the error will always appear when you run Kotlin code as a Scratch file in IntelliJ IDEA or Android Studio.
A typealias
must be defined outside of a function as follows:
typealias Str = String
fun main() {
val name: Str = "Nathan"
println(name)
}
When the Nested and local type aliases are not supported
error message is thrown, most likely there are aliases defined inside a function as shown below:
fun main() {
typealias Str = String
val name: Str = "Nathan"
println(name)
}
This is why typealias
always cause an error when run from Scratch files. All the code you write there is executed as if it would be in the body of the main
function.
When aliasing a class, you can declare the typealias
and the class
as shown below:
class UniqueIdentifier(var value: String)
typealias uid = UniqueIdentifier
fun main() {
val userId = uid("0001")
println(userId.value)
}
The typealias
must be positioned outside of the main
function.
Great work on learning about Kotlin type alias. 👍