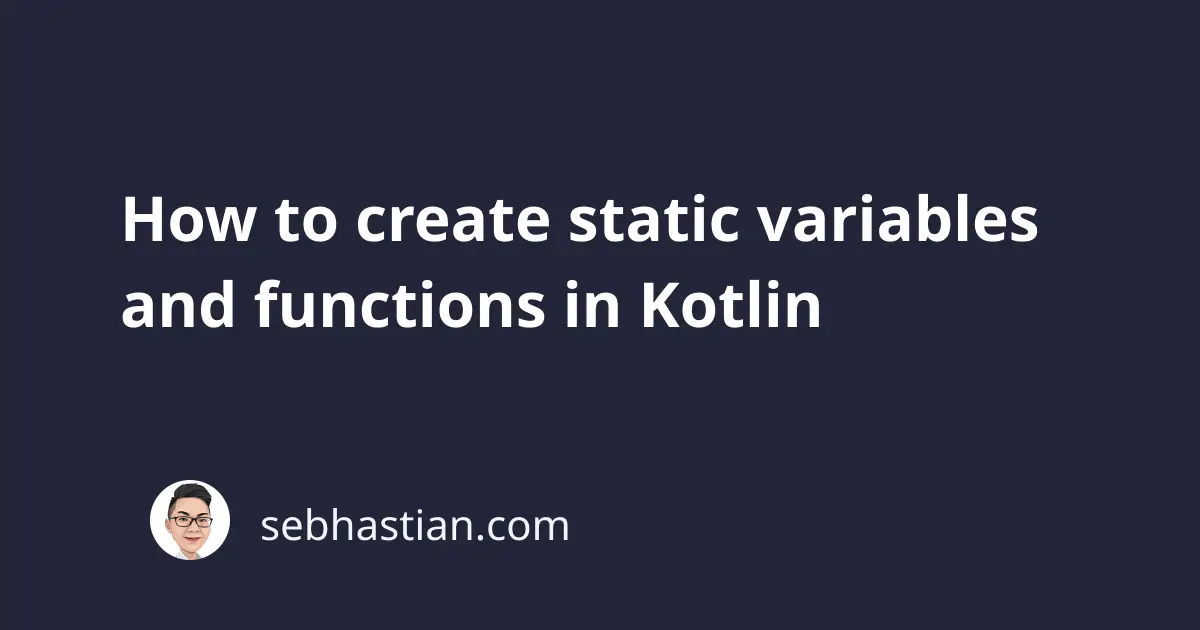
In Kotlin, you have the const
keyword that’s equal to the static
keyword in Java.
The const
keyword is used to create a variable that’s known to Kotlin before the compilation, so you don’t need to create an instance of a class to use it.
The val
keyword is used to create an immutable variable, just like the final
keyword in java.
Combined together, the const val
keyword allows you to create static variables in Kotlin object
as follows:
object MyObject {
const val OBJ_DESCRIPTION = "An object with a static variable"
}
print(MyObject.OBJ_DESCRIPTION)
// An object with a static variable
The const val OBJ_DESCRIPTION
above would be equal to final static String OBJ_DESCRIPTION
in Java.
A static method can also be created in a Kotlin object
just like creating a regular method:
object MyObject {
const val description = "An object with a static variable"
fun staticFun() = print("A static function")
}
print(MyObject.description)
MyObject.staticFun()
When you need to add static members to a class
, however, you need to create a companion object
inside the class
and put the static members inside the object.
Here’s an example of a Kotlin class
with static members:
class MyClass {
companion object {
const val staticVar = "A static variable"
fun staticFun() = print("This is a static function")
}
}
print(MyClass.staticVar)
// A static variable
MyClass.staticFun()
And that’s how you can create static variables and functions inside a Kotlin object
or class
types 😉