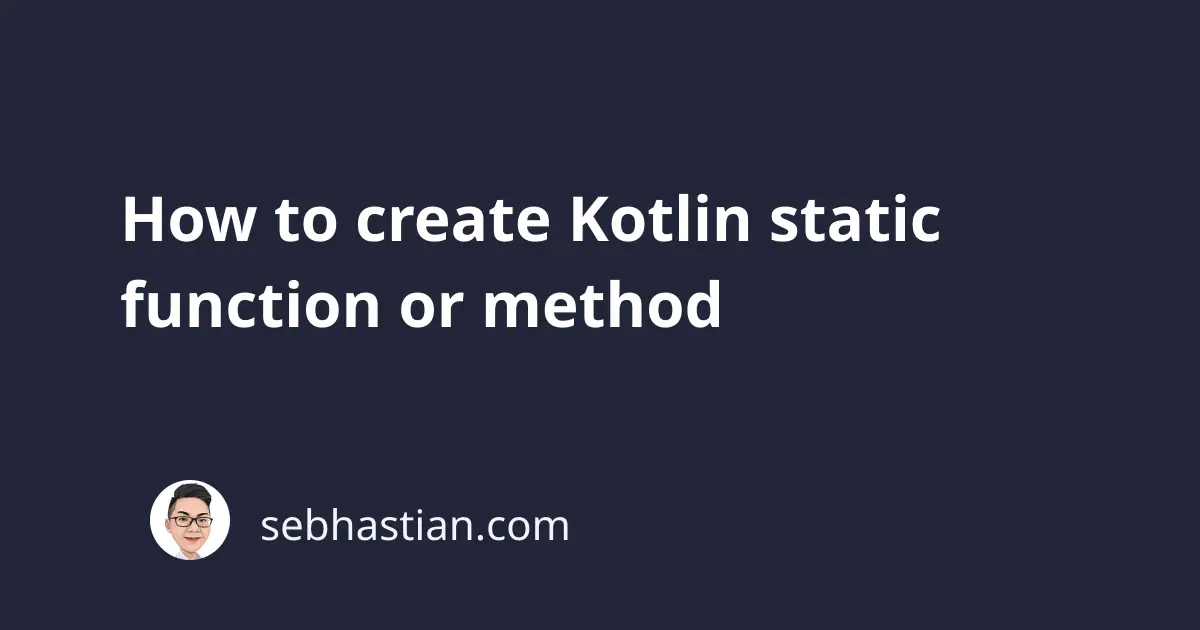
Unlike Java programming language, Kotlin doesn’t have a static
modifier that you can use to define static functions (methods) of a class
.
Instead of using the static
modifier, Kotlin allows you to create a companion object
inside a class to declare static functions.
The following Java code:
class Main {
public static String hello() {
return "Hello World";
}
}
System.out.println(Main.hello()); // Hello World
is equivalent to the following Kotlin code:
class Main {
companion object {
fun hello(): String {
return "Hello World"
}
}
}
println(Main.hello()) // Hello World
In both languages, the function hello()
can be called with Main.hello()
without creating a new instance of the class
.
Under the hood, the Kotlin companion object
creates a Companion
class inside the Main
class, so if you need to call the Kotlin static code from Java, you need to call it from the Companion
class like this:
Main.Companion.hello();
If you don’t want the extra Companion
call, you can add the @JvmStatic
annotation before writing the static function like this:
class Main {
companion object {
@JvmStatic
fun hello(): String {
return "Hello World"
}
}
}
The annotation will cause the Kotlin code to generate an additional static
method when compiled to Java bytecode.
In the code below, the highlighted lines are only generated when you add the @JvmStatic
annotation:
public static final class Main {
@NotNull
public static final Scratch.Main.Companion Companion =
new Scratch.Main.Companion((DefaultConstructorMarker) null);
@JvmStatic
@NotNull
public static final String hello() {
return Companion.hello();
}
public static final class Companion {
@JvmStatic
@NotNull
public final String hello() {
return "Hello World";
}
private Companion() {
}
// $FF: synthetic method
public Companion(DefaultConstructorMarker $constructor_marker) {
this();
}
}
}
With the @JvmStatic
annotation, you can call the method from Java using the same syntax:
Main.hello();
But you only need this when you call the compiled Kotlin code from Java.
Kotlin static function in a Kotlin object
Aside from creating a companion object
in a Kotlin class
, you can also create a Kotlin object
to keep your static functions.
A Kotlin object
is a static instance that can’t be instantiated like a class
, so it’s perfect for storing your static variables and functions.
Here’s the same static hello()
function in a Kotlin object:
object Main {
fun hello(): String {
return "Hello World"
}
}
println(Main.hello()) // Hello World
You are free to use a class
or an object
to define your static functions in Kotlin.