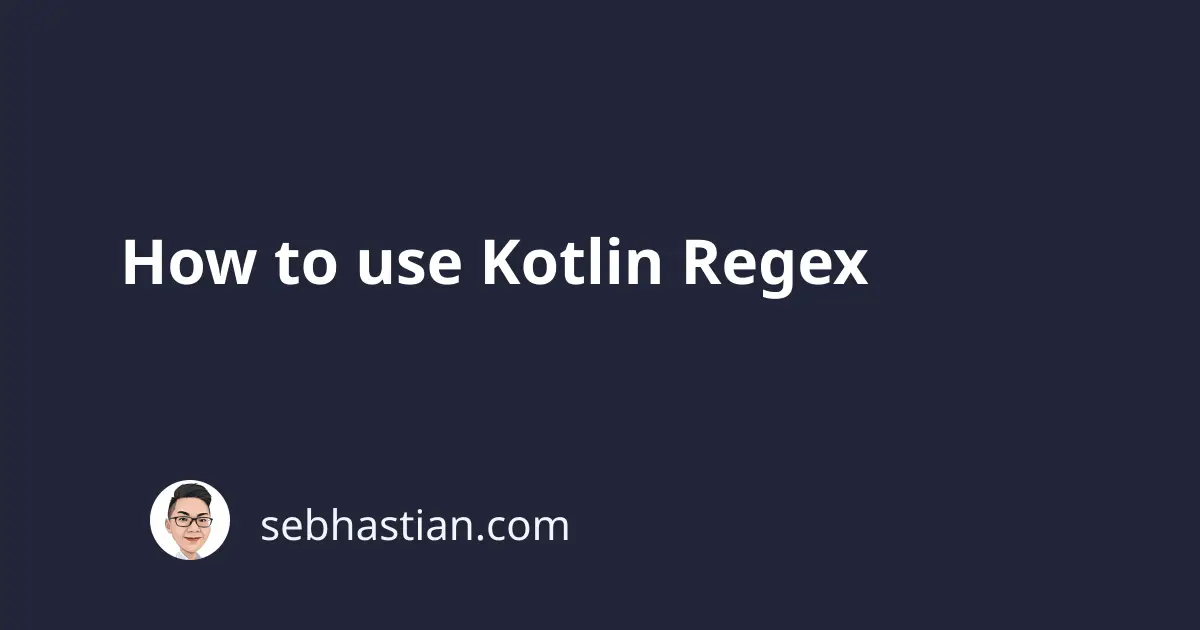
The Kotlin Regex
class can be used to create a regular expression pattern that helps you find or replace matching strings.
The syntax of the Regex
class is as follows:
Regex(pattern: String, option: RegexOption)
// or pass multiple options as a Set
Regex(pattern: String, option: Set<RegexOption>)
Let’s see an example of Regex
in action.
To create a regular expression pattern, create a new instance of the Regex
class as follows:
val regex = Regex("Island")
val text = "The Great Britain Island is located east of the Island of Ireland"
val matches = regex.findAll(text)
matches.forEach {
println(it.value)
}
// result:
// Island
// Island
The instance of the Regex
class can then be used to search or replace any string text by calling a specific method from the instance.
In the example above, the findAll()
method is called to search for the Island
string from the text
variable value.
You can also pass a second argument to the Regex
call as follows:
The list of methods that you can call from the Regex
object can be found in the Kotlin documentation.
Some of the most useful methods are as follows:
find()
findAll()
matchAt()
matchEntire()
replace()
replaceFirst()
Kotlin also has the RegexOption
enum class that’s used to set the options for the running method.
The list of available options are as follows:
IGNORE_CASE
MULTILINE
LITERAL
UNIX_LINES
COMMENTS
DOT_MATCHES_ALL
CANON_EQ
For example, the following code replaces the word Island
with Isle
while ignoring the cases:
val regex = Regex("island", RegexOption.IGNORE_CASE)
val text = "The Great Britain Island is located east of the Island of Ireland"
val newText = regex.replace(text, "isle")
println(newText)
// output:
// The Great Britain isle is located east of the isle of Ireland
You can create any kind of valid regular expression to use with the Kotlin Regex
class.