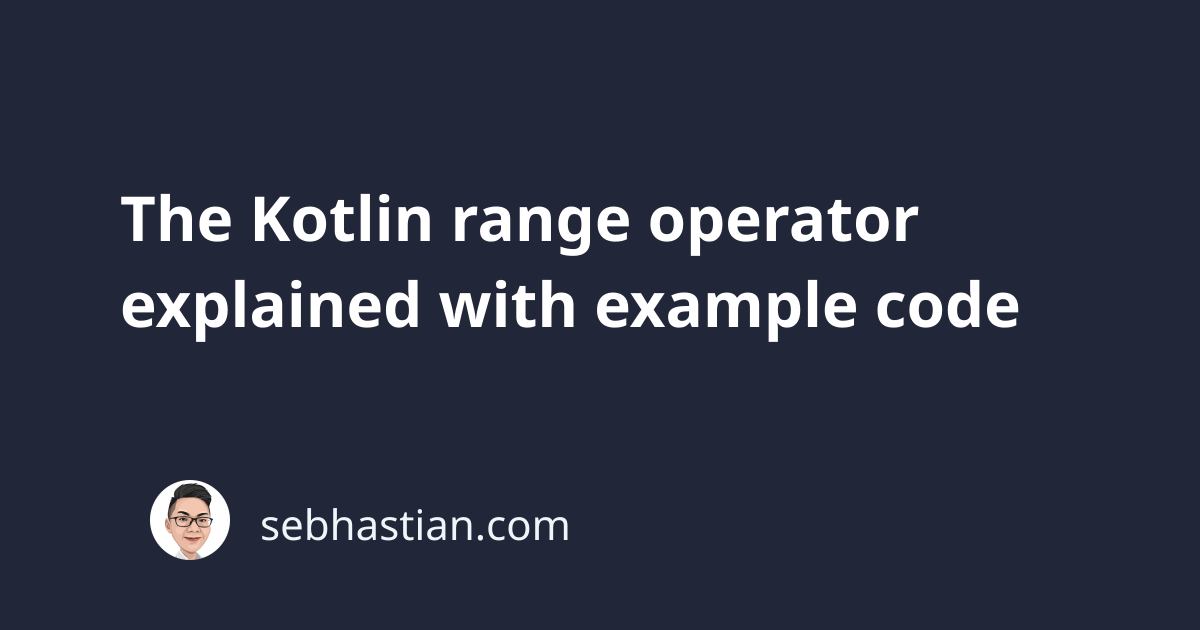
The Kotlin range operator (..
) is a unique operator that allows you to create an interval of numbers or characters.
This range of numbers or characters can then be used for specific purposes like:
- checking if a value is included inside that range
- iterating over the range and producing an output
- perform collection operations like
filter
andmap
There are three types of range that you can create in Kotlin: an IntRange
for integer numbers, a CharRange
for characters, and a LongRange
for Long numbers.
A range is commonly created using the two dots (..
) symbol. Let’s see some ranges in action next.
The following example shows how you can check if the number value 2
is inside an IntRange
of 1..4
:
if (2 in 1..4) {
print("2 is inside 1 to 4")
}
Because the number 2
is inside the range, the print()
statement above will be executed by Kotlin.
You can also initialize a variable as a range as shown below:
var numRange = 1..4
if (2 in numRange) {
print("2 is inside 1 to 4")
}
Next, you can iterate over a range using the for
statement like this:
var numRange = 1..4
for (i in numRange) println(i)
/* Output:
1
2
3
4
*/
An iteration over a range is inclusive for both the start point and the endpoint.
If you don’t want to include the endpoint, you can switch the ..
symbol with the until
keyword:
var numRange = 1 until 4 // range of 1 - 3
for (i in numRange) println(i)
/* Output:
1
2
3
*/
Finally, you can perform collection operations on a range like filter
and map
as shown below:
var numRange = 1..5
var numList = numRange.map {
it + 3
}
print(numList) // [4, 5, 6, 7, 8]
numList = numRange.filter {
it % 2 == 0
}
print(numList) // [2, 4]
The map
and filter
operations produce a List<Int>
type that you can store in a variable.
Kotlin CharRange example
Kotlin also allows you to create a range of CharRange
type which consists of characters as shown below:
var charRange = 'a'..'f'
if ('b' in charRange) {
print("b is inside a to f")
}
Keep in mind that the character range in Kotlin is case-sensitive. When you check for an uppercase letter in
a range of lowercase letters, it will return false
:
var charRange = 'a'..'f'
if ('B' !in charRange) {
print("Uppercase B is NOT inside a to f")
}
A range of characters must be consistent between a lowercase range or an uppercase range.
If you mix the case, you need to start with the uppercase letter since Kotlin follows the ASCII character ordering.
Kotlin won’t be able to return any value from the range if you messed up the range ordering.
Take a look at the following example:
var charRange = 'a'..'C'
for(i in charRange) println(i) // prints nothing
charRange = 'X'..'c'
/*
Output:
X
Y
Z
[
\
]
^
_
`
a
b
c
*/
You can perform the same operations on the CharRange
type just like in the IntRange
type.
Kotlin range progression manipulation
Finally, you can manipulate how the progression in a Kotlin range works.
The previous examples all show how a Kotlin range progresses from a lower value to a higher value.
You can, for example, reverse the progress by using the downTo
operator or the reversed()
function.
Here’s an example:
for (i in 1..5) print(i) // 12345
for (i in 5 downTo 1) print(i) // 54321
for (i in (1..5).reversed()) print(i) // 54321
You can also manipulate the progression to move more than a single step
for each iteration.
For example, you can move 2
steps at a time in the range progression as shown below:
for (i in 1..5 step 2) print(i) // 135
for (i in 6 downTo 1 step 2) print(i) // 642
The value of step
defaults to 1
, and it must always be greater than 0
or Kotlin will throw a runtime error.
And that’s how the range operator in Kotlin.
The Range
type value that the range operator produces can be used for creating a conditional if
statement, iterating over an interval of values, or performing collection operations such as filter
and map
.
Great job on learning the Kotlin range operator! 👍