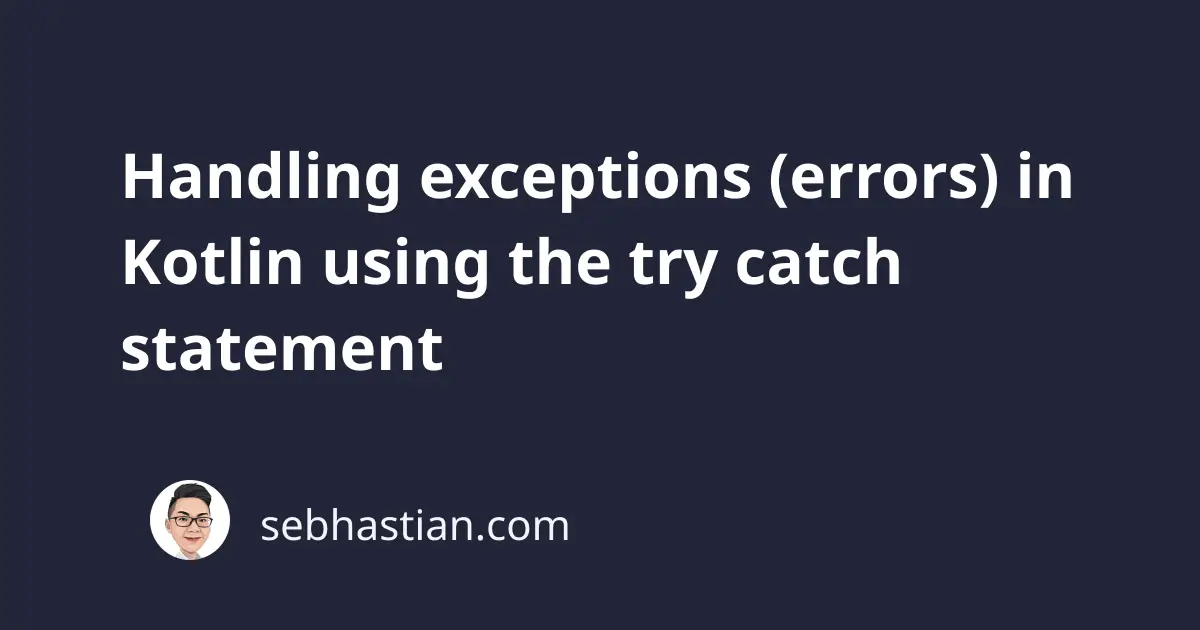
As you write your Kotlin program, there will be times when your code unexpectedly hit an error and can’t continue its execution.
For example, Kotlin has the .toInt()
function that parses a string
as an Int
number and return the result to you.
The function will throw an error when you call it on a string
variable that has no valid whole number representation.
The following code will throw an error:
var myVariable = "Hello"
myVariable.toInt() // this causes error!
println("Finished running code")
Because the string "Hello"
has no valid number representation, Kotlin will throw an error (also known as an Exception
) as shown below:
Exception in thread "main" java.lang.NumberFormatException:
For input string: "Hello"
at java.base/java.lang.NumberFormatException.forInputString
...
When the above Exception
happens, Kotlin will crash and stop executing any code below the line that causes the Exception
.
In the case of our example above, the println()
function won’t be executed.
The try
and catch
statements are a group of statements that allow you to control what happens when Kotlin encounter an Exception
just like the one above.
It can be used to let your code handle exceptions gracefully and continue the code execution.
To use the statements, you can create blocks of code with the following pattern:
try {
// the code to check for error
} catch (e: Exception) {
// what to do when the exception happens
}
The try
and catch
statements each have a code block marked by the curly brackets {}
.
The code you write in their blocks will be executed as follows:
- The code you write inside the
try
block will be executed first - If there is no error until the end of the
try
code, thecatch
block will be skipped - When the
try
code hits an error, Kotlin will skip the line after the error and move to thecatch
block - Kotlin will pass the error details into the
e
variable. You’re free to name it anything
Now let’s try to run the code below to see try..catch
in action:
var myVariable = "Hello World"
try {
myVariable.toInt()
} catch (e: Exception) {
println("Hey you have an error! $e")
}
println("Finished running code")
The output of the code above will be as follows:
Hey you have an error!
java.lang.NumberFormatException: For input string: "Hello World"
...
Finished running code
The call to the .toInt()
function above still causes an exception, but because there’s a try..catch
block, the exception can be handled by Kotlin.
After printing the exception message, Kotlin continues to execute the code below the catch
statement without crashing.
Catch function exception parameter type
Another thing you need to know is that you need to add type annotation to the e
parameter of the catch()
function.
The most common type you can use for the e
parameter is the Exception
class, which is the superclass of all exception subclasses.
One such subclass is the NumberFormatException
class as you’ve seen in the code example above.
Because the toInt()
function throws the NumberFormatException
error, you can replace the Exception
type above as shown below:
var myVariable = "Hello World"
try {
myVariable.toInt()
} catch (e: NumberFormatException) {
println("Hey you have an error! $e")
}
It is safer to use Exception
superclass however, since specifying the exact exception subclass will cause your try..catch
block to ignore exception of other types.
For example, suppose you have a piece of code that throws the ArithmeticException
as shown below:
try {
val result = 25 / 0 // throws ArithmeticException error
result
} catch (e: NumberFormatException) {
println("Hey you have an error! $e")
}
The ArithmeticException
error from the above example is ignored since the catch
function only accepts an exception of NumberFormatException
class.
This is why you may default to annotate the e
type as Exception
unless you know the specific exception you want to catch.
You’ve learned how to handle exceptions using the try catch
statements in your Kotlin program. Great job!