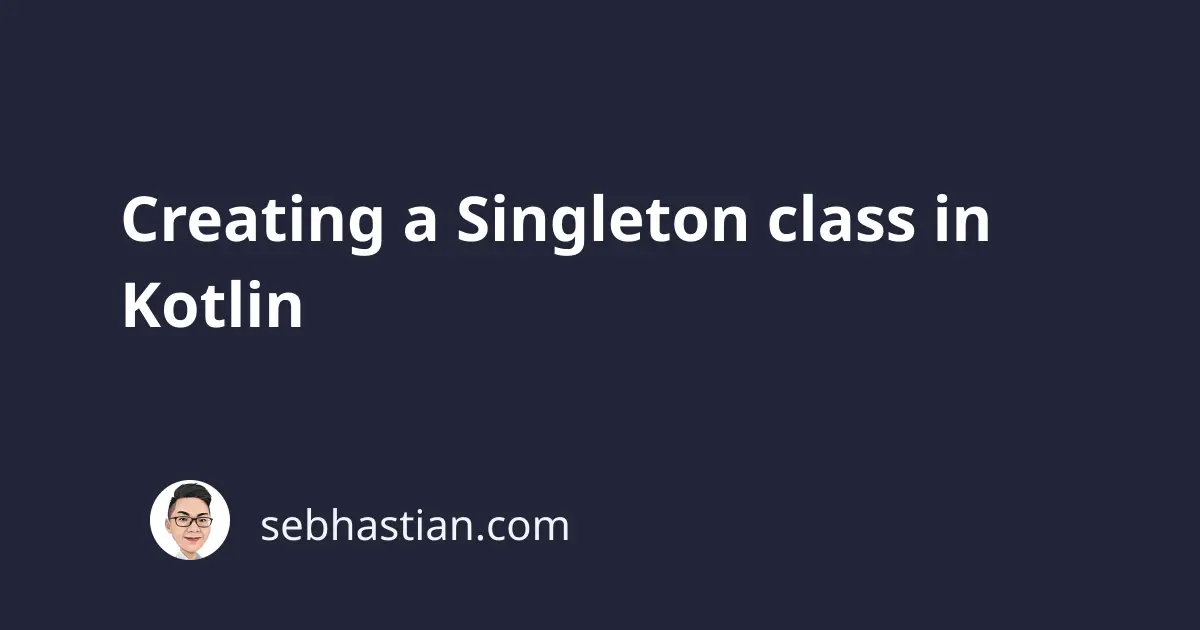
A Singleton class is a class that has only one instance as long as the application lives in the memory.
It also needs to be accessible publicly so you can share the class members between different parts of your application.
In Kotlin, a singleton class can be created by using the object
keyword.
An object
in Kotlin gets initialized during the first it was required by your source code.
For example, suppose you create an object named Util
as shown below:
object Util {
val name = "Nathan"
fun hello() {
println("Hello I'm $name")
}
}
The Util
object will be initialized the first time it was called during the runtime, while subsequent calls will access the same instance.
Util.hello() // object gets initialized
println(Util.name) // use the created instance
Unlike in Java, Kotlin provides built-in support for the Singleton pattern so it’s very easy to create one.
And that’s how you create a Singleton class in Kotlin. 😉