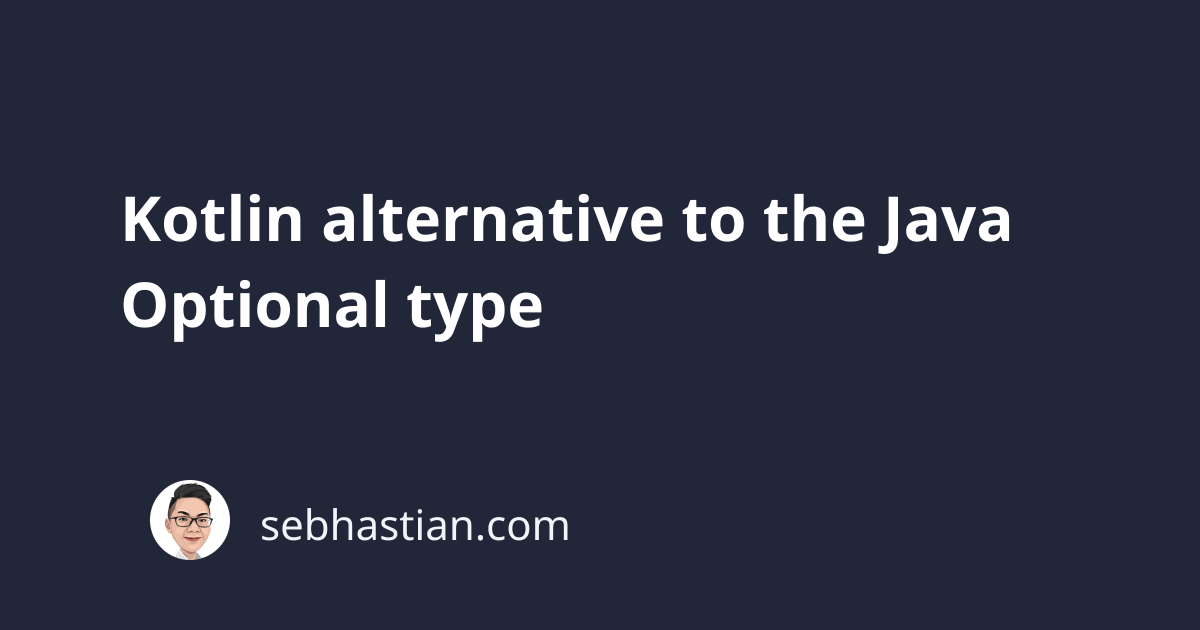
The Java Optional
type is a new class introduced in Java 8 that allows your variables to return empty values.
This type is commonly used when you perform an API call that can return null
values.
With the Optional
type, you can handle the null
or empty value gracefully in your program.
Consider the following Java program example:
public static void main(String[] args) {
Optional<String> myName = getName(true);
}
private static Optional<String> getName(Boolean isUser) {
if (isUser){
return Optional.of("Nathan");
}
return Optional.empty();
}
In the main()
method of the Java code above, the variable myName
value is assigned the result of the call to the getName()
method.
The getName()
method may return a String
wrapped in Optional.of()
to make it an Optional<String>
type.
The method may also return an Optional.empty
value created from Optional.empty()
method.
In Kotlin, there’s no Optional
type to allow null
or empty values in your variable.
Instead, Kotlin uses what’s known as null safety features to help you handle null
values in your source code.
Kotlin uses the question mark ?
symbol to define an optional variable. Consider how the type of the variable below is String?
with a question mark:
var myName: String? = "Nathan"
The ?
above means that the variable myName may have a valid String
value or a null
value.
The equivalent Java Optional
variable would look as follows:
Optional<String> myName = Optional.of("Nathan");
When a variable is null
or empty in Kotlin, you need to call the class
methods or properties using the safe call operator (?.
)
Consider the following Kotlin code:
var myName: String? = null
println(myName?.length)
By just adding a question mark ?
before the call to length
property, Kotlin helps you avoid the NullPointerException
error that commonly occurs in Java source code.
The Java equivalent to the Kotlin code above would be as follows:
Optional<String> myName = Optional.empty();
myName.ifPresent(name -> System.out.println(name));
The Optional.empty()
method call returns an empty instance of the Optional
class.
Java will then check on the value of myName
variable by using the ifPresent()
method.
When there’s a value in the variable, Java will execute the lambda action defined as the ifPresent()
method’s argument.
In the case above, it’s to print out the name
value.
You need to use the Optional.empty()
method to make the Optional
type empty. If you use null
, then the call to the ifPresent()
method above will cause a NullPointerException
error.
Conclusion
The Optional
type is a new addition to the Java programming language since version 8 that helps you handle empty values in your Java code.
In Kotlin, the Optional
type is simplified into Kotlin’s null safety features, which helps eliminate the danger of referencing null
values in your Kotlin code.
You can learn more about Kotlin null safety features here:
Kotlin uses of question marks explained
While Java requires the use of the Optional.empty()
method to handle empty values that are not null
, Kotlin allows you to work with literal null
values in your code to make things easier.