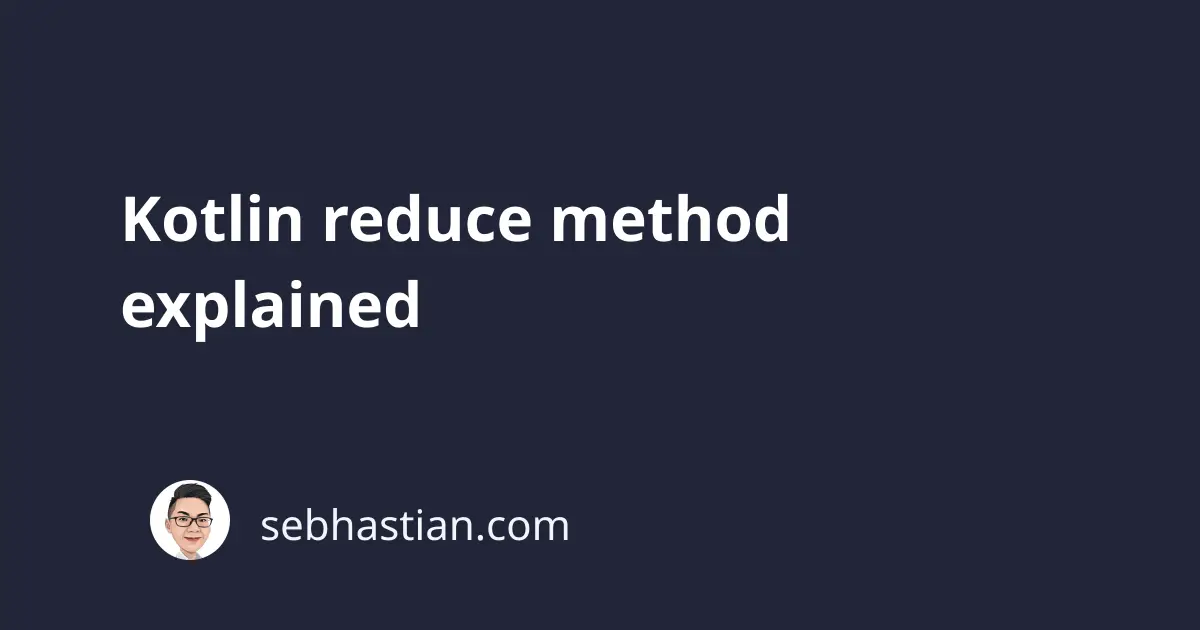
The Kotlin reduce
method is used to apply a reducer function to a set of collections that you have in your code.
A reducer function is a function that performs a custom operation for each element in a collection with the goal to return a value.
The value returned by the reducer function then accumulates until a single final value is returned from the reduce
method.
It’s a bit confusing to learn about the reducer in theory, so let’s see an example of a reducer in action.
Imagine that you have a List
of Int
values, and you want to sum all the values in the list.
You can use the reduce
method as follows:
val numbers = listOf(1, 2, 3, 4)
val aSum = numbers.reduce { accumulator, currentEl ->
println("accumulator: $accumulator")
println("currentEl: $currentEl")
accumulator + currentEl
}
println(aSum) // 10
The output of the println
statement in the reduce
method above looks as follows:
accumulator: 1
currentEl: 2
accumulator: 3
currentEl: 3
accumulator: 6
currentEl: 4
As you can see, the reduce
method iterates over a collection of elements where it was called and pass the values of your collection into the reducer function, which is the entire code inside the curly brackets {}
above.
The curly brackets are Kotlin lambda function expression. It’s simply a shorter way to pass a function without a name to the reduce
method.
Here’s an equivalent to the code above without lambda function expression:
val aSum = numbers.reduce(fun(accumulator: Int, currentEl: Int): Int {
println("accumulator: $accumulator")
println("currentEl: $currentEl")
return accumulator + currentEl
})
The lambda function expression is shorter and more concise than a regular anonymous function as shown above.
For the first iteration of the reducer function, the first index value in your list will be passed as the accumulator
and the second index value as the currentEl
(or current element)
Then, you are free to perform operations on these values, as long as you return a single value that will be passed as the accumulator
value of the next iteration.
The Kotlin reduce
method is available for all types of collections, so you can use it on Int
, String
, Char
, or Boolean
lists as you need.
val letters = listOf("ab", "cd")
val combinedLetters = letters.reduce { accumulator, currentEl ->
accumulator + currentEl
}
println(combinedLetters) // abcd
Now you’ve learned how the reduce
method works in Kotlin. Great work! 👍