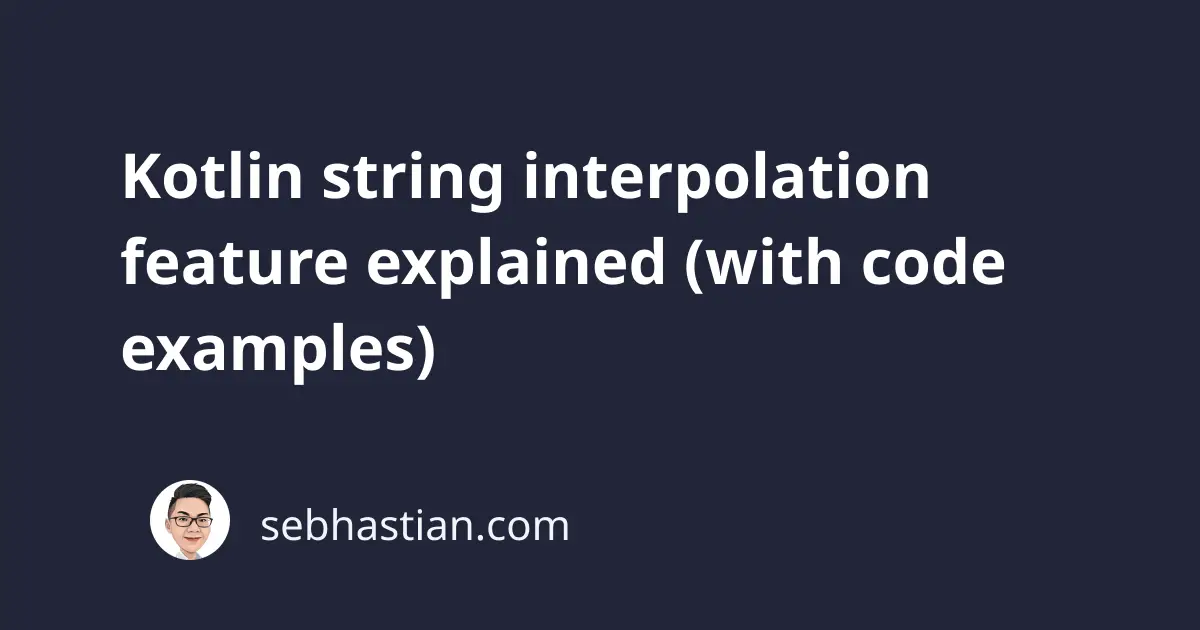
The Kotlin string interpolation feature allows you to embed expressions into a string so that you don’t have to concatenate an expression into a string using the +
symbol.
For example, when you want to print a variable value with some extra text, you may write the code as shown below:
var myName = "Samwise"
print("Hello, " + myName + "!")
While the code works just fine, you can write a cleaner code using string interpolation as follows:
var myName = "Samwise"
print("Hello, $myName!")
The string interpolation feature allows you to evaluate an expression simply by adding the dollar symbol $
before the variable name.
You also don’t need to exit the string and concatenate the variable using the +
symbol with string interpolation.
But keep in mind that when you have an expression that’s more than a single variable name, you need to add curly brackets {}
after the $
symbol.
For example, suppose you want to print out the length of the myName
variable above. Here’s how you do it:
var myName = "Samwise"
print("myName length is ${myName.length}")
// myName length is 7
The same also applies when you want to do other evaluations, such as adding two numbers together:
var x = 8
var y = 10
print("x + y equals ${x + y}")
// x + y equals 18
Finally, you can also call a function from a string thanks to the string interpolation feature.
The following example calls the sum()
function directly inside the print()
string argument:
var x = 8
var y = 10
fun sum(a: Int, b: Int): Int {
return a + b
}
print("x + y equals ${sum(x, y)}")
// x + y equals 18
Now you’ve learned how the string interpolation feature works in Kotlin. Well done! 👍