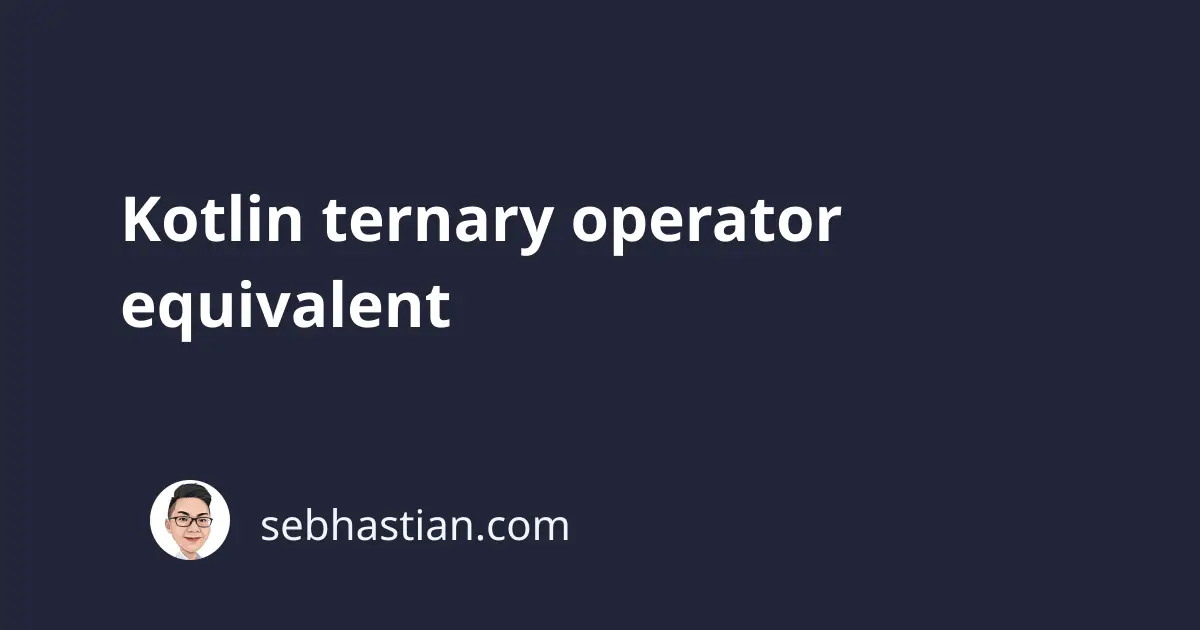
You may have seen the ternary operator in other languages like Java or JavaScript.
A ternary operator allows you to assign a conditional expression to a variable.
For example, the following JavaScript code assigns the value of variable a
to myVar
only if a
contains a truthy value:
let a = 3;
let myVar = a ? a : "else";
console.log(myVar); // 3
If variable a
contains a falsy value (like null
, false
, or undefined
) then the string "else"
will be assigned to myVar
.
Kotlin doesn’t have a ternary operator, replacing the ternary operator with the if..else
statement instead.
Here’s the Kotlin equivalent of the JavaScript code above:
var a: Int? = 3
var myVar = if(a != null) a else "else"
print(myVar) // 3
When you replace the value of a
with null
, the else expression will kick in:
var a: Int? = null
var myVar = if(a != null) a else "else"
print(myVar) // "else"
You don’t need a ternary operator in Kotlin because many control statements like if
, else
, and when
can be used as expressions in Kotlin.
Kotlin allows you to assign the expression result to a variable or return it from a function.
Replacing ternary operator with elvis operator
Alternatively, Kotlin also has the elvis operator that you can use to shorten the if..else
syntax.
The elvis operator is a question mark and colon symbol (?:
) that can be used to create a conditional expression.
Take a look at the following example of replacing the myVar
assignment with elvis operator:
var myVar = if(a != null) a else "else"
// or
var myVar = a ?: "else"
The elvis operator can be used to safely handle assigning the nullable variable a
value to myVar
.
When the value of a
is null
, then Kotlin will take the expression on the right side of the elvis operator.
To summarize, Kotlin doesn’t have a ternary operator like in many other traditional programming languages.
You can use the normal if..else
statements as expressions and assign the result to a variable, or you can use the elvis operator.